Functions are a big part of the Javascript language as they help ensure code modularity and reusability.
In most of these functions, you’ll find a keyword known as the “return” statement, which plays a crucial role in managing the flow of your code.
The return statement signifies the end of a function and returns a particular value to the function’s caller. Once the function’s code reaches the return statement, it stops executing and returns the result to the caller. Every other code written after this value in the function will be unreachable.
In this article, we’ll show you the different ways to use this important keyword in your code. We’ll also go through its proper syntax, structure, and some pitfalls to avoid when using it.
Let’s rock and roll!
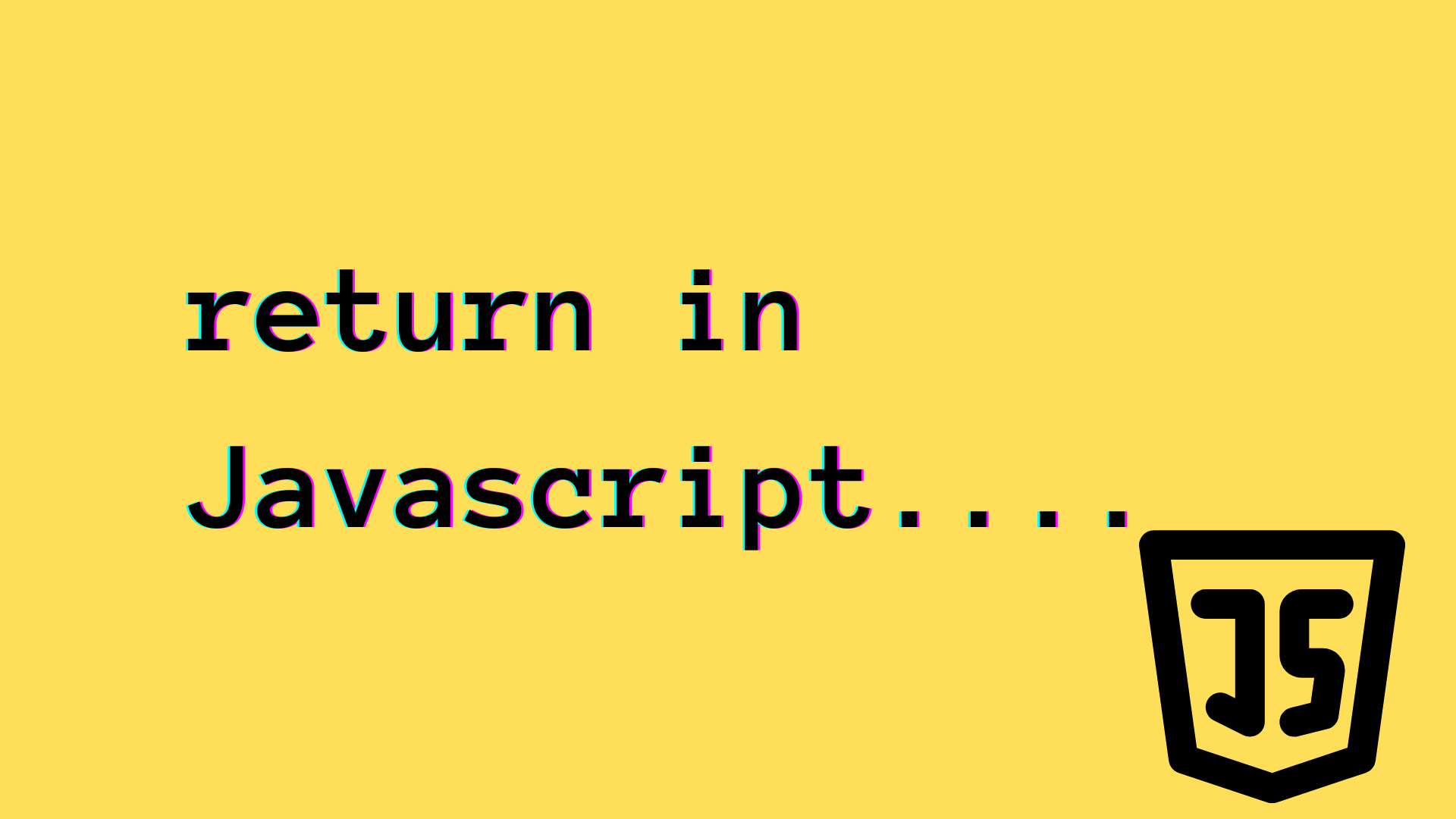
Understanding the ‘Return’ Command
In JavaScript, the return command plays a crucial role when working with functions. When you create a function, it’s common to use the return statement to provide a value that the function generates or processes.
The return statement stops the execution of a function and returns a value to the function caller. In essence, the return statement acts as a bridge between the function’s internal processing and the external code that called the function.
Basic Return Command Syntax
function <func-name>{
//code to be executed
return <expression|variable>
}
In the function above, after the code in the function is executed, the result variable is returned to the function caller. This variable can be stored in another variable, used in a calculation, or printed out to a console.
For example, let’s explore a simple JavaScript function that adds two numbers together:
function add(a, b) {
return a + b;
}
var sum = add(2, 3);
console.log(sum);
Output:
5
In this example, the add function takes two parameters a and b. The return statement in the function body combines a and b with the addition operator and returns the result.
So, when you call the add function with the arguments 2 and 3, it returns 5, which is then stored in the variable sum.
If you don’t include a return statement in your function, or if you don’t specify a value to be returned, JavaScript will implicitly return an undefined value. Here’s an example to illustrate that:
function noReturnValue() {
console.log("This function doesn't return a value.");
}
var result = noReturnValue();
console.log(result);
Output:
In this example, the noReturnValue function does not include a return statement. Therefore, when the function is called, and its result is assigned to the variable result, you’ll see that the return value is undefined.
What Can A Javascript Function Return?
There are many ways you can use the return statement inside of a Javascript function for various applications. Let’s look at some of them:
Primitive Values
We can use the return statement to return primitive values like strings, numbers, booleans, null, etc. To return these values, we just append them to the return keyword.
For examples:
function num(){
return 47
}
function str(){
return "Hello, world!"
}
function bool(){
return true
}
console.log(num())
console.log(str())
console.log(bool())
Output:
47
Hello, world!
true
Object Types
A Javascript return statement can also return datatypes like objects, dates, and data structures like arrays. Let’s look at an example of this:
function getCurrentDate(){
return new Date()
}
console.log(getCurrentDate())
Output:
This simple function returns the current date. We can create more complex functions using the Date module and functions with the return value.
Let’s look at another example with an array:
function streets(){
let street1 = 'Rose avenue'
let street2 = 'Forest hills drive'
let street3 = 'Brampton Crescent'
return [street1, street2, street3]
}
console.log(streets())
Output:
[ 'Rose avenue', 'Forest hills drive', 'Brampton Crescent' ]
Expressions
You can also place expressions in your Javascript return statements. These expressions can be Boolean or even Arithmetic. They will be resolved within the return statement and the answer will be returned to the function’s caller.
Here’s an example:
function interest(principal, rate, time){
return principal * rate * time * 0.01
}
console.log(interest(1000, 5, 3))
Output:
150
In the example above, the interest was calculated right inside the return statement using the mathematical equation. Now, the function will return the interest to the function caller. Let’s look at some boolean expressions:
function isEven(num){
return num%2 == 0
}
console.log(isEven(27))
console.log(isEven(56))
console.log(isEven(22))
console.log(isEven(17))
Output:
false
true
true
false
This example evaluates if the function’s argument is even using the boolean expression.
No Values
Yes, you read that right! The payload of your return statement can be empty. You can choose not to return any value to the function caller from your Javascript function.
Let’s look at an example:
function print(){
console.log("The function has run")
return;
}
var result = print()
console.log(result)
Output:
As we can see from the code above, the function runs, but it doesn’t return anything to the function caller. As a result, the function automatically returns undefined.
This particular function of the return statement is very useful for interrupting the execution of a function. You can use it to break out of the function and return control to the caller once a particular condition has been met.
Multiple Values
A single Javascript function can return multiple values at once by using an Array or a function. You can assign each of these values to a variable by using an array or object destructuring. Let’s take a look at how this works below:
function return_multiple(){
const name = "Kimberly"
const age = 20
const job = "Doctor"
return [name, age, job]
}
const [p_name, p_age, p_job] = return_multiple()
console.log(p_name, p_age, p_job)
Output:
As we can see we have retrieved multiple values and stored them in different variables using an array.
Also, most people prefer using an object when returning multiple values because the variable names don’t have to be in order when you specify the right keywords.
Common Pitfalls of the Return Statement
When using the return statement, there are some particular mistakes you should avoid making as they would result in code errors. Some of these mistakes include:
Dead Code
As we said earlier, the return statement stops function execution. So, any function code written after the return statement will not execute. For example:
function test(){
console.log('I will execute!')
return True
console.log("I will not execute")
}
test()
Output:
As we can see in the result, the first logging statement executes, but the second one after the return statement doesn’t. So, avoid placing code after your return statement as it will not run.
Multi-Line Return Statements
Always try to keep your return statement on a single line. If you spread out the statement over multiple lines, you might get an error. Let’s use our earlier interest function to illustrate this example:
function interest(principal, rate, time){
return
principal * rate * time * 0.01
}
console.log(interest(1000, 5, 3))
Output:
When we run the program, it’s going to return undefined as if the return statement is empty.
To avoid this, keep your return statement on the same line. If the statement is too long and you can’t keep everything on the same line, you can wrap the statement in brackets.
Let’s correct the example in the previous code below:
function interest(principal, rate, time){
return (
principal * rate * time * 0.01
)
}
Now, the function will run correctly.
Final Thoughts
So now you know, the return statement in JavaScript is a powerful tool that allows you to control the flow of your functions and return values to the caller. By mastering its usage and understanding its impact, you can write code that’s more efficient and maintainable.
Now, you should be able to harness the potential of the return statement and elevate your JavaScript programming skills to the next level. Good Luck!
If you’re looking for more great Javascript tutorials, check out this article on How to Print in JavaScript: 4 Quick Ways + Examples. You should also check out this video on how you can integrate GPT4 into your data analytics workflow!
Frequently Asked Questions
Does an arrow function need a return statement?
You can use return statements within an arrow function, but they are not necessary. If your function consists of only one line, you can skip the return statement. For example:
function add1(num1, num2) => {
return num1 + num2
}
function add2(num1, num2) => num1 + num2
Both of the functions above are the same, but the second one is more concise and cleaner without the return statement.
Can I have multiple return statements in one Javascript function?
Yes, it’s possible to have multiple return statements in one function, especially if you are using if else statements. You can have return statements for each specific condition, so when the condition is met, the function will return the right value to the caller and stop executing.
Can I return a function with parameters in JavaScript?
You can return a function with parameters from another function by using an inner function. For example:
function createAdder(x) {
return function(y) {
return x + y;
};
}
const add5 = createAdder(5);
const result = add5(3);
console.log(add5)
consol.log(5)
Output:
In the example above, the createAdder function returns an anonymous function. To use it, we store the function in the add5 variable and call it with 3.