One of the many reasons for Python’s popularity is the extensive collection of data structures that it offers to its users.
Among these data structures, lists play a crucial role in organizing and manipulating data. A major part of working with lists involves adding new elements to them, and Python provides several methods to perform this task seamlessly.
The append() method in Python is a popular function for adding elements to a list. It offers you an easy way to include a new element at the end of an existing list. This method is particularly useful in a variety of tasks, such as appending items while iterating over a collection or populating lists using loops. Python’s append() method stands out as an in-place operation, which means it modifies the list directly without requiring a separate output list.
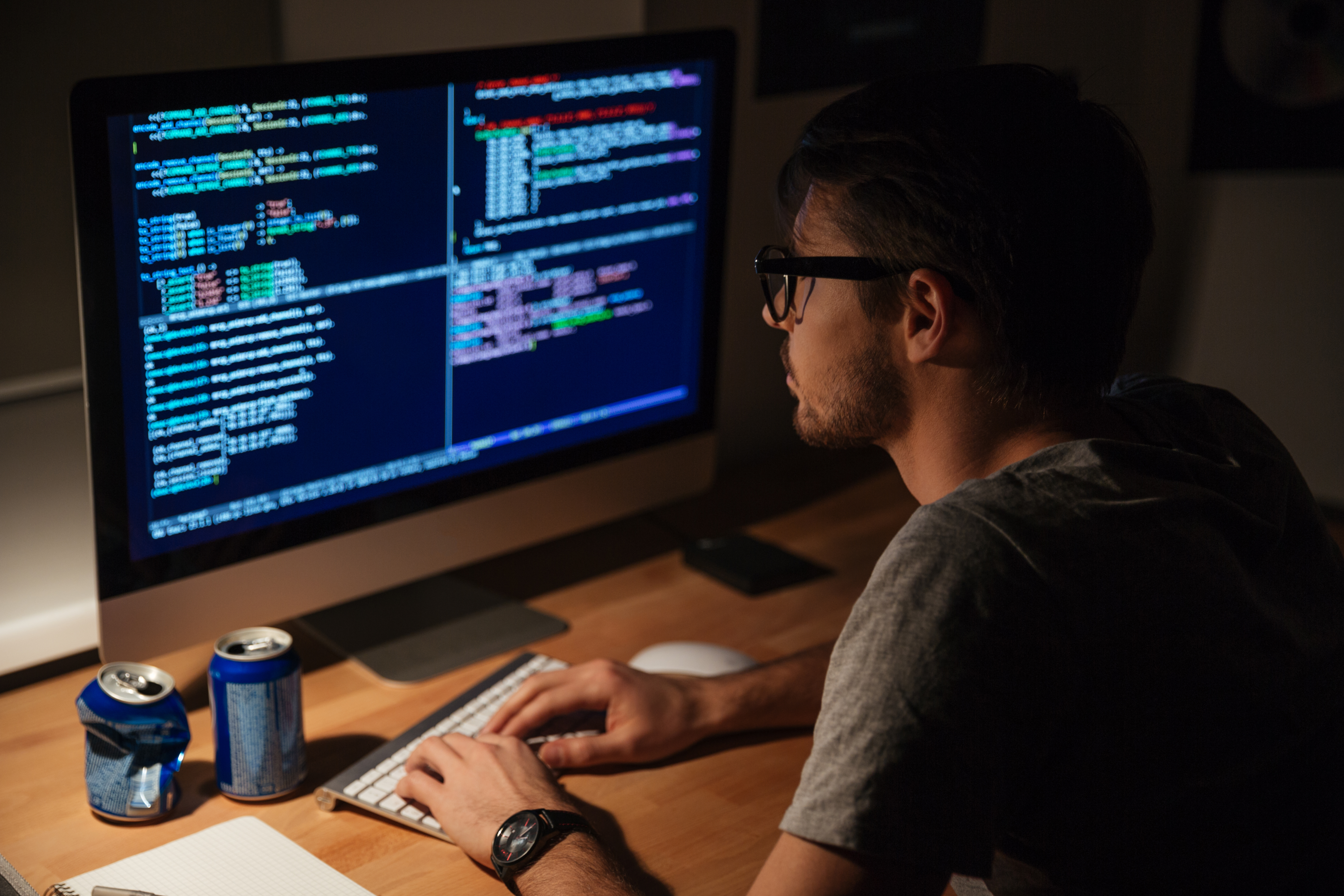
In this article, we dive deep on appending elements to a list in Python. Furthermore, we will also explore alternatives to the append() function.
