Lists are important and frequently used data structures in Python. They are common in data analysis, machine learning, and game development applications. When working with lists, you’ll need to carry out various operations to meet your requirements. One such operation is that of concatenating lists.
To concatenate lists in Python, the simplest approach is to use the ‘+’ operator, which appends elements of one list to another. However, there are other methods like list unpacking, extend(), and list comprehension that caters more to certain use cases.
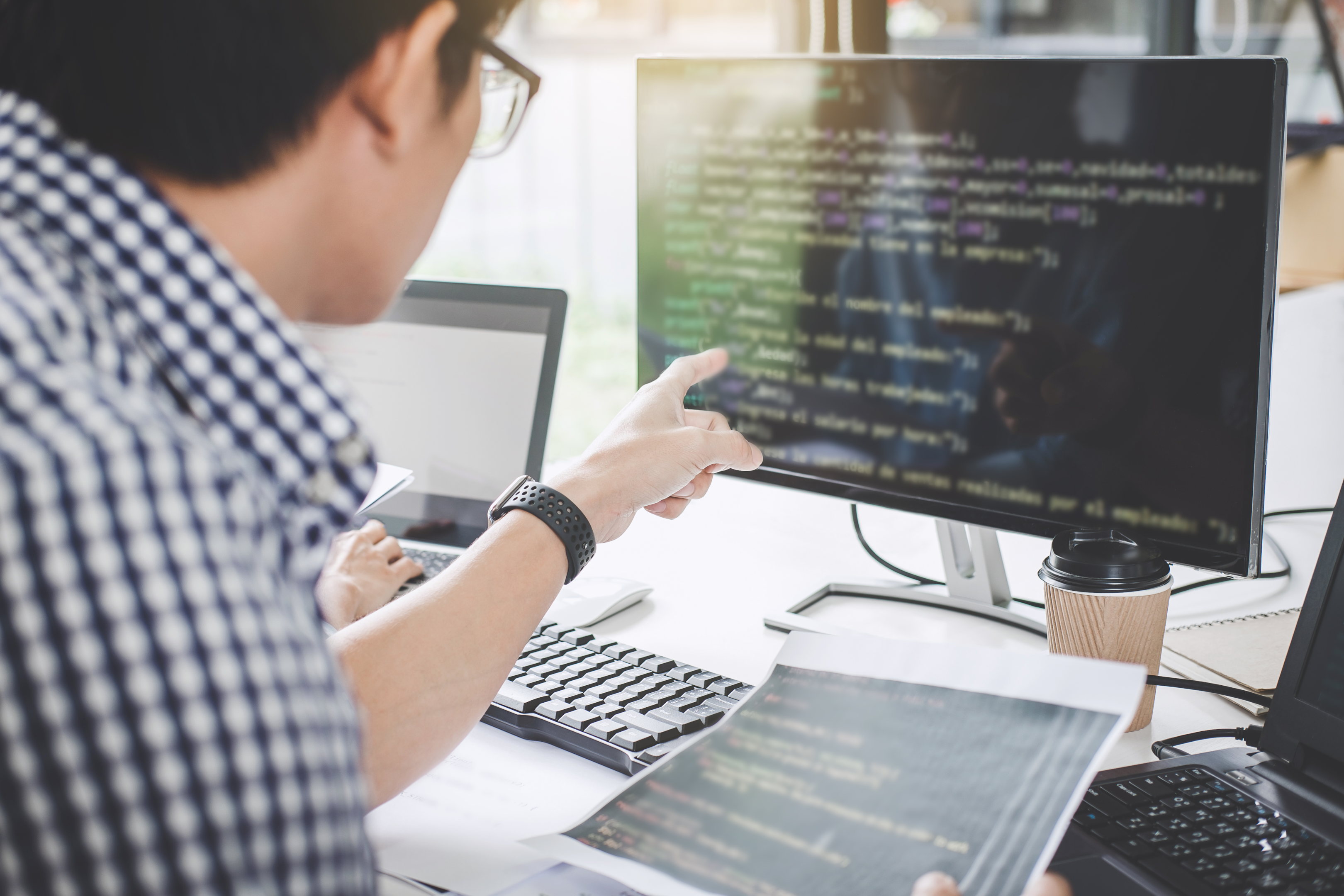
Throughout this article, we’ll explore 8 methods of concatenating lists in Python. We’ll examine their advantages and disadvantages to help you determine the most suitable approach for your particular project. The examples provided demonstrate achieving different outcomes based on your specific requirements.
Let’s get into it!
What is a Python List?
Before we dive into the various methods for concatenating lists in Python, let’s review the basics of Python lists. This will help you better understand the concepts explained in this blog.
Python lists are a particular data structure that allows you to store multiple elements in an ordered and mutable way.
You can create a Python list by placing elements inside square brackets, like numbers, strings, or other objects.
We’ve listed some important pointers about lists below:
- Mutable: Lists in Python are mutable, meaning you can change their content without changing their identity. You can modify a list by adding, removing, or changing all the elements.
- Ordered: Lists maintain the order of elements as they were initially added. They are not sorted automatically. The order of elements matters in a list and can be used to access and manipulate elements.
