Python dictionaries are an incredibly useful data structure that you’ll find yourself reaching for frequently in your programming endeavors. Their unique ability to map keys to their respective values allows for fast, efficient access and simplified code when managing complex datasets.
Python dictionaries are essentially an implementation of associative arrays. They provide a flexible and efficient way to store and manipulate data in the form of key-value pairs.
They are very versatile and can accommodate changeable and ordered collections without allowing duplicate keys.
As we dive into Python dictionaries in this article, you’ll find them to be powerful tools for organizing and accessing your data.
So, be sure to familiarize yourself with the ins and outs of Python dictionaries to unlock their full potential in your projects!
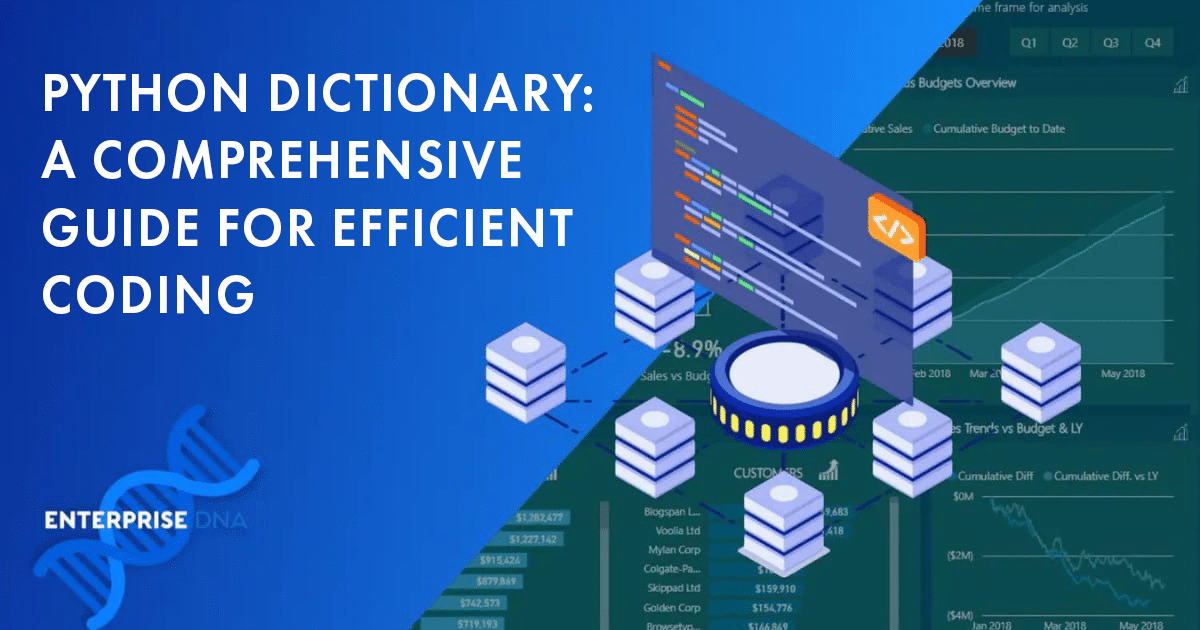
Understanding Python Dictionaries
A dictionary in Python is a powerful and flexible data structure that allows you to store a collection of key-value pairs. Think of a dictionary as a way to map keys to their corresponding values, making it easy to retrieve information that’s related to a specific identifier.
To create a dictionary, you can use curly braces ( { } ) enclosing a comma-separated list of key-value pairs. For example:
your_dict = {"key1": "value1", "key2": "value2", "key3": "value3"}
Keep in mind that dictionaries in Python are mutable, which means you can modify their contents after they’re created. To add or update a key-value pair, simply use the following syntax:
your_dict["new_key"] = "new_value"
#You can also update an old key's value
your_dict["old_key"] = "new_value"
When it comes to accessing values in a dictionary, you only need the key:
value = your_dict["key1"]
In case you try to access a key that doesn’t exist in the dictionary, you will encounter a KeyError. To avoid this, you can use the get() method:
value = your_dict.get("non_existent_key", "default_value")
One thing you have to remember is that a Python dictionary cannot contain duplicate keys. Each key must be unique and be of an immutable data type.
