If you’ve ever wished for a way to merge multiple lists, tuples, files, or dictionaries in Python into a single entity, then you’re in luck! Employ the Python zip() function — your ultimate ally in combining and iterating over multiple iterables.
The Python zip() function is a built-in function that allows you to combine two or more iterables, such as lists, tuples, or dictionaries, into a single iterable. It operates by pairing up elements from each input iterable based on their positions and creating tuples containing the complementary elements.
In this article, we’ll dive into the ins and outs of the Python zip() function, exploring its syntax, common operations, and advanced functionality.
You’ll learn how to combine, compare, and manipulate data from different sources, create dictionaries, traverse files, and even handle potential errors.
So, get ready to unravel the features of Python’s zip() as we guide you through mastering this essential tool in your Python coding!
Understanding Python Zip() Function
In Python, zip() is a built-in function that allows you to combine two or more iterables, such as lists, tuples, or dictionaries, into a single iterable. It enables parallel iteration over multiple iterables, which can lead to improvements in both code readability and efficiency.
The zip() function can be applied to various problems, such as creating dictionaries or pairing elements from multiple input sources. As an iterator, it ensures that memory usage remains low, even when working with large datasets.
Mastering the zip() function will enhance your Python coding skill, as it simplifies complex tasks and leads to cleaner, more maintainable code. With a firm understanding of how to use this function, you’ll be well on your way to becoming a Python maestro!
Now that you know what the Python zip() function is, let’s explore its syntax and usage.
Python Zip Function: Syntax and Usage
To use the zip() function, simply pass the iterables as arguments with separated commas within the zip() function. It then pairs up elements from each iterable based on their positions and creates a zip object containing the complementary elements.
This functionality enables efficient parallel iteration, allowing you to combine data, compare elements, or execute simultaneous operations on complementary elements from different sources.
The general syntax zip() uses is:
Next, let’s go over some common operations with Python Zip() function with a few examples.
1. Combining Iterables Using Zip() Function
In Python, iterables are data structures that can be stepped through one element at a time. They can be either built-in iterables like lists, tuples, dictionaries, and sets or user-defined iterables created by defining a class and implementing the special method `__iter__()`
To combine iterables using zip(), pass your iterables as parameter values separated by a comma. For example, if you want to combine twolists, you would write zip(list1, list2).
After passing arguments, the resulting iterator can be used in a for loop or converted into a list directly using list(zip(list1, list2)).
Example 1: Using a for loop
Output:
In the above code, zip() combines two iterables (names and ages) into a zip object and returns an iterator of tuples where each tuple contains corresponding elements from the input iterables.
The for loop then iterates over each tuple, unpacking it into variables (name and age), and prints the formatted string for each pair of values.
Example 2: Converting into a list
Output:
Here, the zip() function combines the two iterables (names and ages) and returns an iterator of tuples where each tuple contains corresponding elements from the input iterables. The list() function then converts the returned iterator into a list.
i. Handling Multiple Iterables
The zip() function is not restricted to pairing only two iterables; it can handle more than two iterables as well. If three iterables are passed, the zip() function creates tuples by matching elements from all three iterables.
This feature of zip() expands its usefulness in scenarios where synchronization or correlation between multiple sequences is required, enhancing its functionality beyond a simple pairwise combination tool.
Example:
Output:
The zip() function in the above code paired elements from three iterables (names, ages, and scores), creating tuples that combined values based on their respective positions. The function returns a list of tuples representing the combination of name, age, and score for each corresponding element.
ii. Iterables of Unequal Length
If the input iterables have different lengths, the resulting zipped iterator will be determined by the shortest input iterable. This means that the zipped iterator will only contain tuples up to the length of the shortest iterable.
Example:
Output:
Here, the resulting iterator stops when it reaches the end of the shortest input iterable. Since ages have fewer elements than names and scores, the resulting list of tuples, result_zip, only contains pairs of elements until the shortest iterable is exhausted.
To handle unequal lengths and fill up the missing elements, you can use the zip_longest() function from the itertools module. The fillvalue parameter specifies the value to use for filling up an empty list with the missing elements.
In this case, the resulting list will have three tuples, and the missing element in ages will be filled with the specified fillvalue: (“N/A”)
Example: Using zip_longest() to fill up missing elements
Output:
Watch this video to learn more about how to handle missing elements in Python:
2. Unzipping or Unpacking
In Python, zip() is commonly used to combine multiple iterables into a single iterable of tuples. However, you can also perform the reverse operation, called “unzipping,” to extract separate iterables from a list of tuples.
To unzip the list of tuples obtained from zip(), you can use the unpacking operator ( * ) in conjunction with the zip() function. The unpacking operator ( * ) unpacks the list of tuples, distributing each tuple’s elements into separate variables.
The following example illustrates the process:
Output:
In the above code, zipped contains a list of tuples obtained by zipping names and ages. When unzipping using zip(*zipped), the unpacking operator ( * ) unpacks the tuples, and the names and ages elements are printed out separately.
