Python is popular among developers and data specialists because it simplifies text manipulation. Slicing string is an important feature in text manipulation that’s often used when preprocessing data from machine learning models. With Python, slicing string is simple and requires a basic understanding of indexing and strings in Python.
To slice a string in Python, you can use the slice() method or employ the array slicing with the [::] notation. Both approaches rely on providing start and end positions, along with an optional step, to define the desired substring. Furthermore, Python supports positive and negative indexing, which allows you to work flexibly with strings regardless of their length or complexity.
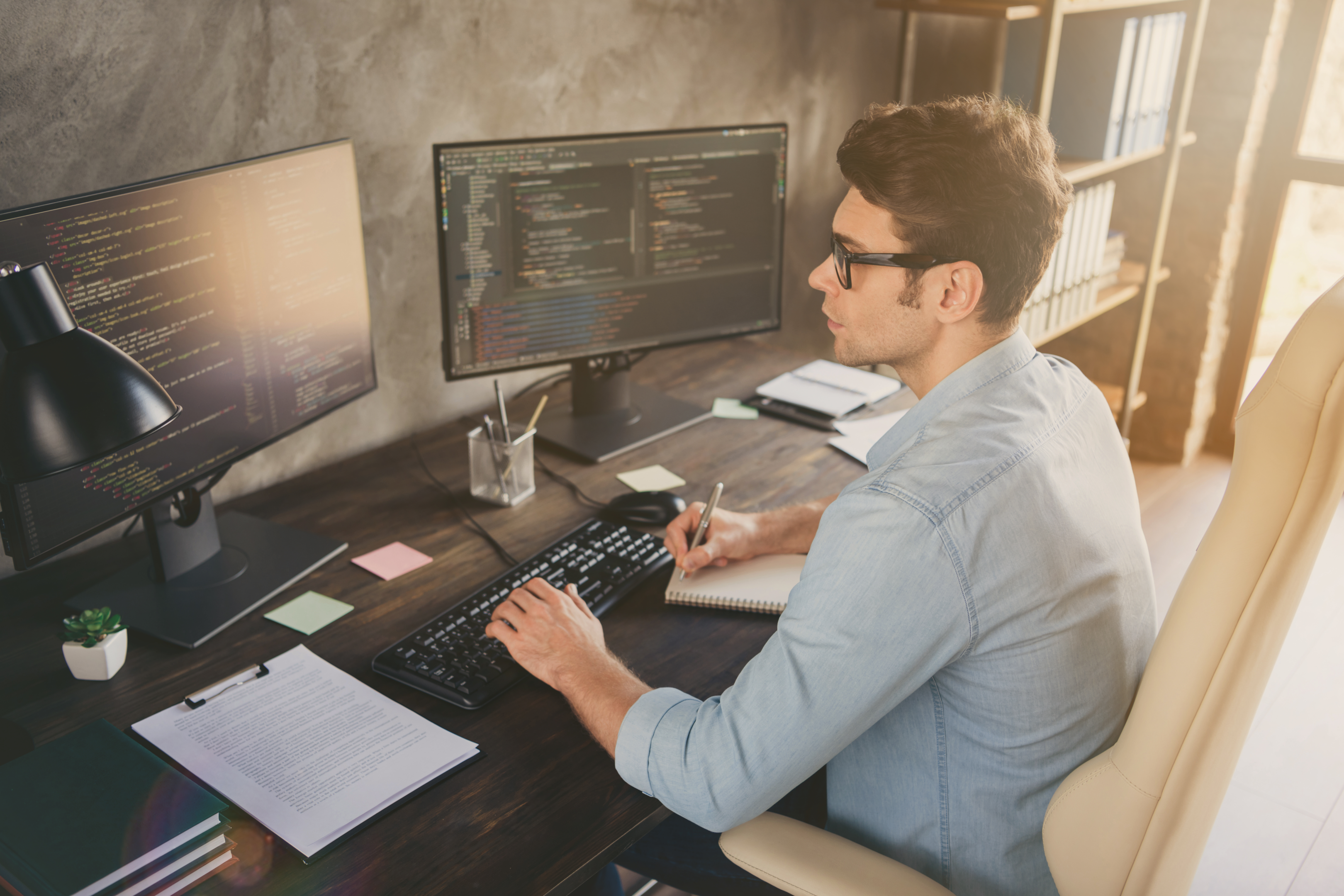
In this article, we’ll explore string slicing in Python, including its syntax, common usage scenarios, and possible pitfalls. With a clear understanding of string slicing, you can unlock the full potential of Python’s string manipulation capabilities.
