Arrays are one of the most fundamental and versatile data structures in JavaScript, and often, developers find themselves needing to adjust the size of these arrays, particularly by removing elements from the end.
In JavaScript, to remove the last element from an array, you can use the pop() method. This method modifies the original array by removing its last element and returning that element.
For instance, if you have an array let fruits = [‘apple’, ‘banana’, ‘cherry’];, calling fruits.pop(); would remove ‘cherry’ from the array.
Other techniques, like splice() and slice(), can also change arrays to meet your needs.
In this article, we’ll walk you through the straightforward process of trimming your arrays, ensuring you have the tools to keep your data clean and efficient.
Let’s get started!
4 Methods to Remove Last Array Element in JavaScript
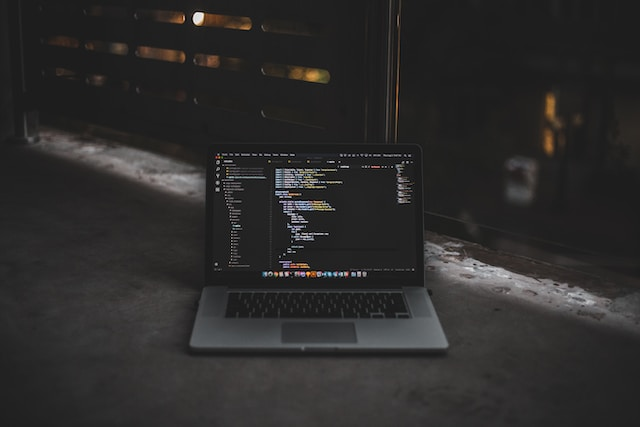
Arrays are foundational structures in JavaScript, and manipulating their contents is a frequent task for developers.
From the direct pop() method to the versatile splice() and even the non-destructive slice(), we’ll explore each technique’s nuances and applications.
Specifically, we’ll look at four methods with practical examples:
- pop(): Directly removes and returns the last element of an array.
- splice(): A versatile method that can remove (or add) elements from an array based on specified indices.
- slice(): Creates a new array by copying a portion of the original, allowing for removal without altering the initial array.
- length: By adjusting the length property of an array, we can implicitly remove the last element.
1. Using pop() to Remove Elements
The pop() method is the most direct way to remove the last element from an array.
When invoked, it modifies the original array by taking off its last element and returns that very element.
The pop() method doesn’t require any arguments and is called directly on the array you wish to modify.
The following example illustrates how the pop() method works:
This example removes the last member from array using pop().
The removedElement variable stores the deleted element and returns the element’s value.
The output in this case will be:
Note: pop() modifies the original array and returns it without the last ‘n’ elements you choose.
If you want to retain the original array’s structure, you’d need to look at non-destructive methods.
2. Using splice() to Remove Elements
Splice() is a powerful JavaScript array method that lets you remove elements and add or replace components.
The syntax for the splice() method is as follows:
array.splice(index, howmany, item1, ..., itemX)
It takes three parameters:
- index: The position at which the change starts.
- howmany: The number of elements to be removed (0 indicates that no elements will be removed).
- item1, item2, …, itemX: Optional parameter. The elements to add to the array from the index position.
For example, given an array of fruits, let’s remove the last element of the array:
In the above, the splice() method removes the last element, ‘date’, from the fruits array, leaving the modified array with three new elements each: ‘apple’, ‘banana’, and ‘cherry’ as you can see in the output below:
3. Using slice() to Remove Elements
The slice() is yet another array method that lets you remove components from an array.
It takes two parameters:
- start: The starting position.
- end: The number of elements to be removed.
The syntax for the slice() method is as follows:
array.slice(start, end)
By using the slice() method, you can remove the last element of an array by providing a negative index as the second parameter.
For example:
We pass 0 as the start index to start the new array with the first entry.
The second parameter, -1, excludes the last element.
The output will be:
JavaScript’s slice() method is a fast way to extract a part of an array without changing its contents.
With a solid grasp of its use, it becomes a vital tool for array manipulation.
The slice() method will create a new array without altering the original.
Pass the starting and end indices to slice() to remove the last element.
The end index is not in the and returns a new array name.
4. Using Length Property
The length property in JavaScript is useful for manipulating arrays because it can count array elements and alter them to meet certain needs.
It’s a less conventional but effective method that involves adjusting the length property of an array.
By decrementing the array’s length by one, you effectively remove the last element.
To remove the last entry from an array, you can directly edit its length property. Set array.length = array.length – 1 to lower the array size and remove the last entry.
As an example:
The output for the above code is:
This method is simple, however, it may cause performance concerns when processing huge arrays. Using pop() is a faster alternative.
Final Thoughts
Navigating the world of JavaScript arrays can initially seem like a maze, but with the right tools and understanding, it becomes a structured path leading to efficient coding.
Removing the last element of an array, a seemingly simple task, offers a glimpse into the depth and versatility of JavaScript.
The methods we’ve explored — pop(), splice(), slice(), and manipulating the length property — each have their unique strengths and contexts where they shine.
As you continue to develop and refine your coding skills, it’s essential to not just know these methods but to understand when and why to use each one.
Embrace the learning process, experiment with different approaches, and always strive to deepen your understanding. Happy coding!
If you’d like to learn more about how to manipulate data structures for data analysis, check out our comprehensive playlist on Power BI below:
Frequently Asked Questions
How to delete the final entry in a JavaScript array without using pop?
To remove the last element of a JavaScript array without using the pop() method, you can use the splice() method.
Here’s an example:
Get the last item in a JavaScript array and delete it?
To get the last item of an array and then delete it, you can use the pop() method. Here’s an example:
How to remove the end or last element of an array in JavaScript?
You can remove the last element of an array in JavaScript using the pop() method:
How to remove the last element of an array without mutating the original array in JavaScript?
To remove the last element of an array without mutating existing elements in the original array, you can use the slice() method: