Python programming language offers a unique set of tools that makes coding not only fun but also accelerate your progress as a programmer. Due to its ease of use, Python has become the go-to language for a variety of industries, from web development and data science to finance and beyond.
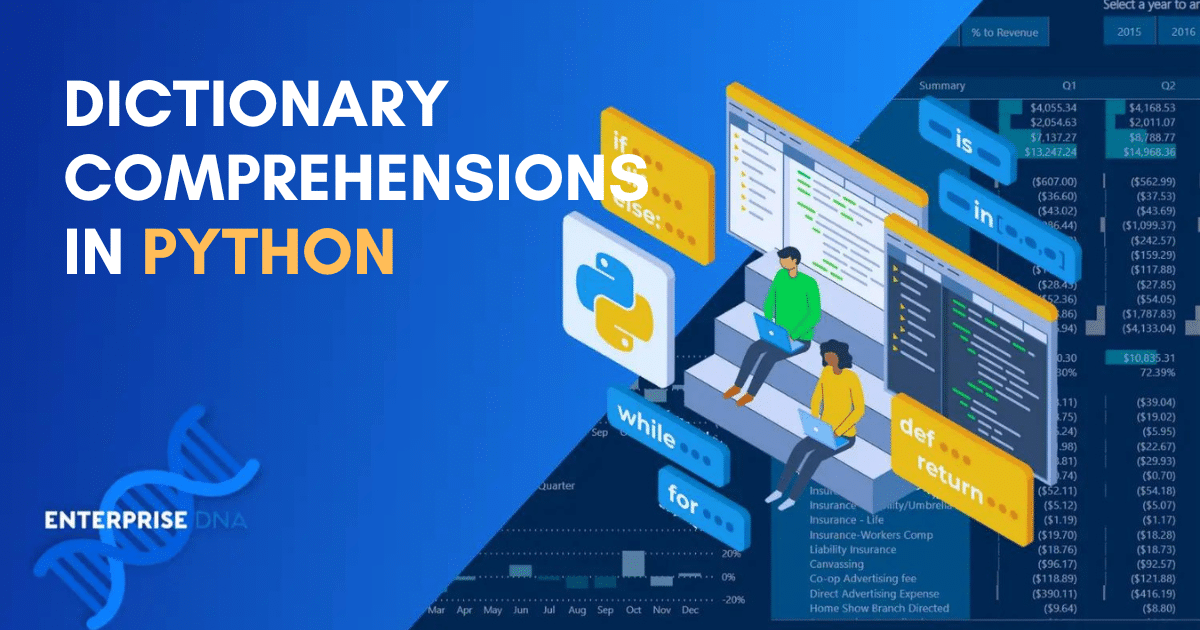
Python dictionary comprehension is an important tool that allows you to generate dictionaries dynamically by iterating over existing iterable data structures or dictionaries to create new ones. You can think of dictionary comprehension as similar to list comprehension but with a slightly different use case.
As you become familiar with comprehensions, you’ll discover they can significantly improve code by promoting clarity and eliminating the need for long, repetitive loops.
If you’re new to Python, you might not have encountered comprehension or used it in your code. Before diving into the syntax, let’s address the primary question: What is Python dictionary comprehension?
What is Python Dictionary Comprehension?
Python Dictionary Comprehension is a concise way to create dictionaries using simple expressions. It allows you to generate new dictionaries without needing to use for-loops.e
Let’s take a look at an example of comprehension. Suppose you have two lists, one containing keys and the other containing values:
keys = ['a', 'b', 'c']
values = [1, 2, 3]
Using dictionary comprehension, you can create a new dictionary by iterating over the two lists and combining the corresponding key-value pairs:
my_dict = {k: v for k, v in zip(keys, values)}
print(my_dict)
The output will be:
{'a': 1, 'b': 2, 'c': 3}
The above example should give an idea of what comprehensions are in Python. To further help you solidify the concept, let’s understand the syntax of dictionary comprehensions in Python.
Understanding the Syntax of Dictionary Comprehension
The syntax for dictionary comprehension is simple but powerful. They offer a concise way to create a new dictionary in Python. Also, they follow a similar syntax to list comprehension but use curly braces {} and a key-value pair separated by a colon.
The basic structure is as follows:
{key: value for (key, value) in iterable}
In this syntax, the key and value represent the expressions used to generate the keys and values of the resulting dictionary. The iterable provides the source of data used to create the key-value pairs.
This syntax was introduced in Python 3 and backported as far as Python 2.7, ensuring wide compatibility across Python versions.
For example, to create a dictionary that maps numbers from 0 to 4 to their squares using dictionary comprehension, you can use the following Python code:
square_dict = {x: x**2 for x in range(5)}
This will result in the following dictionary:
{0: 0, 1: 1, 2: 4, 3: 9, 4: 16}
What if you’d like to iterate over two variables at the same time? You can achieve this using dictionary comprehension with the zip() function to create a dictionary from two iterables, one representing the keys and the other representing the values. Here’s an example:
keys = ['a', 'b', 'c', 'd', 'e']
values = [1, 2, 3, 4, 5]
my_dict = {k: v for k, v in zip(keys, values)}
The resulting dictionary will be:
{'a': 1, 'b': 2, 'c': 3, 'd': 4, 'e': 5}
Dictionary comprehension also allows you to add an optional if statement at the end of the comprehension to apply a conditional filter on the included items.
For example, you can create a dictionary with only even numbers and their squares using dictionary comprehension:
even_square_dict = {x: x**2 for x in range(10) if x % 2 == 0}
This results in:
{0: 0, 2: 4, 4: 16, 6: 36, 8: 64}
Now that you have some familiarity with the syntax of comprehension let’s take a look at some examples of comprehension.
Examples of Dictionary Comprehension
Dictionary comprehension finds a range of use cases due to its powerful and elegant syntax. They can save you time and make your code more readable.
Mapping Numbers to their squares
Consider a simple example where you want to create a dictionary that maps numbers to their squares using Python dictionary comprehensions:
squares = {x: x**2 for x in range(1, 6)}
print(squares)
This will output:
{1: 1, 2: 4, 3: 9, 4: 16, 5: 25}
The above code does the same job as running a loop six times and squaring each number. The code below demonstrates running a loop to take the squares of numbers:
squares = {}
for x in range(1, 6):
squares[x] = x ** 2
print(squares)
At this point, you might be wondering why to use comprehension when you can use loops. In programming, a rule of thumb is to write compact and efficient code.
You can also apply conditions to your comprehension. For instance, you may want to create a dictionary that contains only even numbers and their squares:
even_squares = {x: x**2 for x in range(1, 6) if x % 2 == 0}
print(even_squares)
Running this code will produce a new dictionary like the following:
{2: 4, 4: 16}
Reverse Keys and Values
You can also reverse dictionary elements with ease with dictionary comprehension in Python.
The following code demonstrates how to reverse keys and values of an existing dictionary using dictionary comprehension:
original_dict = {"one": 1, "two": 2, "three": 3, "four": 4}
reversed_dict = {v: k for k, v in original_dict.items()}
print(reversed_dict)
The above dictionary comprehension(or dict comprehension) will print the following:
{1: 'one', 2: 'two', 3: 'three', 4: 'four'}
To get a good understanding of what dictionaries are and how you can use them for different cases, please refer to the video given below:
The examples provided serve as a solid foundation for employing dictionary comprehension in your Python projects.
Also, dictionary comprehension can be further optimized, allowing a single line of code to accomplish complex tasks that might consume more time and memory with loops.
3 Major Advantages of Dictionary Comprehension
You’ve experienced the elegance of dictionary comprehension through the examples provided. Apart from readability, comprehensions offer several advantages, including efficiency, when working with Python’s efficient key/value hash table structure, called dict comprehension.
1. Creating Clear and Simple Code
Comprehensions allow you to create new dictionaries effortlessly and in a readable manner. They make your code look and feel more Pythonic compared to traditional loops.
2. Better Performance with Style
Comprehensions are not only elegant but also efficient. They often work faster than for loops since they use a single expression. Python optimizes this kind of code, eliminating the need for repeatedly calling the dictionary constructor or using the update() method.
3. Easy Filtering and Data Transformation
Another advantage of dictionary comprehension is its simplicity in filtering and transforming data. For instance, you can create a new dictionary from an existing one by applying a condition or modifying the keys or values.
It’s crucial to understand when to use dictionary comprehension in Python, as they can quickly become complex and harder to read and write. Now, let’s explore some use cases for dictionary comprehension in Python.
