Functions are an essential concept in programming that enables you to manage and reuse blocks of code. Knowing how to call a function in Python is a key skill to learn.
In this tutorial, you’ll learn the basics of calling functions, along with some tips for writing efficient code that is easier to understand.
Mastering functions will not only help you create more versatile code but also streamline your programming process and improve your overall efficiency as a developer.
Functions have the same underlying principles, and once you master functions in Python, you’ll be able to expand your understanding of functions compared to other programming languages like Java, R, JavaScript, PHP, etc.
What is a Function?
A function in Python allows you to create reusable pieces of code that can be called throughout your program, simplifying your code and improving readability. It performs a specific task or set of tasks and can be called multiple times within a program.
Defining a Function in Python
There are two main categories of functions in Python:
- User-defined Functions: Functions that a programmer defines to avoid repetition of code
- Built-in Functions: Functions that are defined by someone else and can be used in your code after installing the necessary packages
This section of the Python tutorial focuses on user-defined functions. You’ll learn:
- How to define a function in Python
- The function syntax
- How to use parameters and arguments
Function Syntax
The def
keyword, followed by the function name and parentheses, allows you to define a function in Python. The function body is indented and contains the code to be executed when the function is called. An example of a basic function in Python is given below:
def greet(): print("Hello, World!") greet()
The def
keyword defines a new function with the name greet. The third line of code invokes the function, which executes the code inside the function.
The print
line in the code above is also a function. However, if you are working in a Python 2 environment, it is defined as a statement. Later, in Python 3, it was changed from statement to a function.
Parameters and Arguments
Parameters are the placeholders in the function definition, while arguments are the values passed when calling the function.
To specify parameters, include them within the parentheses of the function definition, separated by commas if there are multiple parameters. When you call a function, you provide the input values called arguments. The following example demonstrates defining a function that takes two parameters:
def add_numbers(a, b): return a+b
The return
statement in the function’s body returns the addition of the two arguments passed into it. You can think of it as a calculator, where you input values and get the desired result in return.
After defining the function, we can call it with arguments of our choice.
add_numbers(3, 5)
The kwargs Argument
kwargs
is short for “keyword arguments” in Python. It allows you to extend a function’s flexibility by permitting the caller to specify any number of keyword arguments when calling the function. The keyword arguments are passed as a dictionary to the function.
In a function definition, you can use the double asterisk **
syntax before the parameter name to accept keyword arguments.
def my_function(**kwargs): for key, value in kwargs.items(): print(f"{key} = {value}") my_function(a=1, b=2, c=3)
In this example, kwargs
will be a dictionary containing the keyword arguments passed to the function. The output is shown in the image below:
Calling a Function
Calling a function means executing the code inside the function’s body. There are four types of function calls in Python:
- Basic Function Call
- Calling with Positional Arguments
- Calling with Keyword Arguments
Basic Function Call
A basic function call invokes the function without passing any arguments into the function. After defining a function, you can call it by using its name followed by parentheses.
If the function does not take any arguments, the parentheses will be empty. The code below is an example of a basic function.
def welcome_message(): print("Welcome to Enterprise DNA!") welcome_message()
Running the code above gives the following output:
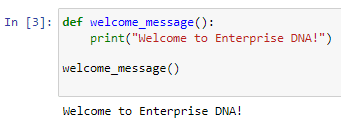
Positional Arguments
Positional arguments are the most common type of argument, and they are passed to the function in the same order as they are specified. The following is an example of a function call with positional arguments:
def welcome_message(name): print(f"Welcome to Enterprise DNA, {name}!") welcome_message("John")
In the example above, the welcome_message()
function takes one argument, name
. When you call this function with the argument John
, it will print the message next to the name.
The code above gives the following output:
Keyword Arguments
Another way to pass arguments to a function is by using keyword arguments. Keyword arguments allow you to specify the name of the parameter along with its value, making the code more readable and reducing the chance of passing arguments in the wrong order.
The example below uses keyword arguments to call the welcome_message
function:
def welcome_message(name, message='Welcome to Enterprise DNA!'): print(message, name) welcome_message(name='Alice', message='Good morning')
In this example, we have defined a function welcome_message()
that has two parameters, name
, and message
. The message parameter has a default argument of Welcome to Enterprise DNA!
When calling the function, we use keyword arguments to pass the value John
, resulting in the output Welcome to Enterprise DNA! John.
Return Values
When working with functions, you’ll often want to return the result of operations inside your function’s body. Return values allow a function to send a result back to the caller. By understanding how to work with single and multiple return values, you can enhance the functionality of your Python programs.
Single Return Value
A single return value function returns one value from the operations in a function.
An example of a single return value function is given below:
def get_greeting(name): return "Hello, " + name + "!" greeting = get_greeting("Alice") print(greeting)
In the example above, the function get_greeting
takes a name as its function argument compiles it and returns a personalized greeting. The return value is then stored in the variable greeting and printed to the console.