3. Dictionary Creation with Zip() Function
Another common use case of the zip() function in Python is to create a dictionary by combining two lists. The zip() function takes multiple iterable objects as arguments and returns an iterator that generates tuples containing elements from each of all the iterables used.
To create a dictionary using zip(), you can pass two lists as arguments to zip() and then convert the resulting iterator of tuples into a dictionary using the dict() constructor.
Here’s an example:
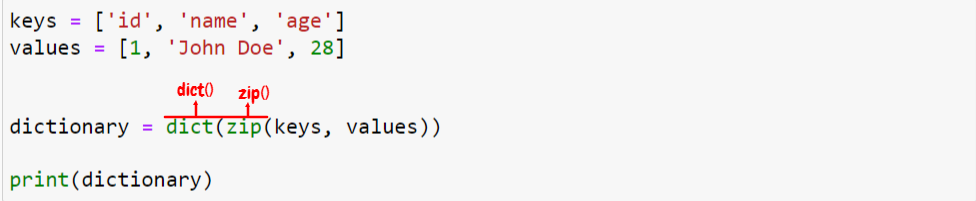
Output:
In the above example, calling zip() function combines the elements from the keys and values lists, creating an iterator of tuples.
The dict() constructor then converts this iterator into a dictionary, where the elements from the keys list become the keys, and the elements from the values list become the corresponding values in the dictionary.
4. Traversing with Enumerate() Function
In Python, the enumerate() function is often used in combination with zip() to simultaneously iterate over two or more sequences while keeping track of the current index. This can be particularly useful when you need to associate an index with the corresponding elements from multiple lists.
Here’s an example that demonstrates the process:
Output:
In this example, zip() combines the elements from the names and ages lists, creating an iterator of tuples. The enumerate() function is then used to iterate over this resulting iterator, providing both the index and the corresponding tuple of (name, age).
Within the loop, you can access and process the elements using name and age variables, along with the associated index.
5. Working with Tuples
The zip() function also generates an iterator that produces tuples as its output. Each tuple contains the corresponding elements from the input iterables. This makes zip() a convenient tool for working with tuples in Python.
To iterate over the resulting tuples obtained from zip(), you can utilize a simple for loop. Alternatively, you can use tuple unpacking, which allows for more concise and readable code.
Here’s an example:
i. Using Simple ‘for’ Loop
Output:
In the above code, zip() takes and combines elements from two iterables: “names” and “ages“, and the function returns an iterator that produces separate tuples. Each tuple contains elements from the input iterables, resulting in a sequence of paired values.
ii. Using Tuple Unpacking
Output:
Here, zip() combines the elements from the “names” and “ages” tuples, creating an iterator of tuples.
During each iteration of the for loop, tuple unpacking is used to assign the elements of each tuple to the variables “name” and “age“. This allows for convenient access to individual elements and enables printing them in the desired format.
Now that you’re familiar with the basic functionality of the zip() function in Python, let’s take a step further and explore its advanced functionality. By harnessing the power of zip(), you’ll unlock a whole new level of data manipulation and iteration techniques.
So get ready to explore advanced zip functionality in Python. Let’s go!
Advanced Zip() Functionality
As we delve deeper into the intricacies of zip() in Python, we’ll explore how it can be used to iterate over multiple iterables in parallel, particularly in scenarios involving files, paths, directories, and archives.
Let’s look at how we can process the contents of multiple files in parallel using zip() along with the open() function.
1. Processing Contents of Multiple Files Using zip() Function
Suppose you have two files, “file1.txt” and “file2.txt,” and you want to read their contents and process them simultaneously. You can achieve this by using the following code
In this code snippet, we use the open() function to open both files simultaneously. By enclosing the two open() statements within the with the statement, we ensure that the files are properly closed after we finish working with them.
The zip(file1, file2) expression combines the lines from file1 and file2 into pairs, which are then unpacked into the variables line1 and line2 in each iteration of the loop. This allows us to process the lines from both files together.
It’s important to note that when working with files, you should handle exceptions properly to account for potential errors such as missing files or incorrect paths.
2. Zipfile Module
Moving on, let’s explore the zipfile module, which provides an interface for working with ZIP archives. With this module, you can extract the contents of a ZIP archive or create new ones.
To extract all files from a ZIP archive, you can use the following code:
import zipfile
with zipfile.ZipFile("example.zip", "r") as zip_ref:
zip_ref.extractall("destination_folder")
In this example, we import the zipfile module, which provides the necessary functions and classes for working with ZIP archives.
By opening the ZIP archive using zipfile.ZipFile(“example.zip”, “r”), we create a ZipFile object named zip_ref. The “r” argument indicates that we want to read the contents of the archive.
Using the extractall(“destination_folder”) method of the ZipFile object, we extract all the files from the archive into the specified “destination_folder”. You can choose any desired folder name.
