Creating a multiline string in TypeScript can be important for you when working with complex text formatting and display. TypeScript is a superset of JavaScript that enables an easier way to manage multiline strings with its capabilities.
To create a multiline string in TypeScript, you can use the template literals, denoted by backticks. You can also use the line break or escape character \n for a newline and combine strings from an array using the array.join() method.
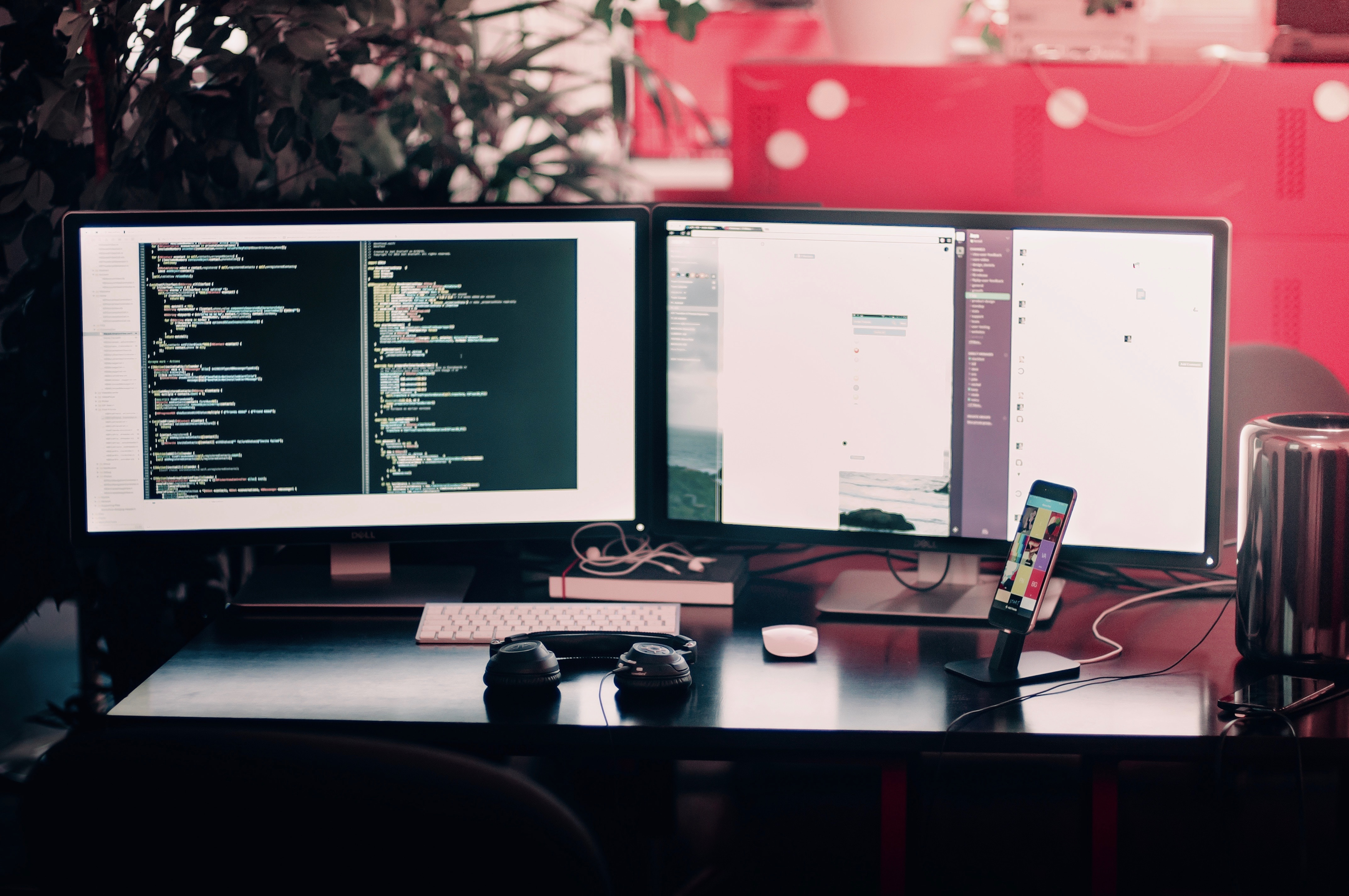
By understanding these different approaches and their respective use cases, you can efficiently create multiline strings in TypeScript. This will make your applications more robust and easier to maintain. In this article, we will discuss how to use TypeScript’s features to create and work with multiline strings effectively.
Let’s get into it!
What is a Multiline String in TypeScript?
As the name suggests, a multiline string span multiple lines.
The following example is a multiline string in TypeSript:
`
This is a multiline string.
In TypeScript, we use backticks.
It makes the code more readable.
`
How to Create a Multiline String With Backticks and Template Literals
In TypeScript, you can create multiline strings using template string literals. These are enclosed in backticks (“) instead of single or double quotes.
Template strings are a feature introduced in ECMAScript 6 (ES6) and are also available in JavaScript. They make it simple to create multiline strings without needing to use escape characters or the string concatenation operator.
The following is an example of creating a multiline string using backticks:
let multilineString: string = `
This is a multiline string.
In TypeScript, we use backticks.
It makes the code more readable.
`;
console.log(multilineString)
This code will create strings with text between backticks that spans multiple lines, and the new lines are preserved in the resulting string.