Multiple Return Values
At times, you will be required to return more than one value from a function in Python. To achieve this, you can use a tuple or a list to wrap the return values.
Following is an example of multiple return values function in Python programming language.
def get_name_and_age(): return ("John", 30) name, age = get_name_and_age() print(name) print(age)
In this example, get_name_and_age
is a function that returns a tuple with two values: a name
and an age
.
You can also return a list of values from a function in Python. The function below demonstrates a list return value from a function.
def calculate_areas(height, width): return [height * width, (height * width) / 2] area, triangle_area = calculate_areas(10, 5) print(area) print(triangle_area)
In this case, the function calculate_areas
takes two arguments, height
, and width
, and returns a list containing the area of a rectangle and the area of a triangle.
Passing Python Functions as Arguments
Python allows you to pass functions as arguments to other functions. When a function accepts another function as an argument, it is referred to as a higher-order function.
The code below is an example of passing a function to another function in Python:
def square(x): return x * x def double(x): return x * 2 def apply_func(func, x): return func(x) result = apply_func(square, 5)
Nested Functions
Nested functions are functions defined within another function, also known as inner functions. These can be useful when creating closures and modular code.
To create a nested function, simply define a function within another function:
def outer_function(x, y): def inner_function(z): return x + y + z result = inner_function(3) return result # Call the outer function print(outer_function(1, 2))
In the example above, the inner_function is defined within the outer_function. The inner function has access to the variables of the outer function, allowing it to perform operations on them.
Lambda Functions
Lambda functions, also known as anonymous functions, are a way to create small, one-time-use functions in Python. In data science projects, you’ll often be working with Lambda functions for creating efficient data structures and pipelines.
To create a lambda function, you can use the lambda keyword followed by a list of arguments, a colon, and then an expression. The expression is what the lambda function will return once it’s called. The following is an example of lambda function in Python:
add = lambda a, b: a + b result = add(3, 4) print(result) # Output: 7
In the example above, we created a lambda function that takes two arguments (a
and b
) and returns their sum. We assigned this lambda function to a variable called add
.
Lambda can be useful in the following scenarios:
- As arguments for higher-order functions like map(), filter(), and sorted().
- As key functions when sorting lists or dictionaries.
The following example demonstrates using higher order function filter() with lambda:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9] even_numbers = list(filter(lambda x: x % 2 == 0, numbers)) print(even_numbers) # Output: [2, 4, 6, 8]
In this example, we used a lambda function to define the filtering criteria for the filter() function. The lambda function checks if a number is even, and the filter() function returns a new list containing only the even numbers.
To see an example of how functions can be useful in different data science scenarios, check the video given below:
Recursion
Recursion is a technique in programming where a function calls itself. This can be a useful way to solve complex problems by breaking them down into smaller, identical subproblems.
When creating a recursive function, you need to keep two things in mind: the base case and the recursive case.
- The base case is the condition that stops the recursion
- The recursive case is where the function calls itself. The recursive case acts as an iterator for the function
The following example demonstrates recursive functions in Python:
def factorial(n): if n == 1: return 1 return n * factorial(n - 1) factorial(5)
In this example, the base case is when n == 1
, and the function returns 1
. The recursive case occurs when n is not equal to 1, and the function calls itself with n - 1
.
Common Errors and Troubleshooting
If you are a beginner programmer, you’ll often run into errors when implementing functions such as recursive and lambda functions. The following sections highlight a few common errors and troubleshooting tips to help you resolve them efficiently.
1. TypeError: missing a required argument: This error occurs when you don’t supply the correct number of arguments that the function expects.
def my_function(fname, lname): print(fname, lname) # Correct call my_function("John", "Doe") # Will raise an error my_function("John")
To fix this error, make sure you are passing the correct number of arguments to your function.
2. TypeError: unsupported operand type. This error may occur when you’re trying to use an operator with incompatible types.
def add_number(a, b): return a + b # Correct call result = add_number(5, 3) print(result) # Will raise an error due to incorrect args result = add_number("5", 3) print(result)
To fix this error, check that your functions are dealing with the right data types, and consider using type casting if necessary
3. NameError: name ‘function_name’ is not defined: This error suggests that the function hasn’t been defined before it’s being called.
# Correct order def greet(): print("Hello, there!") greet() # Incorrect order goodbye() def goodbye(): print("Goodbye!")
To fix this error, ensure that your function is defined properly and that you call it after its definition.
Conclusion
To recap and help you solidify your understanding, the following points summarize the key aspects of calling functions in Python:
- To call a function, use the function name followed by parentheses (e.g.,
function_name()
). - If the function takes arguments, include them within the parentheses (e.g.,
function_name(arg1, arg2)
). - You can create your own functions using the def keyword, defining the function’s scope and any required arguments.
- When calling a function that returns a value, you can assign the result to a variable for later use (e.g.,
result = function_name(args)
).