3 Examples of When to Use Dictionary Comprehension
Comprehensions are useful in the following 3 scenarios:
1. When Creating a dictionary from two lists
{key:value for key, value in zip(list1, list2)}
2. When Filtering a dictionary based on value
{k: v for k, v in original_dict.items() if v > threshold}
3. When Transforming keys or values
{k.upper(): v * 2 for k, v in original_dict.items()}
It’s important to remember that while comprehensions can make your code more elegant and efficient, they can also become difficult to read if they are overly complex.
Let’s look at some of the most common pitfalls when using dictionary comprehensions.
Pitfalls of Dictionary Comprehension
Dictionary comprehensions bring elegance and efficiency but also comes with challenges. We’ll discuss common pitfalls related to readability, complexity, and performance with large data sets.
Balancing Complexity and Readability
Complex dictionary comprehensions can be difficult to read. Prioritize code readability, especially when working with others. In some cases, traditional for loops might be simpler and more maintainable.
Performance with Large Data Sets
For large data sets, dictionary comprehension may not always be optimal. According to Stack Overflow, a built-in dict() constructor can outperform dictionary comprehensions with numerous key-value pairs due to looping in C.
Comparison with Other Methods
In this section, we’ll compare dictionary comprehensions with two alternative methods of creating dictionaries in Python: for loops and lambda functions.
For Loop
Using a for loop to create a dictionary involves initializing an empty dictionary and then iterating through the desired valid key value pair, adding each pair to the dictionary. This is a more verbose method compared to dictionary comprehension.
THe following is an example of creating dictionary using for loops:
dictionary = {}
for key, value in zip(keys, values):
dictionary[key] = value
Conversely, a dictionary comprehension allows you to achieve the same result in a single line:
dictionary = {key: value for key, value in zip(keys, values)}
As you can see, comprehensions provide a concise and more readable way of creating dictionaries in Python.
Lambda Functions
We can also use Lambda functions to create dictionaries. Lambda functions are a way to create small, anonymous functions in Python. They can be used in conjunction with the map() function to create a dictionary.
Let’s look at an example of Lambda functions to create dictionaries:
keys = ['a', 'b', 'c']
values = [1, 2, 3]
key_value_pairs = map(lambda key, value: (key, value), keys, values)
dictionary = dict(key_value_pairs)
By comparison, here’s how the same dictionary can be created using comprehension:
dictionary = {key: value for key, value in zip(keys, values)}
Again, dictionary comprehension provides a concise and more readable alternative to creating dictionaries using lambda functions and the map() function.
Best Practices for Dictionary Comprehension
When using comprehensions in Python, it’s essential to follow best practices to improve readability, maintainability, and efficiency. Here are some best practices to consider:
1. Keep dictionary comprehension simple
Dictionary comprehension is powerful, but it should be clear and concise. If your comprehension gets too complex or spans multiple lines, consider using a traditional for loop instead. This aids code understanding, especially for others or when revisiting your work later.
2. Leverage built-in functions
Python offers built-in functions like zip() and enumerate() that help create comprehensions. Use these functions to streamline your code and make it more readable.
For example, using zip() to combine two lists and create a dictionary results in concise and clean code like this:
{key: value for key, value in zip(keys, values)}
3. Use conditional statements wisely
Incorporate conditional statements in your comprehensions when necessary, but don’t overuse them. They can help filter or modify the resulting dictionary, but too many nested conditions can make the code hard to read. A simple example involving a conditional statement is:
{x: x**2 for x in range(10) if x % 2 == 0}
4. Be mindful of nested dictionary comprehension
Nested dictionary comprehension can generate dictionaries with nested structures. However, like conditional statements, an excess of nested dictionary can make the code harder to decipher. Avoid going beyond two levels of nesting in your nested dictionary comprehension.
Sometimes you might have to use nested dictionary comprehension inside loops to achieve the desired efficiency.
Our Final Say
By now, you’ve mastered dictionary comprehension in Python. This elegant, efficient technique helps create dictionaries by iterating over iterables or using conditions. Comprehensions streamline your code, boosting readability and maintainability.
Creating new dictionaries is easy with comprehension, saving time and effort compared to traditional for-loops. They’re useful in real-world applications like data processing, mapping, and transformation.
If you’d like to further your Python knowledge and become a real pro, sign up for free for our Python course and see how we are creating the world’s best Python users.