As you explore Python and take on more complex projects, you’ll notice that some projects have lots of files. Managing these files can slow your progress and divert your attention, which is why it’s important to learn how to manipulate file paths and directories using Python functions. One essential function to achieve this is os.path.join().
The os.path.join() function allows you to join one or more path segments to create a complete path. It ensures that exactly one directory separator is placed between each non-empty part, with the exception of the last part. This way, you can avoid hard-coding pathnames manually.
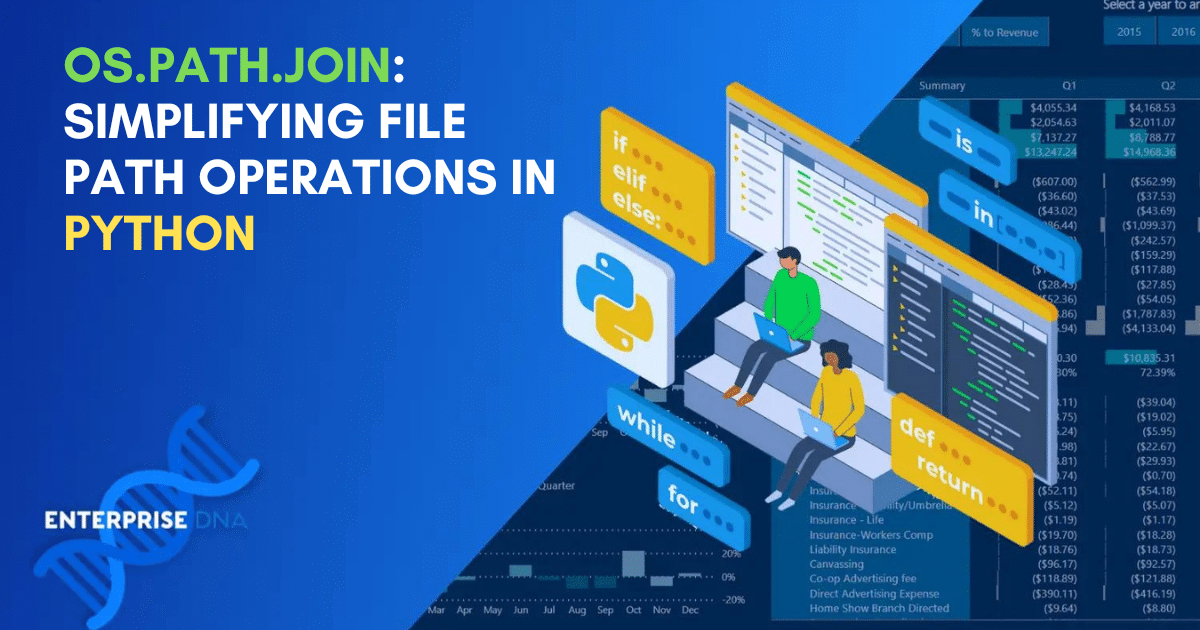
In this article, we’ll explore the use of the os.path module and how you can create platform-independent paths. Let’s start with an overview of the os.path.join!
Overview of os.path.join
os.path.join is a useful method in Python’s os.path module, which allows you to combine one or more path segments to form a complete file system path.
This is helpful when you are working with files and directories because it helps create paths in a platform-independent manner without the need for hard coding.
When you use os.path.join(), it takes path and paths as parameters, where path and the components in paths can be either a string or a bytes object representing a path.
The method concatenates path with all members of *paths, ensuring that only one directory separator appears after each non-empty part, except the last one.
We have listed some important points for you to remember about os.path.join():
It helps you create platform-independent paths that work across different operating systems.
The method automatically takes care of adding the required directory separator (either a forward slash / or a backslash depending on the operating system) between path components.
You can use os.path.join() with other os methods, like os.walk(), when constructing file or folder paths.
The following is an example of how to use os.path.join() to combine files and folders into a single path:
import os
path1 = "folder1"
path2 = "folder2"
filename = "helloworld.txt"
full_path = os.path.join(path1, path2, filename)
print(full_path)
In this example, os.path.join() is used to combine folder1, folder2, and example_file.txt into a single path. The resulting output is shown in the image below:
os.path.join() ensures that you can conveniently work with file and directory paths.
