Python comes loaded with a range of built-in functions. A frequent operation when working with data is to find summary statistics, which include metrics like finding maximum, minimum, mean, and median values. This article will specifically focus on finding the minimum value of a dataset or input arguments using the Python min() function.
The Python min() function allows you to find the smallest value among a group of values or in an iterable data structure. It can be applied to different types of iterables, such as lists, tuples, sets, and dictionaries. To use the Python min() function, you can simply pass an iterable to min(), such as min([2, 3, 1]), which would return 1.
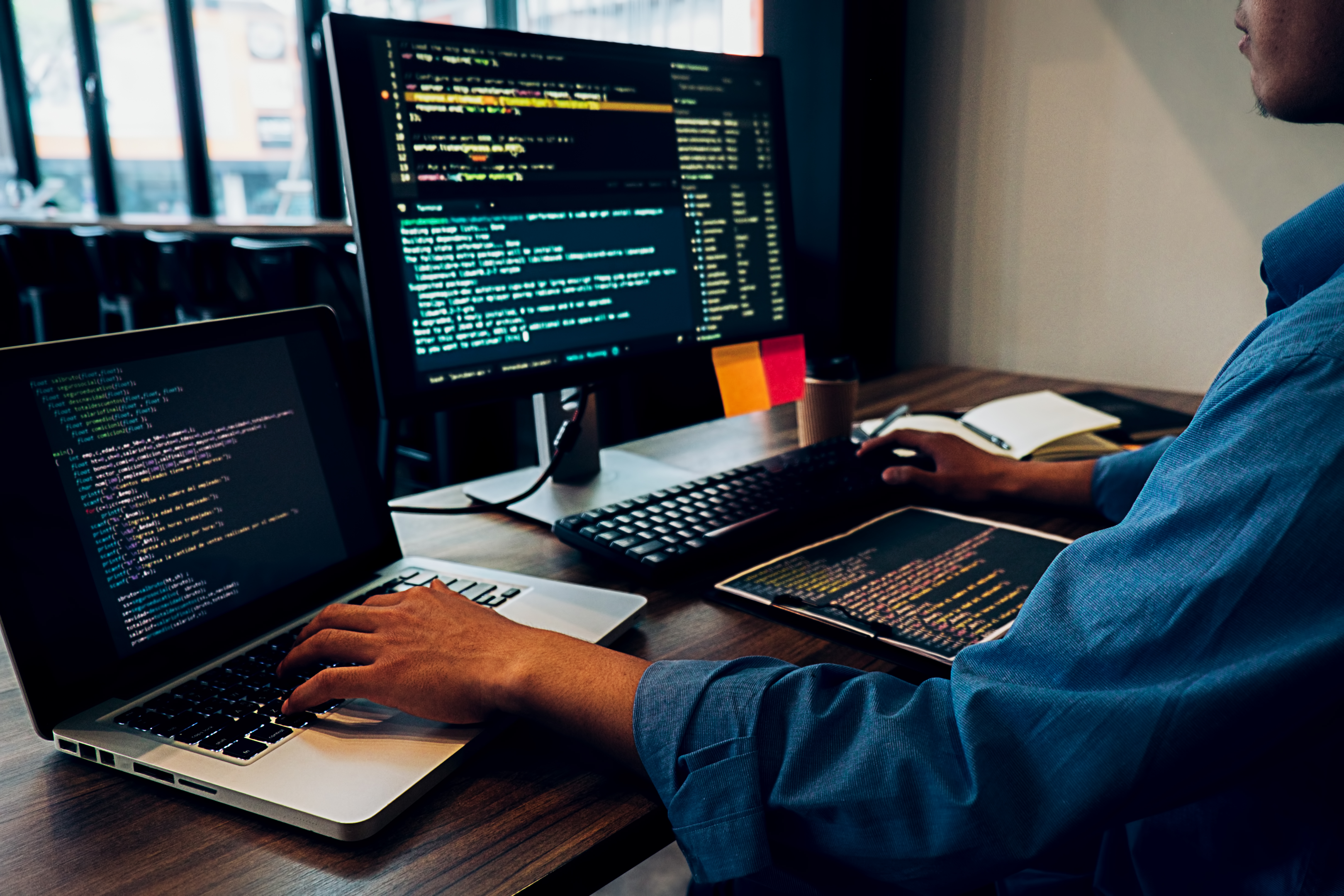
In this article, we’ll explore how to use Python’s min() function to find the minimum value in a group of values or an iterable. We’ll review multiple examples and use cases to help you better understand the concepts.
Let’s get into it!
What is the Syntax of Python min() Function?
The min() function has two main forms of syntax:
- Syntax for Arguments
- Syntax for Iterables
1) What is the Syntax for Arguments?
The first form finds the smallest item between two or more arguments. When you use min() without the iterable, the return value is the largest object among the parameters passed.
The following is the syntax for arguments:
min(arg1, arg2, *args[, key])
Here, arg1, arg2, *args are arguments that can be any two or more numbers (integers, floats) or strings. The optional key parameter is a function to serve as a key or basis of sort comparison.
2) What is the Syntax for Iterables?
The second form can be used to find the largest item in an iterable, such as a list, set, or dictionary.
The syntax looks like the following:
min(iterable, *[, key, default])
Here, iterable can be a sequence (string, tuples, etc.) or collection (set, dictionary, etc.) or an iterator object to be examined.
The optional key parameter is a function to serve as a key or basis of sort comparison. The default value is returned if the provided iterable is empty.
5 Examples of Python min() Function
Now that you are familiar with the syntax of the min() function, let’s further solidify our understanding of the function by going over some examples.
In this section, we’ll go over 5 examples of the min() function. These examples will help you better understand the use of this function.
Example 1: Finding the Smallest Number Among Multiple Arguments
You can use the min() function with multiple individual numbers as arguments to find the minimum value.
The following example explains this use case:
smallest_number = min(45, 23, 78, 31)
print(smallest_number)
min() will return 23, the smallest number among the provided default argument.
Example 2: Finding the Smallest Number in a List
You can also use the Python min() function to find the smallest number in a list.
The following code demonstrates this method:
numbers = [12, 25, 33, 5, 77] # list of numeric values
smallest_number = min(numbers)
print(smallest_number)
This will print the smallest number in the list numbers, which is 5.
Example 3: Finding the Shortest String in a List
You can also use the min() function on a list of strings. The default parameter will return the string which would come first in lexicographic order.
However, you can change its behavior by providing a key function.
Let’s say you want to find the shortest string in a list of strings. The following code accomplishes this task:
words = ["cat", "window", "defenestrate"]
shortest_word = min(words, key=len)
print(shortest_word)
Here, min() uses the length of the words as the comparison key, and it returns “cat”, the shortest word in the list.
Example 4: Finding the Smallest Number in an Array of Complex Numbers Based on a Condition
You can use the min() function with a key function to handle more complex scenarios.
For instance, if you have a list of complex numbers and you want to find the one with the smallest imaginary part, you can use the following code:
complex_numbers = [3 + 2j, 1 + 1j, 2 + 4j, 5 + 1j]
smallest_imaginary = min(complex_numbers, key=lambda x: x.imag)
print(smallest_imaginary)
Here, the min() function uses a lambda function to treat the imaginary part of the numbers as the comparison key.
The output will be:
Example 5: Finding the Dictionary With the Smallest Value for a Particular Key
You can use min() with a list of dictionaries if you provide a function that can compare dictionaries.
Here’s how you can find the dictionary that has the smallest value for a particular key:
people = [
{"name": "Alice", "age": 25},
{"name": "Bob", "age": 20},
{"name": "Charlie", "age": 30}]
youngest_person = min(people, key=lambda x: x['age'])
print(youngest_person)
Here, min() uses a lambda function to treat the age field of the dictionaries as the comparison key.
The output will be:
How to Use the Key Argument in min() Function
The min() function in Python can accept a key argument, which should be a function that takes a single argument and returns a key to use for sorting purposes.
The key function is applied to each item on the iterable to determine the item with the minimum value.
Below, you’ll find 2 examples of customizing the key argument for different scenarios.
Example 1: Using a Custom Function as the Key Argument
You can define your own function to use for the key argument.
For example, if you have a list of dictionaries and want to find the dictionary with the smallest value for a specific key, you could do something like this:
data = [
{'name': 'Alice', 'age': 25},
{'name': 'Bob', 'age': 20},
{'name': 'Charlie', 'age': 30}]
def get_age(person):
return person['age']
youngest_person = min(data, key=get_age)
print(youngest_person)
In this code, get_age is a function that takes a dictionary and returns the value of the ‘age’ key. This function is then used as the key argument in the min() function. The output will be:
Example 2: Using abs() Function as the Key Argument
If you want to find the smallest number based on the absolute value, you can use the abs() function as the key.
The following code explains this method:
numbers = [-10, -5, 0, 5, 10]
smallest_number = min(numbers, key=abs)
print(smallest_number)
In this example, min() uses the absolute value of the numbers for comparison, so it returns the number with the smallest absolute value.
How to Use min() Function in NumPy And Pandas
The Python libraries NumPy and Pandas both have their own min() functions which are more suited to the multi-dimensional arrays and DataFrames used by these libraries.
In this section, we’ll go over the following:
- Using the min() function in numpy
- Using the min() function in pandas
1) How to Use the min() Function in NumPy
You can use the numpy.min() function to return the minimum of an array or minimum along an axis.
If you have a one-dimensional NumPy array, you can use the following code to find the minimum value:
import numpy as np
arr = np.array([2, 5, 1, 7, 3])
print(np.min(arr))
The output will be:
If you have a multi-dimensional array, you can find the minimum value along an axis using the following code:
arr_2d = np.array([
[2, 5, 1],
[7, 3, 6]])
min_val = np.min(arr_2d, axis=1)
print(min_val)
In the above example, np.min(arr_2d, axis=1) returns the minimum value along the row axis for each row.
The minimum value for row 1 is 1, and for row 2 is 3.
2) How to Use the min() Function in Pandas
You can use the DataFrame.min() function to return the minimum of the values for the requested axis in pandas DataFrame.
If you want to find the minimum value for each column in a pandas dataframe, you can use the following code:
import pandas as pd
data = {'A': [1, 2, 3],
'B': [4, 5, 6],
'C': [7, 8, 9]}
df = pd.DataFrame(data)
print(df.min())
In the above example, df.min() returns the minimum value of each column.
Learn more about data transformation and visualization by watching the following video:
Final Thoughts
The min() function is a key tool for finding the smallest values in your data, whether it be numbers, strings, or even more complex data structures. By learning how to use it, you’ll gain the ability to write more efficient code.
This Python function has a straightforward syntax but carries a complex logic. Its optional key parameter opens up a range of possibilities for customized comparisons, offering you the flexibility to solve various problems.
Moreover, when you are working with large datasets or complex numerical computations, the min() function proves helpful with its compatibility with libraries like NumPy and Pandas. This means that whatever your data looks like, min() can help you extract valuable insights with ease.
Frequently Asked Questions
How do I use the key parameter in min function?
The key parameter in the min function allows you to define a function to customize the sorting criteria. T
he function should take a single argument and return a value that will be used for comparison.
For instance, let’s say you want to find the minimum string length in a list of strings, you can use the len function as the key:
strings = ["apple", "orange", "banana"]
min_length = min(strings, key=len)
How to get the index of the minimum value in a list?
To get the index of the minimum value in a list, you can use the min function with the enumerate function and the key parameter.
The following is an example:
numbers = [3, 5, 2, 1, 5]
min_index = min(enumerate(numbers), key=lambda x: x[1])[0]
Can I use lambda with min function in Python?
Yes, you can use lambda with the min function in Python.
The lambda function can be used as the key parameter.
For example:
elements = [{"value": 1}, {"value": 2}, {"value": 3}]
min_element = min(elements, key=lambda x: x["value"])
How to find minimum value in a list without built-in function?
To find the minimum value in a list without using the built-in min function, you can use a simple loop to iterate over the elements and keep track of the smallest value found so far.
Here’s an example:
numbers = [3, 5, 2, 1, 5]
min_value = numbers[0]
for number in numbers:
if number < min_value:
min_value = number
What is the time complexity of the min function in Python?
The time complexity of the min function in Python is O(n), where n is the number of elements in the input iterable.
This is because the min function performs a single pass through the input iterable to find the minimum value.
What is the difference between max() and min()?
Python’s max and min functions serve the same purpose but in opposite directions.
The max function returns the largest item in an iterable or the largest value among two or more arguments, while the min function returns the smallest item in an iterable or the smallest value among two or more arguments.
Both max and min can take an optional key parameter to customize the sorting criteria.