Opening files is an important task in any programming language. With its built-in functions and straightforward syntax, Python makes it simple to work with files. If you want to open files, then the open() function is the answer.
Python provides the open() function for interacting with files. The function accepts two arguments: file name and access mode parameter. To open a file, simply specify the file name with its full path if it’s not in the same directory as the Python script and its access mode, such as ‘r’ for reading or ‘w’ for writing. If the file doesn’t exist, the open() function will create the file, depending on the access mode used.
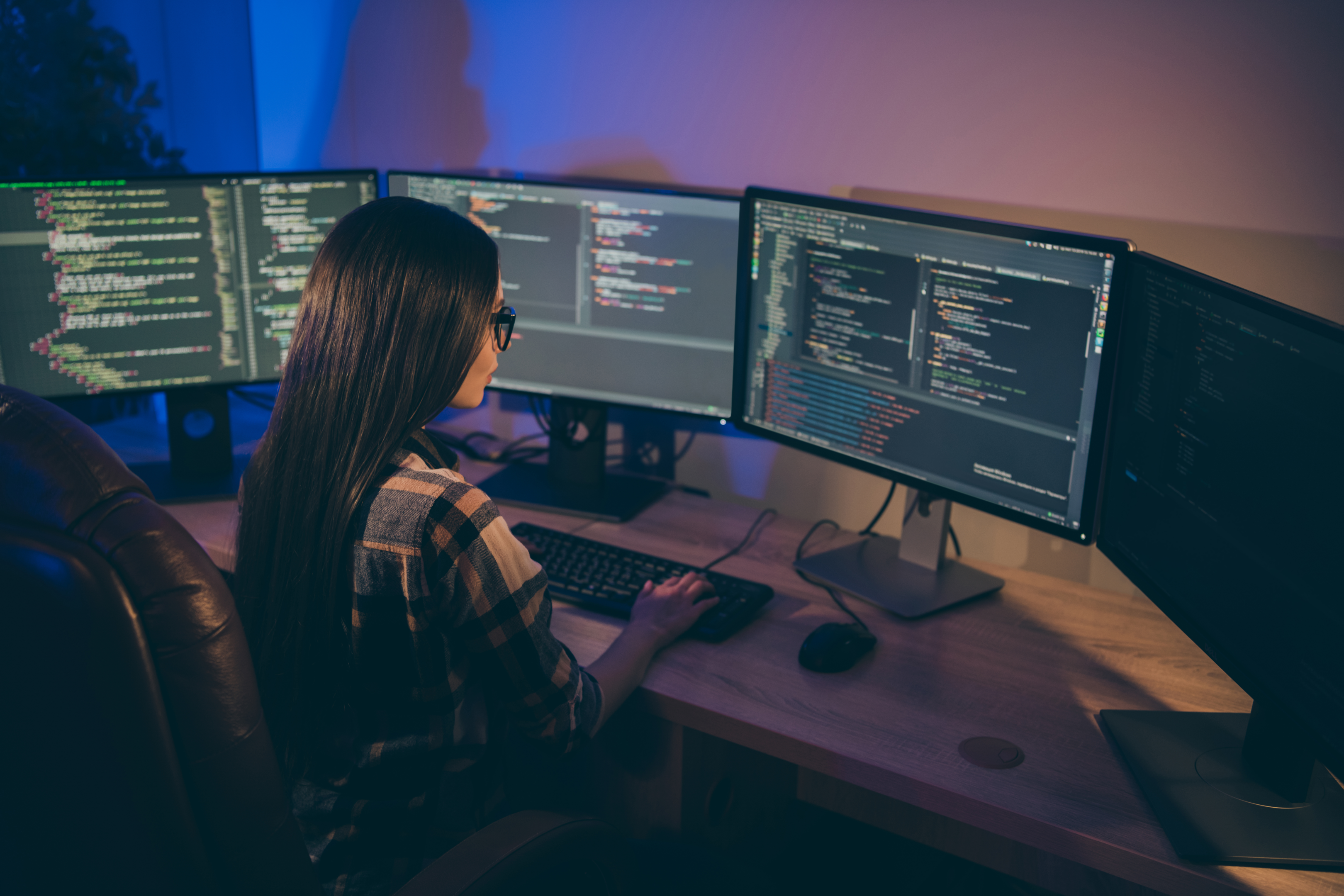
Throughout this article, you’ll learn more about the access modes, how to use them, and other aspects related to file handling in Python. Whether you’re new to programming or an experienced developer, knowing how to work with files is a skill that will prove useful in your projects.
Let’s get started!
What is the Syntax of the open() Function?
The general syntax of the open() function is as follows:
file_object = open(name, mode)
The parameters of the open() function are:
- name: The name of the file you want to open (including the file extension). You may use the file name directly if the file is located in the same directory as the Python script. If the file is located elsewhere, use the full path of the file.
- mode: The mode in which you want to open the file (as explained in the Open Modes section).
The following is an example of opening a text file for reading:
file_object = open("example.txt", "r")
To close the file after using it, call the close() method on the file object:
file_object.close()
What Are the Types of Open Modes?
When opening a file in Python, you can specify different modes depending on your needs.
The following are 6 common open modes:
- ‘r’: Read mode
- ‘w’: Write mode
- ‘a’: Append mode
- ‘x’: Exclusive creation mode
- ‘b’: Binary mode
- ‘t’: Text mode
In this section, we’ll discuss the 5 modes with examples to help you build a solid foundation in opening files in Python.
1. How to Open a File in Read Mode
In Python, read mode is used when you want to read data from a file without making any changes to it.
The mode is indicated by ‘r’.
If the file does not exist, an IOError will be raised.
The following is an example of how to open a file in read mode:
file_object = open("app.txt", "r")
You can use the read() method on the file object to read data from a file.
For instance:
data = file_object.read()
print(data)
The output will be:
If you need to read one line at a time, you can use the readline() method:
line = file_object.readline()
print(line)
When you’re done reading from a file, it’s important to close it using the close() method.
The following code shows how to close a file from Python:
file_object.close()
2. How to Open a File in Write Mode
In Python, the write mode is used when you want to create a new file or overwrite the contents of an existing file.
This mode is indicated by the ‘w’ character.
If the file does not exist, it will be created. If the file exists, it will be truncated to zero length before being opened for writing.
To open a file in write mode, you can use the code given below:
file_object = open("app.txt", "w")
The “w” in the open() function instructs the interpreter to open the file in write mode.
After creating the file handle, you can start writing to the file. To write data to a file, you can use the write() method as shown below:
file_object.write("This is an example text.")
After writing to the file, you can close the file connection with the following code:
file_object.close()
3. How to Open a File in Append Mode
The append mode allows you to add data to the end of the existing file.
Let’s say you want to open a file named app.txt and append the string “Hello, World!” to it.
You can use the following code to achieve this:
# Open the file in append mode
with open('app.txt', 'a') as file:
# Write a string to the file
file.write("Hello, World!\n")
In this code, the open() function is used with ‘a’ as the second argument to open the file in append mode. The with statement is used to manage the file context.
The write() method is then used to append the string “Hello, World!\n” to the file. The \n character is a newline, which means that each time you run this code, a new line will be started for the appended string.
After running the above code, the output will be:
As you can see, the append code added the text “Hello, World!” to the text document.
4. How to Open a File in Exclusive Creation Mode
In Python, the exclusive creation mode (‘x’) is used to create and open files for writing. If the file already exists, the operation will fail, preventing any existing file from being overwritten.
This is helpful when you want to ensure that the file you’re working with is new and doesn’t contain any previous data.
The following is an example of opening a file in exclusive creation mode:
try:
with open('example.txt', 'x') as f:
f.write('Hello, world!\n')
except FileExistsError:
print('File already exists.')
In this example, Python attempts to create and open the file example.txt.
If the file already exists, a FileExistsError is raised, and the error message ‘File already exists.’ is printed.
If the file doesn’t exist, it is created, the string ‘Hello, world!\n’ is written to it, and it is then closed when execution leaves the with statement.
5. How to Open a File in Binary Mode
In Python, binary mode is used when you’re working with non-text files like images, executables, etc.
This mode doesn’t interpret the data in any way, it just reads or writes the bytes as they are.
The binary mode has sub-modes to open a binary file. The sub-modes are:
- ‘rb’: Binary read mode
- ‘wb’: Binary write mode
- ‘ab’: Binary append mode
- ‘xb’: Binary exclusive creation mode
The following is an example of opening a file in binary mode:
with open('1.png', 'rb') as file:
data = file.read()
print(data)
# data now contains the binary content of 'example.jpg'
In this code, ‘rb’ as the second argument in open() is used to open the file in binary read mode. The read() function is then used to read the entire content of the file into the variable data.
The above code will read the entire file using a file pointer.
The output will be:
The output is binary data of the image.
6. How to Open a File in Text Mode
The text mode is the default mode for working with files. It’s used when you’re working with text-based files like a .txt file, .csv file, Python file, etc.
This mode automatically encodes and decodes the binary data to and from the character strings as you read and write.
The sub-modes of opening a file in text mode are:
- ‘rt’: Text read mode (default)
- ‘wt’: Text write mode
- ‘at’: Text append mode
- ‘xt’: Text exclusive creation mode
Even though the ‘t’ is the default mode and it is rarely used explicitly, it can be combined with any other mode like ‘r’, ‘w’, ‘a’, ‘x’, etc.
The following is an example of opening a file in text mode for reading:
with open('example.txt', 'rt') as file:
data = file.read()
In this code, ‘rt’ as the second argument to open() is used to open the file in text read mode. The read() function is then used to read the entire content of the file into the variable data.
How to Handle Exceptions When Opening Files
When working with files in Python, handling exceptions that might occur while opening, reading, or writing files is important.
This ensures that your code continues its execution smoothly.
When opening files, you can run into the following exceptions:
- FileNotFoundError
- PermissionError
Let’s look at how you can handle each of the above exceptions.
1. How to Handle FileNotFoundError Exception
One common exception you might encounter in file handling is the FileNotFoundError. This exception occurs when the file specified in the path does not exist or cannot be accessed.
To handle this exception, you can use the try-except block.
For instance:
import os
filename = "example_file.txt"
try:
with open(filename, 'r') as file:
content = file.read()
except FileNotFoundError:
print(f"File {filename} not found or inaccessible.")
In this example, we first import the os module, which provides several tools and functions for interacting with the operating system.
Then, we define the file path and attempt to open the file using the with open() as a construct.
If the specified file is not found or cannot be read, a FileNotFoundError is raised, and we print a message to inform the user about the issue.
2. How to Handle PermissionError Exception
Another possible exception when working with files is the PermissionError. This exception is raised when the operating system denies permission to access the file.
To handle this exception, simply extend the except block as follows:
import os
filename = "example_file.txt"
try:
with open(filename, 'r') as file:
content = file.read()
except FileNotFoundError:
print(f"File {filename} not found or inaccessible.")
except PermissionError:
print(f"Permission denied to access {filename}.")
Handling both FileNotFoundError and PermissionError ensures that your program can quickly identify the problem and provide a useful message to the user.
Working with Different File Types
In Python, you can work with different file types, including standard text, binary, CSV (comma-separated values), and even zip archives.
We will now give you a brief overview of handling different file types when opening files in Python.
1. How to Open Text Files
To open a text file, you use the open() function with the appropriate file name and mode, such as ‘r’ for reading or ‘w’ for writing.
The following is an example of opening a text file:
file_handle = open("example.txt", "r")
2. How to Open Binary Files
To work with binary files, you’ll need to use the ‘rb’ (read binary) or ‘wb’ (write binary) modes when opening the file.
The following is an example of opening a binary files:
file_handle = open("example.bin", "rb")
3. How to Open a CSV File
Python has a built-in csv module that simplifies working with CSV files.
To read data from a CSV file, you can open it in text mode, and use the csv.reader() function.
The following is an example of opening a csv file:
import csv
with open("example.csv", "r") as csvfile:
csv_reader = csv.reader(csvfile)
for row in csv_reader:
print(row)
4. How to Open ZIP Archives
ZIP is a popular compression format used to store multiple files within a single archive.
Python supports working with zip archives using the zipfile module.
To read data from a zip archive, open it in binary mode with the ‘rb’ flag, and use the ZipFile class.
The following is an example of opening a zip file:
import zipfile
with zipfile.ZipFile("example.zip", "r") as zip_archive:
for file in zip_archive.namelist():
print(file)
Learn more about file handling in Python by watching the following video:
Final Thoughts
Understanding how to properly open, read, and write to files is an indispensable skill. This is because it’s a common task, whether you’re logging data from a sensor, reading configuration files, or writing results from data analysis.
File handling allows you to interact with local files on your machine and can serve as a stepping stone to working with more complex data storage systems.
Moreover, the principles you’ve learned here can be applied to various tasks. The functions we’ve discussed are simple yet powerful, giving you the flexibility to easily handle text and binary data.
Frequently Asked Questions
What is the syntax to open a file in Python?
To open a file in Python, you can use the open() function.
The general syntax for file operation is:
file_object = open("file_name", "access_mode")
The file_name is the name of the file you want to open, and the access_mode determines how the file will be accessed (e.g., read, write, etc.).
How can I read Python files line by line?
To read a file line by line in Python, you can use a for loop in combination with the open() function:
with open("file_name", "r") as file:
for line in file:
print(line)
This method will read each line in the file and print it to the console.
What are the different file open modes in Python?
In Python, you can open a file in various modes:
- “r”: Read mode, opens the file for reading and raises an error if the file does not exist.
- “a”: Append mode, opens the file for appending and creates the file if it does not exist.
- “w”: Write mode, opens the file for writing and creates the file if it does not exist.
- “x”: Exclusive creation mode, creates the file but raises an error if the file already exists.
How do I write to a file using Python?
To write to a file using Python, you can use the write() method with the “w” or “a” access mode:
with open("file_name", "w") as file:
file.write("This is a new line.")
This will write the text “This is a new line.” to the file specified by file_name.
What is the ‘with’ statement used for when opening a file in Python?
The with statement is used for opening a file in Python as it ensures that the file is properly closed after the code block is executed, even if an exception occurs.
This is known as using a context manager.
It is considered a best practice as it simplifies the handling of file closing and helps to prevent file handling issues or resource leaks.
The following is an example:
with open("file_name", "r") as file:
content = file.read()