For example, you can use a tuple, String, Integer, or even a Boolean for your key. However, you can’t use lists, dictionaries, or sets.
There are no such restrictions for values. You can use both mutable and immutable data types.
Removing an element from a dictionary is done by using the del keyword:
del your_dict["key1"]
They also provide several methods for iterating through their contents. For instance, you can loop over the dictionary keys, values, or even both:
for key in your_dict.keys():
print(key)
for value in your_dict.values():
print(value)
for key, value in your_dict.items():
print(key, value)
You can now use these dictionary methods and techniques to efficiently store, retrieve and manipulate data while working with Python.
How to Create a Python Dictionary
There are two main ways you can create Python dictionaries: using curly brackets and the dict() constructor.
Using Curly Brackets
One method you can use to create a dictionary is by utilizing curly brackets({}). To initialize an empty dictionary, you can simply use empty_dict = {}.
To create a dictionary with key-value pairs, separate the keys and values with colons. Next, separate each pair with a comma:
python_dict = {
'key1': 'value1',
'key2': 'value2',
'key3': 'value3'
}
Using this format, you’ve created a Python dictionary with three key-value pairs. Remember that the keys must be unique while the dictionary values can be duplicated.
Using Dict Constructor
Another method for creating a Python dictionary is to use the dict() constructor. To create an empty dictionary, simply call this function without any arguments: empty_dict = dict().
To create a dictionary using the dict() constructor, you can use the following syntax:
python_dict = dict(
key1='value1',
key2='value2',
key3='value3'
)
This method also creates a Python dictionary with three key-value elements. Note that, unlike the curly brackets method, the dict() constructor does not require quotes around the keys.
However, the dictionary values still require quotes around them
Now that you’re familiar with two ways of creating Python dictionaries, you can start implementing them in your code and harness their powerful features in your applications.
How to Access Dictionary Elements
In this section, you will learn about different ways to access elements in a dictionary. Dictionaries store data in key-value pairs, and you can access each dictionary value using their respective keys.
We will cover two popular methods: Using keys and the get method.
Using Keys
One of the most straightforward ways to access dictionary elements is by using the keys. To get the value of a particular dictionary key, simply put the key inside square brackets next to the dictionary name:
my_dict = {'name': 'John', 'age': 30, 'city': 'New York'}
name_value = my_dict['name']
phone_value = mydict['phone-number']
In this example, name_value would store the string ‘John’. Note that if you try to access a nonexistent key, Python will raise a KeyError.
Using Get Method
An alternative to using keys directly is the get() method, which allows you to access dictionary elements without the risk of raising a KeyError. Instead, the get method returns a default value if the specified key is not found:
my_dict = {'name': 'John', 'age': 30, 'city': 'New York'}
name_value = my_dict.get('name', 'Key not found')
country_value = my_dict.get('country', 'Key not found')
In this example, name_value would store the string ‘John‘ as before since the ‘name‘ key is present in the dictionary. However, country_value will store the default value ‘Key not found‘ since the ‘country‘ key does not exist in the dictionary.
The get() method provides a more flexible way of accessing dictionary elements, as it allows you to handle missing keys gracefully. You can choose to use either method depending on your requirements and the possibility of encountering nonexistent keys.
How to Modify Dictionary Elements
In this section, you’ll learn how to modify elements within a Python dictionary. There are two primary operations for accomplishing this: adding new elements and updating existing ones.