Let’s get into it!
Understanding the Basics of Slicing Strings
Before we start writing code for Python string slicing, it’s crucial that you understand the following 2 concepts:
- Python String
- String Indexing
The above 2 concepts lay the foundation for slicing stings, therefore, a solid understanding of the above concepts is important.
1) What is a Python String?
A string in Python is a sequence of characters. It’s a type of data that’s used to represent text rather than numbers.
A Python string is immutable, and slicing one creates a new substring from the source string and the original string remains unchanged.
In Python, you can define a string by enclosing a sequence of characters within single or double quotes.
my_string = "Hello, world!"
In the above example, “Hello, world!” is a string. It’s stored in the variable my_string.
Python strings are iterable objects, meaning you can loop through each character in the string using a for loop or a while loop in combination with an iterator.
2) What is String Indexing?
String indexing allows you to access individual characters in a string using their position, also known as the index.
The index positions of a string are always numbered. The start index is 0 for the first character and increments by 1 for each subsequent character.
Indexing can be of two types:
- Positive Indexing
- Negative Indexing
1) What is Positive Indexing?
In positive indexing, the indexing of the elements starts from 0 for the first element from the left or the beginning of the sequence. The second element will have an index of 1, the third element will have an index of 2, and so on.
The following is an example of positive indexing:
my_string = "Hello"
print(my_string[0]) # Output: H
print(my_string[1]) # Output: e
print(my_string[2]) # Output: l
print(my_string[3]) # Output: l
print(my_string[4]) # Output: o
As you can see, the indexing starts from 0 and increases by 1 for each subsequent element.
2) What is Negative Indexing?
Python slice works with negative indexing as well. Negative indexing is the concept where the indexing of elements starts from -1 for the last element from the right or end of the sequence.
The second last element will have an index of -2, the third last will have -3, and so forth.
Negative indexing is used to access elements from the end of the sequence.
The following is an example of negative indexing:
my_string = "Hello"
print(my_string[-1]) # Output: o
print(my_string[-2]) # Output: l
print(my_string[-3]) # Output: l
print(my_string[-4]) # Output: e
print(my_string[-5]) # Output: H
As you can see, negative indexing starts from -1 at the end of the string and decreases by 1 for each element moving toward the start.
How to Slice a String in Python
In Python, there are 2 ways to slice a string.
- Using Slice Notation
- Using the slice() Function
In this section, we’ll go over the syntax and explanation of each method. There will be examples for each method to help you better understand the concepts.
1) How to Slice a String Using Slice Notation
This is the most common and concise way to slice a string in Python.
The syntax for slice notation is given below:
str[start:stop:step]
In Python’s slice notation str[start:stop:step],:
- start denotes the starting position of the slice operation.
- stop is the index where the slicing should end. The stop index is exclusive, meaning the slice does not include this index.
- step indicates the increment between each index for slicing; for example, a step of 2 would take every other character. This is an optional parameter.
It’s important to note that using a second index that’s less than or equal to the first index will result in an empty string.
5 Examples of Slicing String Using Slice Notation
Now that you’ve learned the syntax and parameters of the slice notation, let’s look at some examples of string slice using slice notation.
Example 1: Extracting a Substring
Let’s say you have a string, and you want to extract a portion of it.
You can use slicing to do this:
str = "Hello, world!"
print(str[0:5])
In the above code, we are trying to extract Hello from the string. The output of the above script will be:
Example 2: Reversing a String
You can use slicing notation to reverse a string.
Let’s say we want to reverse a string. we can do this by setting the step parameter to -1, which means “step backward by one element”.
str = "Hello, world!"
print(str[::-1])
The above code will reverse the string as shown below:
Example 3: Getting Every nth Character
You can use the step parameter to get every nth character from the string.
For example, here’s how you can get every second character:
str = "Hello, world!"
print(str[::2])
The above code will print every second character from the string as shown below:
Example 4: Removing the last character
Sometimes, you might want to remove the last character from a string.