- Indexing: List elements are accessed by their index. Python uses zero-based indexing, which means that the first element is at index 0, the second at index 1, and so forth. You can also use negative indexing to access elements from the end of the list (-1 refers to the last item, -2 refers to the second last item, etc.).
- Duplicates Allowed: Lists can contain duplicate elements. They also support elements of different data types (e.g., integers, strings, and other lists).
- Size Flexibility: Lists can grow or shrink dynamically based on the operations performed on them.
8 Methods for Concatenating Lists in Python
In this section, we’ll explore 8 ways to concatenate lists in Python. Each method will be followed by examples to help you better understand the concept.
Specifically, we’ll go over the following ways of concatenating lists in Python:
- Using the ‘+’ Operator
- Using the ‘extend()’ Method
- Using List Comprehension
- Using the ‘append()’ Method in a Loop
- Using the ‘itertools.chain()’ Function
- Using the ‘+= operator’
- Using numpy’s ‘concatenate()’ Function
- Using List unpacking
1) How to Concatenate Lists Using ‘+’ Operator
The most conventional method for concatenating lists is using the ‘+’ operator. The ‘+’ operator in the context of lists works as a concatenation operator, combining two or more lists into a new one without altering the original lists.
The new list is a linear sequence of elements from the first list followed by those of the second list.
The following is an example of concatenating lists using the ‘+’ operator:
# define two lists
list1 = [1, 2, 3]
list2 = [4, 5, 6]
# use the '+' operator to concatenate the lists
combined_list = list1 + list2
# print the result
print(combined_list)
This code will output concatenated list as shown below:
When to Use ‘+’ for Concatenation
The ‘+’ list concatenation operation is perfect when you’re merging a small number of lists and want to keep the original lists unchanged.
However, it’s important to remember that this method may not be the most efficient for large lists or two or more lists due to its memory usage.
It creates a new list, leaving the originals intact, but for large-scale operations, methods like extend() or itertools.chain() might be more efficient.
2) How to Concatenate Lists Using the ‘extend()’ Method
The extend() function in Python adds all the elements of a list (or any iterable), to the end of the current list. Unlike the ‘+’ operator, extend() modifies the original list.
The following is an example of concatenating lists using the extend() method:
# define two lists
list1 = [1, 2, 3]
list2 = [4, 5, 6]
# use the 'extend' method to add elements of list2 to list1
list1.extend(list2)
# print the result
print(list1)
This will output the list concatenated in an end-to-end manner:
When to Use the extend() Method
The extend() method in Python is beneficial when you wish to append the contents of an iterable to an existing list while being unconcerned about preserving the original list. This method modifies the initial list in place, which offers an advantage in terms of memory efficiency, particularly with larger lists or a substantial number of lists.
Extend() is preferable to the ‘+’ operator in such scenarios as it doesn’t create a new list but modifies the existing one.
3) How to Concatenate List Using List Comprehension
List comprehension method in Python is a compact way of creating a list from sequences. You can use it for concatenating lists, where it can iterate over multiple lists and collate their items.
To concatenate two lists in Python using list comprehension, you can use the following code:
# define two lists
list1 = [1, 2, 3]
list2 = [4, 5, 6]
# use list comprehension to concatenate the lists
combined_list = [item for sublist in (list1, list2) for item in sublist]
# print the result
print(combined_list)
In the above example, the outer loop (for sublist in (list1, list2)) iterates over the tuple of lists, and the inner loop (for item in sublist) iterates over each list item.
When to Use List Comprehension
You can use list comprehension when you require more than simple merging. It allows you to incorporate conditions or apply functions to items as they’re concatenated, thereby allowing more complex operations within a single line of code. This makes your code cleaner and more readable, especially when dealing with small to medium-sized lists.
4) How to Concatenate Lists Using the ‘append()’ Method in a Loop
The append() method in Python adds a single item to the end of the list.
To use append() to concatenate lists, you need to use it in a loop to add each element from the second list to the first list individually.
The following is an example of this method:
# define two lists
list1 = [1, 2, 3]
list2 = [4, 5, 6]
# use the 'append' method in a loop to add elements of list2 to list1
for item in list2:
list1.append(item)
# print the result
print(list1)
The output will be:
In this case, each item from list2 is appended to the end of list1, resulting in the concatenated list. The append() method modifies the original list (list1 in this case).
When to Use the append() Method
You might use append() for concatenation when you want to add elements from one list to another within a loop that performs additional operations or checks on each element.
For instance, you might be filtering elements, or applying some function to elements, as you add them.
For straightforward list concatenation, other methods like extend() or itertools.chain() can be more efficient and are specifically designed for that purpose.
5) How to Concatenate Lists Using the ‘itertools.chain()’ Function
The itertools.chain() function is a method provided by Python’s itertools module. It is used to concatenate iterable objects like lists or other data structures like dictionaries in a memory-efficient way.
The following is an example of concatenating two lists in Python, using this method:
# import the itertools module
from itertools import chain
# define two lists
list1 = [1, 2, 3]
list2 = [4, 5, 6]
# use 'itertools.chain()' to concatenate the lists
combined_list = list(itertools.chain(list1, list2))
# print the result
print(combined_list)
In this example, itertools.chain(list1, list2) returns an iterable that produces the elements of list1 and list2 as if they were a single list. By passing this iterable to list(), we join lists together and create a new list that contains all of the elements from both list1 and list2.
When to Use the itertools.chain() Method
The itertools.chain() function is ideal for list concatenation in cases where memory efficiency is a significant concern.
This method becomes especially useful when dealing with a large number of lists or very large lists, as itertools.chain() doesn’t create an entirely new list but instead returns an iterator that can traverse over the original lists as if they were a single list.
This can greatly save memory resources compared to methods like ‘+’ or extend() that generate a new list.
6) How to Concatenate Lists Using the ‘+= operator’
In Python, the ‘+=’ operator is an inplace extension that can be used to concatenate lists, similar to using the extend() method. It modifies the original list by adding elements from another list to the end.
The following is an example of concatenating lists using this method:
# define two lists
list1 = [1, 2, 3]
list2 = [4, 5, 6]
# use the '+= operator' to concatenate the lists
list1 += list2
# print the result
print(list1)
In this example, list1 += list2 is equivalent to list1.extend(list2). It adds each element from list2 to the end of list1, modifying existing list in place.
When to Use the += Operator
The += operator is an excellent choice if you want to create a new concatenated list by appending another list to the original list and you don’t need to preserve the original lists.
This operator, just like the extend() method and modifies the original list in place.
7) How to Concatenate Lists Using NumPy’s ‘concatenate()’ Function
NumPy’s concatenate() function is a powerful tool for concatenating arrays along an existing axis. This method can be used for lists as well, but it returns a NumPy array instead of a list.
The following is an example of concatenating lists using the NumPy’s concatenate() function:
# import the numpy module
import numpy as np
# define two lists
list1 = [1, 2, 3]
list2 = [4, 5, 6]
# use 'numpy.concatenate()' to concatenate the lists
combined_array = np.concatenate((list1, list2))
# print the result
print(combined_array)
In this example, np.concatenate((list1, list2)) returns a NumPy array that contains all of the elements from list1 and list2. The input lists are passed as a tuple to the concatenate() function.
When to Use NumPy’s ‘concatenate()’ Function
This method is particularly useful when you’re working with numerical data and you want to take advantage of the benefits that NumPy arrays offer over lists, such as a wider range of operations, more efficient numerical computations, and the ability to handle multi-dimensional data.
8) How to Concatenate Lists Using List Unpacking
List unpacking is a Python feature that allows you to extract elements from a list or other iterable. You can use it to concatenate lists by creating a new list and unpacking the contents of the existing lists into it.
The following is an example of concatenating lists using this method:
# define two lists
list1 = [1, 2, 3]
list2 = [4, 5, 6]
# use list unpacking to concatenate the lists
combined_list = [*list1, *list2]
# print the result
print(combined_list)
In this example, the expression [*list1, *list2] creates a new list and unpacks the elements of list1 and list2 into it. The * operator is used to unpack the lists.
Learn more about handling data in Python by watching the following video:
Final Thoughts
As we wrap up, let’s consider why understanding list concatenation in Python is important for you as a programmer. Simply put, list concatenation is one of the most common operations in Python programming. It allows you to merge, manipulate, and transform your data. When you’re dealing with data in lists, you’ll find yourself needing to concatenate those lists in some way.
Mastering the different methods of list concatenation equips you with the flexibility to choose the right tool for different data structures. There is no best method because each method has its unique strengths, be it efficiency, readability, or the ability to handle complex tasks succinctly. Having a range of methods at your disposal enables you to write more efficient, readable, and appropriate code for your particular use case.
Frequently Asked Questions
How can I merge two lists in order?
To merge two lists in order, you can use the + operator:
list1 = [1, 2, 3]
list2 = [4, 5, 6]
merged_list = list1 + list2
You can also use the extend() method:
list1.extend(list2)
What is the most efficient way to combine multiple lists?
The most efficient way to combine multiple lists can be achieved through the built-in itertools.chain() function.
import itertools
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list3 = [7, 8, 9]
combined_list = list(itertools.chain(list1, list2, list3))
How do I merge nested lists?
To merge nested lists, you can use a list comprehension.
nested_lists = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
merged_list = [element for sublist in nested_lists for element in sublist]
How can I concatenate lists without duplicates?
To easily concatenate the lists without duplicates, you can use a set.
list1 = [1, 2, 3, 4]
list2 = [3, 4, 5, 6]
merged_list = list(set(list1 + list2))
How do I combine two lists into a 2D array?
To combine two lists into a 2D array, you can use the zip() function with a list comprehension.
list1 = [1, 2, 3]
list2 = [4, 5, 6]
result = [list(x) for x in zip(list1, list2)]
How do I merge lists vertically?
If you want to merge lists vertically (alternating elements from each list), you can use the zip() function and a list comprehension.
list1 = [1, 2, 3]
list2 = [4, 5, 6]
merged_list = [item for pair in zip(list1, list2) for item in pair]