Both of these methods will help you maintain the accuracy and usefulness of your dictionary. Let’s look at them:
Adding New Elements
Adding a new element to a dictionary is simple. To do so, specify the new key-value pair using an assignment operator, like this:
your_dict = {"key1": "value1", "key2": "value2"}
your_dict["new_key"] = "new_value"
After executing the above code snippet, your dictionary will now include the new pair:
{"key1": "value1", "key2": "value2", "new_key": "new_value"}
You can also add multiple key-value pairs at once by using the dictionary’s update() method
new_elements = {"key3": "value3", "key4": "value4"}
your_dict.update(new_elements)
Now, your dictionary will contain the following entries:
{"key1": "value1", "key2": "value2", "key3": "value3", "key4": "value4"}
Updating Existing Elements
To update the value of an existing element, you simply need to reference the key and assign a new value to it:
your_dict["key1"] = "updated_value1"
This change will result in the following dictionary:
{"key1": "updated_value1", "key2": "value2", "key3": "value3", "key4": "value4"}
If you need to update multiple elements at once, use the update() method again. To use it, pass in a new dictionary containing the desired modifications.
For example, let’s update the your_dict dictionary with the values from the updates dictionary:
updates = {"key2": "updated_value2", "key4": "updated_value4"}
your_dict.update(updates)
With these updates applied, the your_dict dictionary will look like this:
{"key1": "updated_value1", "key2": "updated_value2", "key3": "value3", "key4": "updated_value4"}
These operations are essential tools when working as they allow you to keep your data structures up-to-date and accurate.
Deleting Dictionary Elements
In this section, we will explore two common ways to delete dictionary elements in Python: the del keyword and the clear() method.
Del Keyword
The del keyword is used to remove a specific key-value pair from a dictionary. It operates in-place and raises a KeyError if the key does not exist in the dictionary. To delete an element, simply use the following syntax:
del my_dict[key]
For example, if you want to delete a key called “brand” from a dictionary named my_dict, you will write:
del my_dict["brand"]
Keep in mind that it is a good practice to ensure that the key exists in the dictionary before using the del keyword. You can do this by checking if the key is in the dictionary:
if "brand" in my_dict:
del my_dict["brand"]
Clear Method
The clear() method is another way to delete dictionary elements. This method removes all dictionary keys and values in one operation, resulting in an empty dictionary.
To use the clear() method on your dictionary, simply call it. For example, if you have an existing dictionary named my_dict and you want to remove all its contents, you would write:
my_dict.clear()
In conclusion, you have learned two methods to delete elements from a Python dictionary: the del keyword for removing individual key-value pairs and the clear() method for emptying an entire dictionary.
What Are Some Common Dictionary Methods?
In this section, we will explore some common Python dictionary methods. These built-in methods will help you manipulate and modify dictionaries to suit your needs.
Keys
To retrieve all the keys from your dictionary, you can use the keys() method. This will return a view object containing all the dictionary’s keys.
For example:
your_dict = {'a': 1, 'b': 2, 'c': 3}
key_view = your_dict.keys()
print(key_view)
# Output: dict_keys(['a', 'b', 'c'])
Values
Similarly, the values() method allows you to obtain all the values stored in the dictionary. This is also presented as a view object.
For instance:
value_view = your_dict.values()
print(value_view)
# Output: dict_values([1, 2, 3])
Items
If you need to access both keys and values simultaneously, use the items() method. This returns a view object containing key-value pairs in the form of tuples.
Let’s look at an example:
item_view = your_dict.items()
print(item_view)
# Output: dict_items([('a', 1), ('b', 2), ('c', 3)])
Update
The update() method allows you to modify your dictionary by adding or updating key-value elements. To use the method, you have to provide a dictionary as an argument.
To merge two dictionaries or update specific keys, use this method:
your_dict.update({'d': 4, 'e': 5})
print(your_dict)
# Output: {'a': 1, 'b': 2, 'c': 3, 'd': 4, 'e': 5}
Pop
Using the pop() method, you can remove a specific item from the dictionary. To use it, provide the key to be removed as an argument in the method.
The method will return the value associated with the specified key and remove it from the dictionary:
removed_value = your_dict.pop('a')
print(removed_value)
# Output: 1
print(your_dict)
# Output: {'b': 2, 'c': 3, 'd': 4, 'e': 5}
Popitem
The popitem() method removes the last key-value pair in the dictionary. It returns a tuple containing the removed key and value. For example:
removed_item = your_dict.popitem()
print(removed_item)
# Output: ('e', 5)
print(your_dict)
# Output: {'b': 2, 'c': 3, 'd': 4}
By using these methods, you can effectively manage and manipulate your dictionaries to achieve a variety of tasks.
Using Python Dictionaries
In this section, you will learn different techniques to work with dictionaries, such as looping over them, using dictionary comprehensions, and working with nested dictionaries.
Looping Over Dictionaries
To loop over a dictionary, you can use the built-in method items() to iterate over key-value pairs. This allows you to perform operations on both keys and values in a readable and efficient manner.
Here is an example:
my_dict = {"apple": 1, "banana": 2, "cherry": 3}
for key, value in my_dict.items():
print(key, value)
Alternatively, you can use the keys() and values() methods to iterate over either the dictionary keys or values exclusively.
What Are Dictionary Comprehensions?
Dictionary comprehensions are a concise way to create dictionaries using a single line of code. They are similar to list comprehensions but work with dictionaries instead.
Here is an example of a dictionary comprehension that squares the numbers from 1 to 5:
squared_numbers = {num: num**2 for num in range(1, 6)}
Dictionary comprehensions can be highly valuable for tasks like filtering dictionaries or transforming their values.
What Are Nested Dictionaries?
Nested dictionaries are dictionaries that contain other dictionaries as values. They can be used to represent complex hierarchical data structures.
Here’s an example of a nested dictionary:
nested_dict = {
"person1": {"name": "Alice", "age": 30},
"person2": {"name": "Bob", "age": 24}
}
To access an element in a nested dictionary, use multiple indexing:
name_p1 = nested_dict["person1"]["name"]
You can further edit or manipulate elements in the nested dictionaries as needed. Remember to keep track of the keys and nesting levels when working with complex nested dictionaries to avoid confusion or errors.
Common Dictionary Use Cases
Python dictionaries are versatile, enabling you to organize and manage various data types. In this section, you will explore some common use cases for dictionaries, which will enhance your understanding and help you implement them effectively in your projects.
1. Storing key-value data: Dictionaries are ideal for storing data with unique keys and associated values, such as usernames and user information or product IDs and product details:
{
"username1": {"name": "Alice", "email": "[email protected]"},
"username2": {"name": "Bob", "email": "[email protected]"}
}
In this video, we use a dictionary to store data values gotten from a GoogleNews API call.
2. Counting occurrences: You can use dictionaries to count the occurrences of items, such as the frequency of words in a document. Using keys to represent the unique items, and values to indicate the count, you can effectively manage such data.
Fun fact, you can do this with the Counter class in the Python Collections module. It takes in an unordered list and returns a dictionary with each unique element as a key and their frequency as their respective value.
3. Mapping keys to multiple values: If you need to store multiple values for each dictionary key, you can use lists or sets as values in your dictionary:
{
"fruits": ["apple", "banana", "mango"],
"vegetables": ["carrot", "broccoli", "spinach"]
}
4. Caching computed results: Dictionaries can be used for caching results of expensive operations. This is especially useful in memoization techniques and dynamic programming, where you store the results of computations to avoid redundant calculations.
5. Creating inverted indexes: In cases like search engines, you may require an inverted index that maps each search term to a list of resources that contain the term. Utilizing dictionaries allows you to store this data efficiently:
{
"python": ["resource1", "resource3"],
"dictionary": ["resource2", "resource3", "resource4"]
}
These are just a few examples to illustrate the power and versatility of Python dictionaries. By understanding these use cases and their applications, you can harness the full potential of dictionaries to build efficient solutions for your programming tasks!