You can do this with the following example code:
str = "Hello, world!"
print(str[:-1])
The above code will remove the last character from the string which is ‘!’. The output is shown below:
Example 5: Getting the last n characters
If you want to get the last n characters from a string, you can use negative indexing with slicing.
The following code demonstrates this method:
str = "Hello, world!"
print(str[-5:])
The above code will output the last 5 characters of the string as shown below:
2) How to Slice a String Using slice() Function
The slice() function is a built-in function that creates a slice object using the slice constructor. It can be used to slice a string (or a list, or any other sequence type).
The slice() function takes the same start, stop, and step parameters as slice notation.
The syntax for slice function is given below:
slice(start, stop, step)
4 Examples of slice() Function
In this section, we’ll look at 5 examples of slice() function for slicing a Python string.
Example 1: Extracting a Substring
Say you have a timestamp in the format HH:MM:SS, but you’re only interested in the hours and minutes. You can use the slice() function to extract that specific part of the timestamp.
The following code demonstrates this method:
timestamp = "12:30:45"
slice_obj = slice(0, 5)
hours_and_minutes = timestamp[slice_obj]
print(hours_and_minutes)
The above code will extract the first five characters of the string, i.e., “12:30”
Example 2: Reversing a String
Reversing a string can be useful in a variety of applications.
For example, in DNA sequencing, the reverse complement of a DNA sequence is often needed.
The following is an example of this method:
dna_sequence = "AGTCCG"
slice_obj = slice(None, None, -1)
reversed_dna_sequence = dna_sequence[slice_obj]
print(reversed_dna_sequence)
The output will be:
Example 3: Removing the Last Character
This can be handy when dealing with strings where you want to ignore the last character.
For instance, sometimes data is delivered with a trailing comma or other punctuation that you want to remove.
data = "123,456,789,"
slice_obj = slice(None, -1)
cleaned_data = data[slice_obj]
print(cleaned_data)
The output will be:
Example 4: Getting the Last ‘n’ Characters
This can be useful for numerous tasks. For example, if you have a list of filenames and you want to get the file extension, which is typically the last three characters, you can use this method.
The following code demonstrates this method:
filename = "image.png"
slice_obj = slice(-3, None)
file_extension = filename[slice_obj]
print(file_extension)
The above code will print ‘png’ to the console:
Learn more about text processing in Python by watching the following video:
Final Thoughts
Understanding how to slice strings in Python opens up a range of possibilities for data manipulation and exploration. Whether you’re aiming to extract specific substrings, sample your data, or reverse a string, Python’s slicing offers a convenient, concise, and efficient method.
As you dive deeper into Python programming, you’ll discover that slicing isn’t just limited to strings. It extends to other sequence types such as lists and tuples. The underlying concepts that you learn here will be applicable to other iterables as well.
Frequently Asked Questions
What is the syntax for string slicing in Python?
In Python, the syntax for string slicing is: str_object[start_pos:end_pos:step].
The slicing starts at the start_pos index (inclusive) and ends at the end_pos index (exclusive).
The step parameter is optional and is used to specify the number of steps to take between characters. If not provided, the default step value is 1.
How can you reverse a string using slicing?
You can reverse a string in Python using slicing by specifying an empty start and end position with a step of -1.
For example:
text = "Hello, World!"
reversed_text = text[::-1]
reversed_text will now hold the value !dlroW ,olleH.
How do you slice a string with a negative index?
Negative indexing in Python allows you to access elements from the end of a sequence, like a string, by counting backward.
To slice a string using a negative index, use the same syntax as before but with negative index values.
For example:
text = "Hello, World!"
sliced_text = text[-5:-1]
sliced_text will now hold the value orld.
What are some common applications of string slicing?
String slicing is a versatile technique in programming languages, and its applications include:
- Extracting substrings from a larger string.
- Reversing strings.
- Modifying certain sections of a string without changing the whole string.
- Parsing data from structured strings (e.g., log files).
How does the step argument work in string slicing?
The step argument in string slicing determines the number of indices between characters in the sliced string.
For example, with a step of 2, every second character between the start and end positions will be included.
A negative step value will reverse the order of characters in the slice. If the step argument is not provided, it defaults to 1.
text = "abcdef"
sliced_text = text[1:5:2]
sliced_text will now hold the value bd.