The output of the above code will be:
What is String Interpolation With Expressions in TypeScript?
Template literals also allow you to embed expressions inside the string. You can use the ${expression} syntax to insert the value of an expression within the string. This makes it easy to combine dynamic values with static text.
The following is an example of string interpolation in TypeScript:
let name: string = "Alice";
let age: number = 30;
let userInfo: string = `Name: ${name}
Age: ${age}
City: New York`;
console.log(userInfo);
This TypeScript code declares two variables, name and age, and assigns them the values “Alice” and 30 respectively. It then constructs a multi-line string userInfo using these variables and a template literal, and finally prints this string to the console.
String interpolation helps eliminate the need for concatenation using the + operator and makes the code more readable.
How to Manipulate Multiline Strings Using the Split and Join Methods
Split and join are two powerful prototype methods available to you when working with strings.
The split() method divides a string into an array of substrates. The join() method links elements of an array into a string.
- Split(): This method takes a separator as a parameter. It splits the string at each instance of the separator and returns an array.
let text = `Line 1 Line 2 Line 3`; let lines = text.split("\n");
- Join(): This method takes a separator as a parameter too. It reverses the split operation by joining the array elements into a single string with the separator.
let combinedLines = lines.join("\n");
You can easily split, manipulate, and rejoin strings using these methods.
Common Issues and Solutions With TypeScript
When working with multiline strings in TypeScript, you can sometimes run into issues with the syntax.
For this section, we’ve listed some common issues and their solutions.
1. Dealing With TypeScript Errors
One issue while working with multiline strings in TypeScript is accidentally introducing errors.
For instance, using single quotes instead of backticks can lead to errors as it doesn’t allow multiline strings. To prevent such issues, make sure to use backticks (`) when creating multiline strings.
For instance:
let myString = `
firstname: John,
lastname: Doe
`;
Another common issue is the improper use of string interpolation. To avoid errors, ensure you’re using the ${expression} syntax inside the multiline string.
For example:
let firstName = 'John';
let lastName = 'Doe';
let myString = `
firstname: ${firstName},
lastname: ${lastName}
`;
2. Handling Special Characters
When dealing with special characters in a multiline string, handling them correctly is important. You can enclose your string with backticks to avoid issues if you need to include characters such as single quotes or double quotes within the multiline string.
For example:
let myString = `
"firstName": 'John',
"lastName": 'Doe'
`;
However, use the appropriate escape sequence or newline character when dealing with other special characters that need to be escaped.
For instance, to include a backslash (\) or backtick (`), use a newline character such as:
- Backslash: \\
- Backtick: “`
The following is an example:
let myStringWithBackslash = `
folder1\\folder2\\file.txt
`;
let myStringWithBacktick = `
This is an example of a \`backtick\` inside a multiline string.
`;
In case your string operation involves case-sensitivity, you can use toLowerCase() method to prevent errors by converting the string data to lowercase:
let myString = `
firstname: ${firstName.toLowerCase()},
lastname: ${lastName.toLowerCase()}
`;
By following these, you’ll be better prepared to handle common issues in creating and working with multiline strings in TypeScript.
Final Thoughts
Mastering multiline strings in TypeScript is a vital tool for effective coding. Understanding how to use backticks and template literals to create multiline strings will help streamline your code, making it cleaner and more readable.
This tool is especially handy when dealing with large blocks of text or when you need to embed variables directly within your strings. This means fewer mistakes and easier debugging, which ultimately saves you time.
Furthermore, adopting such efficient coding practices makes your code more accessible to others, fostering better collaboration.
In the end, TypeScript, like any other programming language, is all about giving you the tools you need to create clean, efficient, and easy-to-understand code. And understanding how to create and manipulate multiline strings is a big part of that.
To learn more about how you can use scripting languages in your workflow, check out this video on Power Query/M language:
Frequently Asked Questions
In this section, you’ll find some frequently asked questions you might have when working with multiline strings in TypeScript.
How can I define a multiline string literal in TypeScript?
To create multi-line strings in TypeScript, simply use backticks (`) around your string instead of single or double quotes.
For example:
const myMultilineString = `This is a
multiline
string`;
What is the syntax for string interpolation across multiple lines in TypeScript?
In TypeScript, you can use string interpolation with the ${expression} syntax within multiline strings.
For instance:
const name = "John";
const multilineStringWithInterpolation = `Hello, ${name}.
This is a multiline
string with interpolation.`;
How can I create a multiline string without adding a newline in TypeScript?
You can join an array of strings using the join() method. This will create a multiline string without adding a new line character:
const myString = ["This is a", "multiline", "string"].join(" ");
In React with TypeScript, how can I represent a multiline string?
To represent a multiline string in a React component with TypeScript, simply return the string as a JSX expression using the backtick syntax:
const MyComponent = () => (
<>
{`This is a
multiline
string in a React component`}
</>
);
How do I utilize multiline code in TypeScript?
To use multiline code in TypeScript, simply write your code within backticks as a multiline string. This is useful for inline JavaScript templates or embedding other multiline content.
const myMultilineCode = `function example() {
console.log("This is multiline code");
}`;
Are there any differences between multiline strings in TypeScript and JavaScript?
There are no major differences between multiline strings in TypeScript and JavaScript. Both languages support using backticks for defining multiline strings and string interpolation.