The above example is provided to give an overview of the usefulness of os.path.join(). In the next section, we’ll look at the syntax of os.path.join() to help you better understand the logic behind this function.
What is the Syntax of os.path.join()?
In this section, we’ll cover the syntax and parameters of the os.path.join() method in Python.
The syntax for using os.path.join() is as follows:
os.path.join(path, *paths)
We’ve listed the key parameters for you that you’ll need to understand when using this function:
path: This is the first part of the file or folder’s address. It’s usually a string (a sequence of letters, numbers, or symbols), but it can also be other types of data that act like a string.
*paths: These are any other parts of the file or folder’s address that you want to add to the first part. You can add as many parts as you want. Just like with the first part, these can be strings or other types of data that act like strings.
When using os.path.join(), the method concatenates the provided paths while inserting the appropriate separator (e.g., / or ) based on your system. This ensures that combined paths are compatible with your operating system and other Python functions.
Now that you know the syntax of os.path.join() function, let’s look at the os module which allows you to use this function.
How to Use the os.path.join Function
To start using the os.path.join method in your Python program, you first need to import the necessary os module. os means that it is an operating system-dependent functionality.
To import the os module, simply add the following line at the beginning of your Python script:
import os
Once you have imported the os module, you can make use of various methods, including os.path.join.
This method is particularly useful for combining path components intelligently. It helps to avoid issues with path concatenation and makes your code more portable and readable.
To use os.path.join, you can call it with the desired path components as arguments:
combined_path = os.path.join(path1, path2)
When you incorporate the os module and os.path.join method in your code, you ensure that your file path manipulations are consistent and reliable across different platforms.
It’s also important that you know how to work with paths when using the os.path.join() function in your Python code.
How to Work with Paths
In this section, we’ll look at the different components of path which will enable you to make or manipulate paths according to the problem at hand.
Specifically, we’ll look at the following:
Concatenating Path Components with os.path.join()
Absolute and Relative Paths
1. Concatenating Path Components with os.path.join()
When working with files and directories in Python, you often need to manipulate pathnames with the join method. The os.path.join() function is a helpful tool for this purpose.
The following example shows how you can concatenate paths using the os.path.join method:
import os
path1 = "home"
path2 = "your_directory"
filename = "your_file.txt"
fullpath = os.path.join(path1, path2, filename)
print(fullpath)
In this Python script, we are manipulating file paths by joining together the parts “/home”, “your_directory”, and “your_file.txt” using the os.path.join() function.
The result is a single string that forms a complete concatenated path, which represents the location of the your_file.txt file in the system.
To learn more about manipulation and transformation in Python, check the following video out:
2. Absolute and Relative Paths
When using the os.path.join() function, you can work with both absolute and relative paths.
An absolute path is a complete path that begins with the root directory and includes all pathname components.
The following is an example of working with an absolute path component using the os.path.join() method:
import os
# Absolute path
abs_path1 = "/var/www"
abs_path2 = "html"
filename = "index.html"
full_abs_path = os.path.join(abs_path1, abs_path2, filename)
print(full_abs_path) # "/var/www/html/index.html"
In this script, we’re constructing an absolute path to a file named index.html located in the html directory under /var/www.
We achieve this by using the os.path.join() function to combine “/var/www”, “html”, and “index.html” into a single, full path.
A relative path indicates the location of a file or directory relative to the current directory. The following is an example of working with relative paths in the os.path.join() method:
import os
rel_path1 = ".."
rel_path2 = "other_directory"
filename = "example.txt"
full_rel_path = os.path.join(rel_path1, rel_path2, filename)
print(full_rel_path) # "../other_directory/example.txt"
In this code, we are creating a relative path to a file called example.txt in a directory named other_directory. This directory is one level up from the current directory, represented by “..”.
We are using the os.path.join() function to put these parts together into one complete relative existing path.
Another important component of working with directories is accessing the current working directory. When you are using the os.path.join() function, you’ll be accessing the current working directory most of the time. This is because the current working directory acts as a starting point when constructing new ones.
Let’s look at how you can access the current working directory!
How to Access the Current Working Directory in Python
When working with paths and directories, you might come across situations where you need to navigate through directories or access files from different locations. Understanding the concept of the current working directory (CWD) is crucial for this purpose.
The current working directory is the folder that your Python script is currently executing in.
To get the current working directory, you can use the os.getcwd() function from the os module as shown in the code example below:
import os
current_directory = os.getcwd()
print("Current working directory:", current_directory)
This code gives us the current working directory as shown below:
Are Paths Created with os.path.join Cross-Platform Compatible
Yes, the os.path.join() function ensures that you create file paths in a portable way, regardless of the underlying operating system.
This function takes care of using the correct directory separator for the current platform, making your code more adaptable and maintainable.
For instance, take the following example where we join paths and files using os.path.join():
import os
path = os.path.join('directory1', 'directory2', 'file.txt')
print(path)
At the time of writing, we used a Windows operating system, and the output looked like the following:
The output shows the current user’s home directory.
However, if you’re using a Unix-based operating system, your output will look like the following:
/directory2/file.txt
In the above example, you can see that the directory separators are different depending on the operating system, which illustrates how os.path.join() automatically handles the differences for you.
Now that you are familiar with the essential components of os.path.join() function, let’s look at the examples and use cases of this function.
What Are the Use Cases of os.path.join()?
In this section, we’ll explore some examples of how to use os.path.join() in your Python projects. These examples will help you better understand when to use os.path.join() function in your code.
1. Reading and Writing Files
When you’re working with files, you often need to provide a complete path to the file. This path might need to be constructed dynamically, based on factors such as the user’s operating system, the current working directory, or user input.
os.path.join() helps you build these paths in a reliable and platform-independent way.
The following is an example of reading and writing files with the help of os.path.join():
directory = input("Enter the directory where the file is stored: ")
filename = input("Enter the filename: ")
path = os.path.join(directory, filename)
with open(path, 'r') as file:
print(file.read())
In this script, we’re asking the user to input the directory and the filename. Then we use os.path.join() to form a full path to the file. Afterward, we’re opening this file in read mode and printing out its contents.
2. Creating New Directories
When you’re creating new directories using os.makedirs(), you can use os.path.join() to create the path to the new directory.
The following is an example of making new directories with os.path.join():
base_directory = "/tmp"
new_directory = "my_new_directory"
path = os.path.join(base_directory, new_directory)
os.makedirs(path, exist_ok=True)
In this script, we are creating a path to a new directory named my_new_directory under the /tmp directory. We then use os.makedirs() to create this new directory on our system, if it doesn’t already exist.
3. Listing Files in a Directory
If you want to list all files of a certain type in a directory, you can use os.path.join() to create the paths to each file.
The following example demonstrates creating paths to all files using os.path.join():
directory = "E:ospathjoin"
for filename in os.listdir(directory):
if filename.endswith(".txt"):
path = os.path.join(directory, filename)
print(path)
In this script, we’re scanning through all the files in the specified directory, E:ospathjoin, using a for loop.
For each file that ends with “.txt”, we combine the directory and the filename to form a full path, which we then print.
4. Iterating Over Paths with a For Loop
You can also utilize a for loop to iterate over two or more paths components and join them using os.path.join. This can be particularly useful when working with long lists or multiple nested directories. For example:
import os
paths = ["folder1", "folder2", "folder3", "file.txt"]
combined_path = ""
for p in paths:
combined_path = os.path.join(combined_path, p)
print(combined_path)
In this script, we’re creating a path to a file named file.txt located within a series of nested directories (folder1, folder2, folder3). We do this by sequentially joining each part of the path using os.path.join(), and the method returns a path that is printed out.
Final Thoughts
os.path.join() is an essential part of Python programming language when dealing with file and directory paths. The ability to manipulate paths in a manner that’s compatible with all operating systems is an important skill to have. It ensures that your code remains less prone to errors.
Moreover, understanding and using os.path.join() will help you interact with the file system — reading from files, writing to files, creating directories, and more.
As you continue your journey in Python programming, you’ll find os.path.join() useful when working with directories. The more you use it, the more you’ll appreciate its value. By learning and mastering this function, you’re taking a significant step forward in becoming a more proficient Python programmer!