Remember to consult the official documentation for the zipfile module to explore additional functionalities and options that can be useful for your specific needs.
That’s it on advanced zip() functionality. You’ve learned how to efficiently process the contents of multiple files using zip() and open(), as well as how to work with ZIP archives using the zipfile module. It is important to keep practicing and exploring these techniques as it will enhance your Python programming skills.
Now let’s move over to error handling.
Error Handling and Exceptions with the Zip() Function
When working with the zip() function in Python, it is essential to be aware of the potential errors that can occur.
In this section, we’ll explore the different types of errors that can occur when working with zip() and discuss how to handle them effectively.
1. TypeError
The TypeError can occur when the input to zip() is not iterable or when all the iterables before being zipped are of different types that cannot be combined.
For example:
string = "Hello"
integer = 123
zipped = zip(string, integer) # Raises TypeError
In this case, the zip() function will raise a TypeError since the second argument (integer) is not iterable. You can handle TypeError by changing the input to the correct type or by using the try-except method.
Here’s how to handle a TypeError using the try-except method:
2. RuntimeError
The RuntimeError can occur when the zip() function encounters an unexpected runtime condition that prevents it from generating tuples. This error is usually raised due to external factors that affect the iterator.
For instance:
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
zipped = zip(list1, list2)
for i in zipped:
print(i)
if i == (2, 'b'):
raise RuntimeError("Encountered a runtime error!")
In this example, a RuntimeError is raised when the tuple (2, ‘b’) is encountered. This demonstrates how a runtime condition outside of the zip() function can lead to an error. To handle this error, you can use the try-except block to catch and handle the RuntimeError.
Here’s how:
3. Custom Exceptions
In addition to built-in exceptions like TypeError and RuntimeError, you can also define custom exceptions to handle specific scenarios that may arise when working with zip().
For instance, you can define a custom exception called UnequalLengthError to handle situations where the input iterables have unequal lengths.
Here’s how:
By creating custom exceptions, you can provide more specific error messages and implement dedicated error-handling logic tailored to your application’s requirements.
In summary, when working with zip() in Python, you should also be aware of the different kinds of errors that can occur. These errors can occur due to incompatible types, unexpected runtime conditions, or specific scenarios you define.
By utilizing the proper exception-handling techniques we’ve explained, you can effectively handle these errors and ensure the smooth execution of your code.
Final Thoughts
The Python zip() function is a powerful tool that allows you to combine and iterate over multiple iterables in parallel. By using zip(), you can simplify complex tasks, improve code readability, and enhance the efficiency of your Python programs.
We explored various aspects of zip(), including its syntax, usage, and common operations. You learned how to combine iterables, create dictionaries, handle different lengths of iterables, unzip tuples, and work with tuples efficiently.
We also delved into advanced functionality, such as processing multiple files in parallel and working with ZIP archives.
Mastering zip() expands your data manipulation and iteration capabilities, making you a more proficient Python developer. So continue exploring its features and incorporating them into your projects. With zip(), you’re equipped with a versatile tool to tackle a wide range of programming challenges.
Happy coding!
Frequently Asked Questions
What is the zip() function in Python?
zip() in Python is a built-in function that takes multiple iterable objects (such as lists, tuples, or strings) as input and returns an iterator of tuples.
Each tuple contains elements from the input iterables, paired together based on their respective positions. In other words, it aggregates elements from multiple iterables into a single iterable.
How do you write a zip function in Python?
The zip() function is a built-in function in Python readily available for use. You can directly use it by passing the desired iterables as arguments to the function using the following syntax:
Code Examples

Output:

How to create dictionary in Python using zip function?
To create a dictionary in Python using the zip() function, you can pass two iterables to zip(): a single iterable argument containing the keys and the other containing the values. Then, you can convert the resulting iterable of tuples into a dictionary using the dict() constructor.
Sample code:
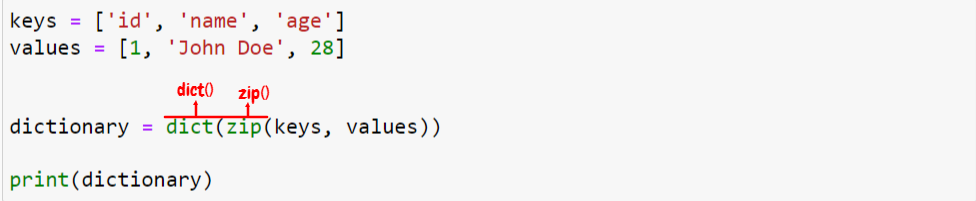
Output:

Can you zip 3 lists in Python?
Yes, you can use the zip() function to zip three lists in Python. Simply pass one or more iterables as arguments to the zip() function, and it will return an iterator of tuples, where each tuple contains elements from the corresponding positions of the input lists.
Output: