Creating multiline strings is a common requirement for developers handling text-based data. JavaScript provides several methods to accomplish this, making it easier for developers to work with textual content in an organized and readable manner.
You can create multiline strings in JavaScript by enclosing the string with backticks (“) to create a template literal.
You can also create these multi-line strings by using string concatenation or escaping each line with a backslash( \).
Understanding these techniques can greatly enhance a web developer’s ability to work with complex, text-heavy applications or scripts.
So, let’s look at how multiline strings work!
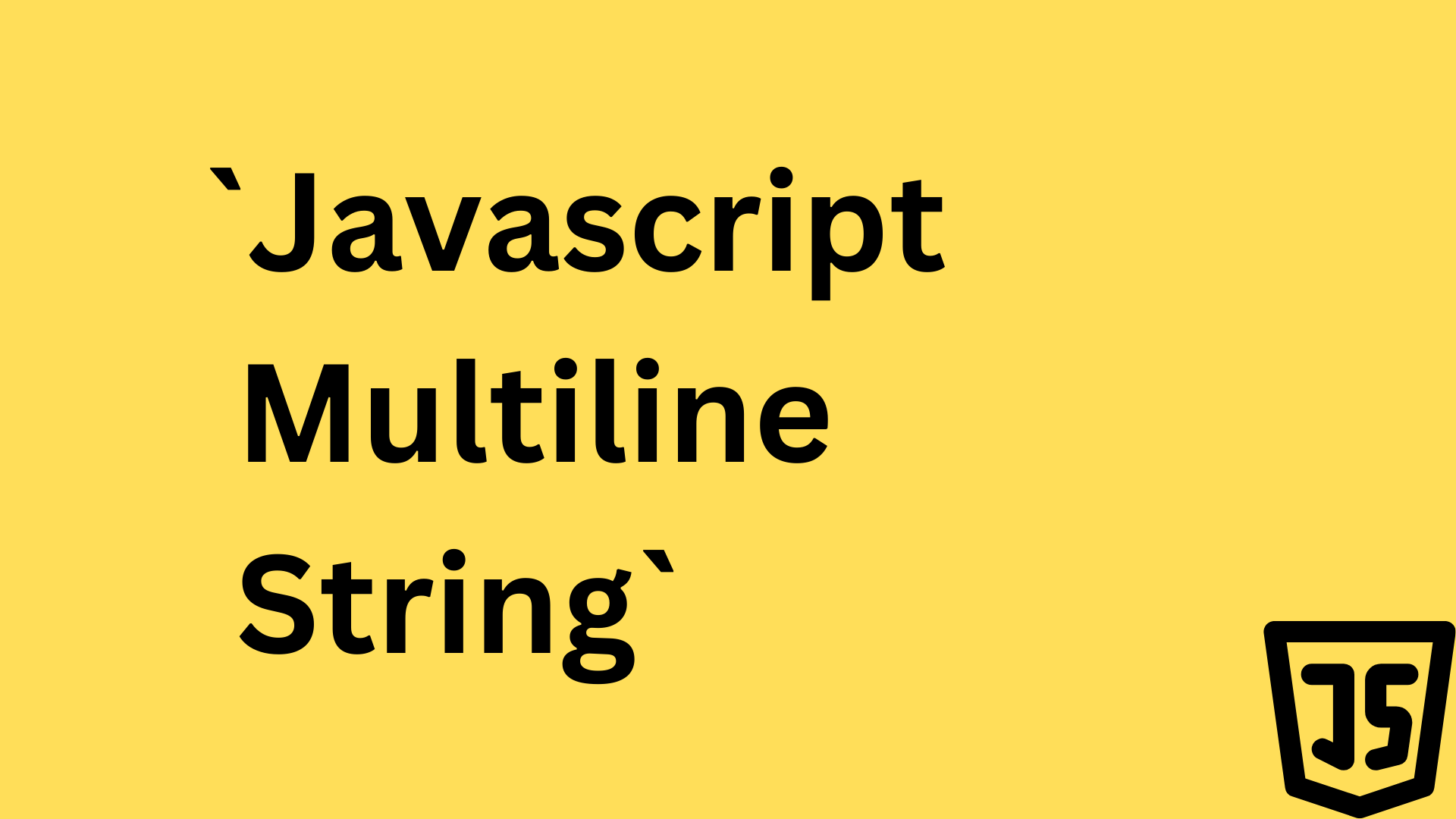
Understanding Multiline Strings
Multiline strings in JavaScript are a way to represent text spanning multiple lines within the code.
Traditionally, JavaScript deals with strings as single-line values, enclosed in single or double quotes.
However, when dealing with larger blocks of text, this approach makes the code less readable and more complicated to maintain.
Using multiline strings helps improve the maintainability of the code, especially for large blocks of text, templates, or queries.
The clear and legible format enhances the readability and ease of modification for developers working on JavaScript projects.
Some use cases of multiline strings in Javascript include:
- HTML templates: When creating HTML templates within JavaScript, multiline strings make it easier to visualize the structure and apply changes.
- SQL queries: In JavaScript applications that interact with databases, multiline strings can be used to construct SQL queries. This makes it easier to read and edit the queries within the code.
How to Implement Multiline Strings in Javascript
There are several methods for creating multiline strings in JavaScript. Each method serves the same purpose but each has its own benefits and limitations.
In this section, we’ll explore these techniques and their practical applications.
Using Template Literals
Introduced in ES6, template literals offer a more advanced and flexible method for creating multiline strings. By enclosing a string between backticks, we can create special strings called template strings.
These template strings can be used to perform complex operations like displaying multiline strings, string interpolation, etc.
Here’s an example:
const name = `Hello
My name is John
I love Javascript`
console.log(name)
Output:
Another feature we can use within template strings is string interpolation.
String interpolation is the process of embedding expressions within string literals, using ${expression} syntax.
It reduces the need to concatenate strings and variables manually.
For example:
let name = "John";
let greeting = `Hello
My name is ${name}
I love Javascript`;
console.log(greeting)
Output:
Hello
My name is John
I love Javascript
As you can see, the name variable is embedded directly into the string.
Using Backslashes
Backslashes (\) are a very useful tool for creating multiline strings in your Javascript code.
However, there is one key difference between backslashes and the method listed above.
Backslashes are only useful for creating multi-line strings in your code. They do not format the output as a multiline string. When you print the string, everything still gets displayed on the same line.
For example, let’s enclose a multi-line text in double quotes without a backslash at each line break.
We can see that errors are raised in the IDE. Now, let’s add the backslashes to the end of each line in the text.
Output:
First line Second line Third line
Now, the errors are gone and the program prints out normally. We can also see that all the lines in the string are printed together.
Using the Newline Character
In JavaScript, you can print strings in a multi-line format using the newline(\n) character at the end of each line. This method is more cumbersome than using template literals, but it is still a valid way of creating multiline strings using single and double quotes.
Remember, template literals aren’t supported in all browsers, so you might need to use a different method while creating programs. To use this method, simply add the newline character at every point in the string where you want a line break.
Let’s take a look at it in action:
let text = "This is a multiline \nstring created using \nthe newline character.";
console.log(text)
Output:
This is a multiline
string created using
the newline character.
This technique allows you to break up the text over several lines for readability while keeping the string intact. However, it lacks some features like string interpolation.
Using String Concatenation
You can use the string concatenation operator(+) to create multiline strings. This is very helpful when you want to break up a long string over several lines.
Here’s an example:
var my_str = "This is the first line " +
"This is the second line " +
"This is the third line"
console.log(my_str)
Output:
This is the first line This is the second line This is the third line
If you want everything printed on different lines, you can add the newline character at the end of each phrase.
Here’s an example:
var my_str= "This is the first line\n" +
"This is the second line\n" +
"This is the third line\n"
console.log(my_str)
Output:
This is the first line
This is the second line
This is the third line
In summary, for a more modern and flexible approach with additional features, template literals are recommended for creating multiline strings.
However, if compatibility is a concern and you don’t need the extra functionality, you can use the additional methods above to create strings that span multiple lines.
Practical Examples of Multiline Strings in Javascript
There are many applications for multi-line strings in Javascript.
Two of the most common applications include HTML templates and SQL queries.
Let’s look at some practical examples of both.
HTML Templates
In JavaScript, it is common to work with HTML tags within strings, especially when dynamically generating or updating HTML content.
The following example demonstrates how to embed an HTML tag (such as <h1>) within a JavaScript string using template literals.
<h1>HTML Template Example</h1>
<div id = "newline"></div>
<button onclick=display_txt()>Click me!</button>
<script>
let my_var = `<p>TYou clicked the button!</p>
<p>Here's the Hidden Text</p>
<p>Goodbye!</P>`
function display_txt(){
document.querySelector('#newline').innerHTML = my_var;
}
</script>
Output:
In this example, the javascript code displays some text when we click the button. The HTML code for the text is stored inside a javascript variable using template literals.
You can also use string concatenation, depending on your specific requirements.
SQL Queries
We can also store SQL queries inside variables using multi-line strings. These variables are often long and they span multiple lines. So, storing them with multiline strings makes it easier to display this information in a legible format.
Here’s an example:
As we can see, the second command is easier to read because we used the multi-line string format.
Compatibility and Support
All browsers support the newline character and string concatenation. However, since template literals are a relatively new feature, some browsers might not support them.
Here is a list of some of the browsers and engines that support this feature:
Browser Support
- Chrome: Template literals, which support multiline strings, are fully supported in Google Chrome starting from version 41.
- Firefox: Mozilla Firefox has provided full support for template literals since version 34.
- Safari: In Apple’s Safari browser, template literals are supported beginning with version 9.
- Edge: Microsoft Edge started supporting template literals from its inception, starting with version 12.
- IE: Internet Explorer does not support template literals or multiline strings, and its development has been discontinued. Users are advised to switch to Edge or another modern browser.
- Opera: Opera offers full support for template literals since version 28.
Javascript Engine Compatibility
- V8: Google’s V8 JavaScript engine, which powers Chrome and Node.js, began supporting multiline strings with template literals starting in version 4.2.
- SpiderMonkey: Mozilla’s SpiderMonkey engine, used by Firefox, has provided support for template literals since version 34.
- JavaScriptCore: Safari’s JavaScriptCore engine (also known as Nitro) implemented support for template literals in version 9.
- Chakra: Microsoft’s Chakra engine, which powered EdgeHTML-based versions of Microsoft Edge, introduced support for template literals in Edge version 12.
- Carakan: Opera’s Carakan engine fully supports template literals as of version 28.
Final Thoughts
JavaScript multiline strings can be a valuable tool to enhance the readability and organization of your code. By utilizing multiline strings, you can significantly improve your code’s readability and maintainability, ultimately resulting in a better developer experience!
To conclude, it is essential to understand the different methods of creating multiline strings in JavaScript. Once you do, you can easily choose the best solution that suits your particular coding needs!
If you would like to learn about creating visuals in Power BI with a single line of code using R check out this video:
Frequently Asked Questions
How can I create multi-line strings from a Javascript Array?
You can create multi-line strings from a Javascript array using the Javascript join() function. If you use the newline character as the delimiter, each array element will be on a separate line.
Let’s demonstrate this:
const arr = ["Line 1", "Line 2", "Line 3"]
console.log(arr.join("\n"))
Output:
Line 1
Line 2
Line 3
How to escape a newline in a template string?
You can escape a new line in a template string by using a backslash. If you add a backslash at the end of the line, Javascript will continue printing out the string on the same line.
For example:
const str = `Line 1 and \
Line 2 will print on the same line
Line 3 will print on a different line`
console.log(str)
Output:
How to escape backticks in multi-line strings?
To escape backticks within multi-line strings using template literals, you can use the backslash (\) character before the backtick to make it an escape character:
let stringWithBacktick = `This string contains a backtick: \` And now the string continues.`;
How do you create a multiline string in TypeScript?
In TypeScript, which is a superset of JavaScript, you can create multiline strings using the same syntax as JavaScript – by using template literals:
let multilineString = `This is a multiline
string in TypeScript, created
using template literals.`;