Python offers various methods for working with lists. But, one common operation when dealing with them is shuffling elements to create a new sequence with random order.
This technique can be useful in numerous scenarios, such as creating random sequences for testing purposes, forming diverse data combinations, or generating random game scenarios.
To shuffle a list in Python, you can use the shuffle() function from the random module to randomly rearrange elements in a list. You can also use the sample() function which returns a new shuffled list without changing the existing one.
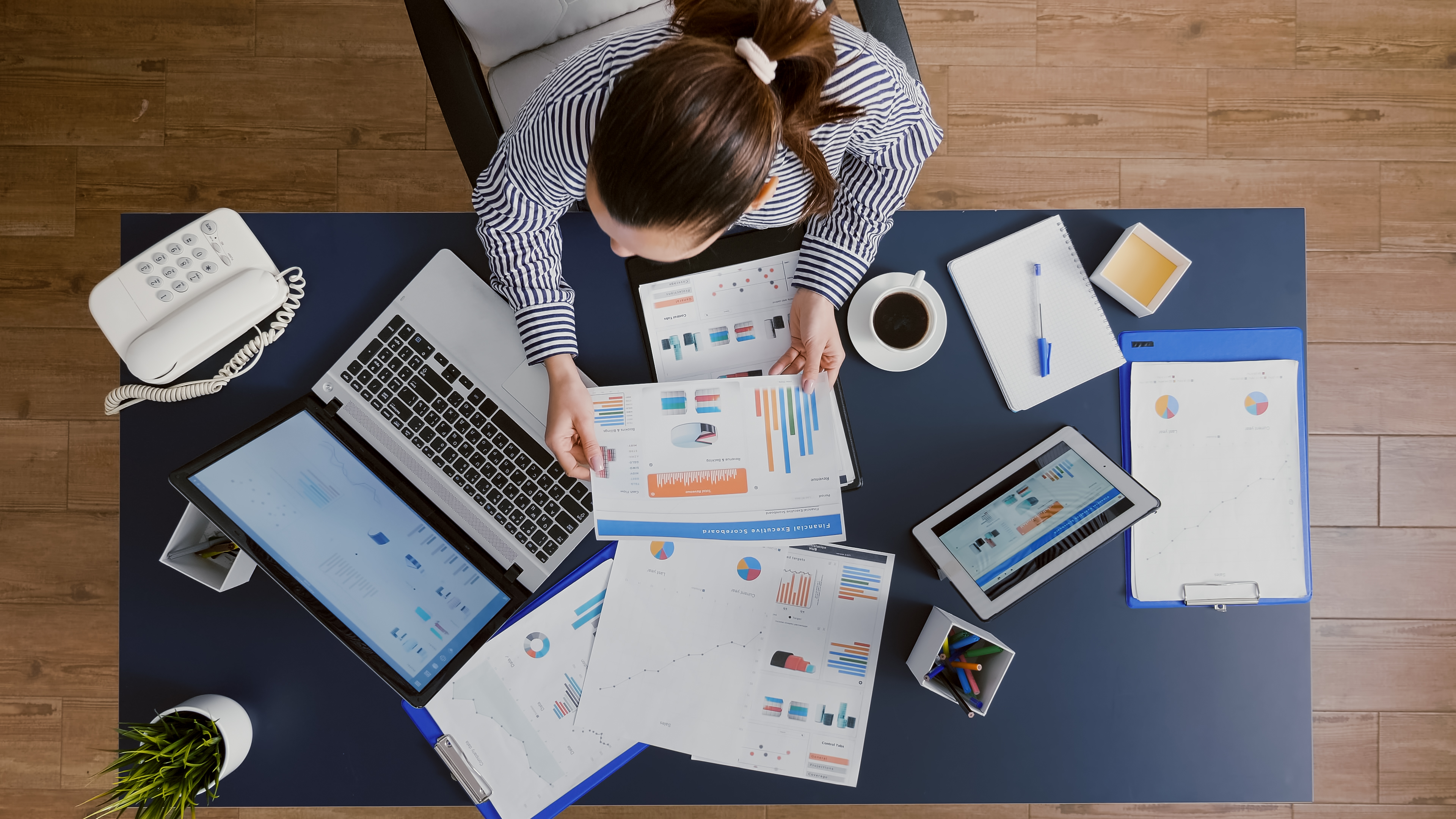
Understanding how to use Python’s random.shuffle() method effectively is important as it can help you create dynamic and unpredictable behaviors within your applications. This article will explore how to use shuffle() and sample() for shuffling a list in Python.
Let’s get into it!
How to Shuffle List in Python
In Python, shuffling a list refers to the process of rearranging its elements in a random order. This can be useful in various applications, such as simulations, games, or data analysis tasks, where the order of elements in a list needs to be randomized.
Python offers more than one method to shuffle a list. The random library provides a convenient shuffle() function to shuffle random list items. This function shuffles a given sequence in place, meaning that the original order of the elements is lost, and a new random order is generated.
The shuffle() function has the following syntax random:
random.shuffle(x)
Where x is the list or sequence you want to random shuffle.
The following is an example of how to use the shuffle() function:
import random
countries = ["China", "USA", "UK", "France"]
random.shuffle(countries)
print(countries)
The output will be a shuffled version of the original list elements, as shown below:
It’s essential to note that the shuffle() function returns None and modifies the original list or array. Therefore, it’s unsuitable for cases where you must maintain the original list order.
To return a new list containing elements from the original list without modifying it, you can use the sample() function from the random library:
import random
countries = ["China", "USA", "UK", "France"]
shuffled_countries = random.sample(countries, len(countries))
print(shuffled_countries)
The second argument of the sample can be a random argument or random number and can be less than or equal to the size of the list.
The output of the sample method will be:
If you’d like your code to be reproducible, you can use the random seed functionality that comes as part of the module random.
What is In-Place Shuffling?
In Python, one of the most efficient ways to shuffle a list is by using in-place shuffling. This means that the original list is modified, instead of creating a new list with shuffled items.
In-place shuffling can be achieved using the random.shuffle() function from Python’s random library.
Here’s an example of how to use random.shuffle() for shuffling random:
import random
my_list = [1, 2, 3, 4, 5]
random.shuffle(my_list)
print(my_list)
In this example, the random.shuffle() function is called with my_list as an argument. The function arranges the items in the list based on a random index, and the print() statement displays the shuffled list.
How to Customize Shuffle Behavior in Python
In Python, you can shuffle a list using the random.shuffle() method from the random module. However, you might find cases where customizing the default shuffle behavior is needed. You can do this by defining a custom randomizer function and passing it to the shuffle() method as an argument.
The custom randomizer function should return a float between 0.0 and 1.0. This numeric value determines the shuffle pattern of the list’s elements.
For example:
import random
def custom_random():
# Define your custom random function logic here
return random.random() * 0.5
my_list = [1, 2, 3, 4, 5]
random.shuffle(my_list, custom_random)
This custom randomizer will create a “light” shuffle, as it generates values only between 0.0 to 0.5, which may suit your needs better in certain cases. You can adjust the behavior by modifying the custom random function.
If you need to shuffle multiple lists with the same order, you can first define the permutation and apply it to all lists
For example:
import random
# Define your lists.
list1 = ['a', 'b', 'c', 'd', 'e']
list2 = [1, 2, 3, 4, 5]
list3 = ['alpha', 'beta', 'gamma', 'delta', 'epsilon']
# Create a permutation of indices.
indices = list(range(len(list1))) # Generate a list of indices.
random.shuffle(indices) # Shuffle the indices.
# Apply the permutation to your lists.
list1 = [list1[i] for i in indices]
list2 = [list2[i] for i in indices]
list3 = [list3[i] for i in indices]
print(list1)
print(list2)
print(list3)
The output will be:
In situations where a certain sample size is needed from a shuffled list, this can be achieved using random.sample()
For example:
import random
sample_size = 3
my_list = [1, 2, 3, 4, 5]
shuffled_sample = random.sample(my_list, sample_size)
random.sample() returns a new list consisting of unique elements from the input list, with a length equal to the specified sample size. This is useful when you want to pick a smaller, randomized subset from a larger list.
The above method generates output similar to the one shown in the screenshot:
To ensure a deep copy of the original list remains intact, use the copy module and its copy() or deepcopy() functions:
import random
import copy
original_list = [1, 2, 3, 4, 5]
copied_list = copy.copy(original_list) # shallow copy
deep_copied_list = copy.deepcopy(original_list) # deep copy, used for nested lists
random.shuffle(copied_list)
By using the copy module, you can work with the shuffled list without affecting the original list’s order.
To learn more about lists and Python techniques, check the following video out:
Final Thoughts
Shuffling a list in Python is a key technique that holds substantial value in fields like data science, where randomized data sets can help enhance the accuracy of models.
Furthermore, understanding how to shuffle lists also means you’re adding another powerful tool to your programming skill set.
By learning this technique, you’re able to create diverse scenarios for testing, generate unexpected inputs for your functions, and even build fun elements like games and lotteries.
Also, the knowledge of how to keep original lists intact while creating shuffled versions, or maintaining correspondence across multiple lists, provides you greater control over your data manipulation tasks.
Frequently Asked Questions
How can I randomly shuffle a list?
To randomly shuffle a list in Python, you can use the random.shuffle() function from the random module. Simply import the module and pass your list as an argument to the function.
For example:
import random
my_list = [1, 2, 3, 4, 5]
random.shuffle(my_list)
print(my_list)
What function is used to shuffle lists?
The random.shuffle() function from the random module is used to shuffle lists in Python. It rearranges the order of elements in the list in a random manner.
How to set a seed for random.shuffle?
To set a seed for the random.shuffle() function, you can use the random.seed() function. This allows you to obtain a consistent random order each time your program runs.
Here’s an example:
import random
random.seed(42) # Any number can be used as the seed
my_list = [1, 2, 3, 4, 5]
random.shuffle(my_list)
print(my_list)
How can I shuffle a string in Python?
Strings are immutable in Python, which means you cannot shuffle them directly. However, you can convert the string to a list of characters, shuffle the list using random.shuffle(), and then join the characters back into a string. Example:
import random
my_string = "hello"
string_list = list(my_string)
random.shuffle(string_list)
shuffled_string = ''.join(string_list)
print(shuffled_string)
What alternatives are there to shuffle a list?
An alternative method to shuffle a list is using the random.sample() function. This creates a new list with the same elements but in a random order:
import random
my_list = [1, 2, 3, 4, 5]
shuffled_list = random.sample(my_list, len(my_list))
print(shuffled_list)
Is it possible to shuffle two lists together?
To shuffle two lists together, you can first combine the lists using the zip() function and then shuffle the combined zipped list.
After shuffling, you can unzip the lists back into two separate lists. Here’s an example:
import random
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
zipped_list = list(zip(list1, list2))
random.shuffle(zipped_list)
list1, list2 = zip(*zipped_list)
print(list(list1))
print(list(list2))