Showcasing the alternatives will help you in choosing the best method for your use case.
Let’s get into it!
What is a Python List?
Before we get into appending lists in Python, it’s important that you have a solid understanding of the basics of Python lists.
This will help you utilize the more advanced ways of appending lists.
A Python list is an important data structure that allows you to store multiple elements in an ordered and mutable way.
You can create a Python list by placing elements inside square brackets. It is recommended to have elements of the same data type in lists.
We’ve listed some important pointers about lists below:
- Mutable Objects: Lists in Python are mutable, which means that you can change their content without changing their identity. You can modify a list by adding, removing, or changing all the elements.
- Ordered: Lists maintain the order of elements as they were initially added. They are not sorted automatically. The order of elements matters in a list and can be used to access and manipulate elements.
- Indexing: List elements are accessed by their index. Python uses zero-based indexing, which means that the first element is at index 0, the second at index 1, and so forth. You can also use negative indexing to access elements from the end of the list (-1 refers to the last item, -2 refers to the second last item, etc.).
- Duplicates Allowed: Lists can contain duplicate elements. They also support elements of different data types (e.g., integers, dictionaries, floating point numbers).
- Size Flexibility: Lists can grow or shrink dynamically based on the operations performed on them.
4 Ways to Append Python Lists
After reviewing the basics in the previous section, let’s discuss 4 ways of appending Python lists.
Each method will be followed by examples to help you better understand its use cases.
We will learn appending Python lists with the following methods:
- Using append() method
- Using extend() method
- Using insert() method
- Using + operator
1) How to Append Using append() method
The append() list method in Python is used to add a single item to the end of a list. This means that the order of the elements is the same as the order in which you added them.
The following is an example of appending an element to a list:
# Start with an empty list
my_list = []
# Add an element to the list
my_list.append("Hello")
# Now the list contains one element
print(my_list) # Output: ['Hello']
# Add another element
my_list.append("World")
# Now the list contains two elements
print(my_list) # Output: ['Hello', 'World']
In this example, we started with an empty list and used append() to add two elements to the same list. After each append(), we printed the list to show how it was updated.
The append() method is often used in conjunction with a loop to dynamically add elements to a list.
This is a common pattern when you want to build up a list based on some kind of computation or based on values from another list or iterable such as a tuple object.
The following is an example of appending lists using append() in a loop:
# Start with an empty list
squares = []
# Loop over the numbers from 0 to 4
for x in range(5):
# Compute the square of the number
square = x ** 2
# Append the square to the list
squares.append(square)
# Print the list of squares
print(squares) # Output: [0, 1, 4, 9, 16]
In this example, the for loop iterates over the numbers from 0 to 4. On each iteration, it computes the square of the current number and appends it to the squares list.
The squares list starts out empty and grows on each iteration of the loop.
2) How to Append Using extend() Method
You can use the extend() method in Python to add multiple elements to a list at once.
Unlike append(), which adds its argument as a single element, extend() adds the elements of its argument one by one. The argument should be an iterable, such as a list or a tuple.
The following is an example of this method:
# Start with a list
my_list = [1, 2, 3]
# Extend the list with another list
my_list.extend([4, 5, 6])
# Now the list contains six elements
print(my_list) # Output: [1, 2, 3, 4, 5, 6]
In this example, we started with a list of three elements. Then, we extended this list with another list containing three more elements.
After calling extend(), the original list contains all six elements.
3) How to Append Using insert() Method
You can use the insert() method in Python to add an element at a specific position in the list.
It is similar to the append() function but the only difference is that insert() gives you control over the position where the new element is added.
The input parameter of insert() method are:
- The index at which the new element should be inserted.
- The new element itself.
The insert() method has other optional arguments for further customization as well.
The following is an example of Python list append using insert() method:
# Start with a list
my_list = [1, 3, 4]
# Insert a new element at position 1
my_list.insert(1, 2)
# Now the list contains four elements
print(my_list) # Output: [1, 2, 3, 4]
In this example, we started with a single object of three elements. Then, we inserted the number 2 at position 1. List indices start at 0 in Python, so the number 2 is now the second element in the list.
The other elements were moved to the right to make room for the new element.
4) How to Append Using + Operator
You can use the + operator to concatenate, or join together, two or more lists. This is appending the elements of one list to another list.
However, unlike the append(), extend(), and insert() methods, the + operator does not modify the original lists. Instead, it creates a new list that contains the elements of both original lists.
The following example demonstrates this method:
# Create lists
list1 = [1, 2, 3]
list2 = [4, 5, 6]
# Use the + operator to concatenate the lists
list3 = list1 + list2
# list3 contains the elements of both original lists
print(list3) # Output: [1, 2, 3, 4, 5, 6]
In this example, list1 and list2 are not modified when we create list3. The appended list is shown below:
This method is also referred to as concatenation and works for multiple lists as well.
Advanced Append Techniques
In Python, working with complex data structures often involves lists and their manipulation using built-in methods such as append().
There are 2 data structures where lists are commonly utilized:
- Stacks
- Queues
Stacks can be implemented using Python lists, where elements are added and removed from the end of the list using the append() and pop() methods.
The following is an example of this method:
stack = []
stack.append("apple")
stack.append("banana")
stack.append("cherry")
print(stack) # Output: ['apple', 'banana', 'cherry']
stack.pop() # Output: 'cherry'
This Python script initializes an empty list stack, then adds the strings “apple”, “banana”, and “cherry” to it using the append() method.
After printing the list, it removes and returns the last element (“cherry”) from the list using the pop() method.
For queues, the append() method can be used to enqueue elements at the end of the list. To dequeue elements from the front, you can use the pop(0) method. However, this method is inefficient due to shifts in the remaining list elements.
To avoid this, you can use Python’s built-in deque class from the collections module:
from collections import deque
queue = deque()
queue.append("apple")
queue.append("banana")
queue.append("cherry")
print(queue) # Output: deque(['apple', 'banana', 'cherry'])
queue.popleft() # Output: 'apple'
This Python script starts by importing the deque class from the collections module and initializes an empty deque named queue.
It then appends the strings “apple”, “banana”, and “cherry” to the deque, prints it, and finally removes and returns the first element (“apple”) from the deque using the popleft() method.
Python offers a number of ways to work with complex data structures while maintaining readability and efficiency. The append() method is especially useful when dealing with lists and their variations, such as stacks and queues.
Learn more about working with data by watching the following video:
Final Thoughts
Learning to append lists helps streamline your code, making it cleaner and more efficient.
Whether you’re developing applications, automating tasks, or analyzing data, you’ll find that manipulating lists is a daily necessity.
Furthermore, it allows you to organize, store, and manipulate data effectively, which is why it’s crucial to become proficient at it.
The append() function, along with its counterparts like extend(), insert(), list concatenation, list comprehension, and the unpacking operator, provide diverse ways to modify lists.
Each method has its own strengths, and understanding when to use it can help you write more efficient and readable code.
Frequently Asked Questions
In this section, you’ll find some frequently asked questions that you may have when working with appending lists in Python.
How do I add an item to a list in Python?
To add an item to a list in Python, you can use the .append() method. This method adds an element to the end of the existing list.
Here’s an example:
my_list = [1, 2, 3]
my_list.append(4)
print(my_list) # Output: [1, 2, 3, 4]
What is the difference between append() and extend()?
append() adds an element to the end of a list, while extend() adds multiple elements from an iterable, such as a list or a tuple, to the end of a list.
Here’s an example to illustrate the difference:
my_list = [1, 2, 3]
my_list.append([4, 5])
print(my_list) # Output: [1, 2, 3, [4, 5]]
my_list = [1, 2, 3]
my_list.extend([4, 5])
print(my_list) # Output: [1, 2, 3, 4, 5]
How do I concatenate two lists in Python?
You can concatenate two lists in Python by using the + operator.
Here’s an example:
list1 = [1, 2, 3]
list2 = [4, 5, 6]
combined_list = list1 + list2
print(combined_list) # Output: [1, 2, 3, 4, 5, 6]
How can I insert a single element at a specific index in a list?
To insert an element at a specific index in a list, you can use the .insert() method. This method takes two arguments: the index at which you want to insert the element and the element itself.
Here’s an example:
my_list = [1, 2, 4]
my_list.insert(2, 3)
print(my_list) # Output: [1, 2, 3, 4]
Is it possible to append a string to a list?
Yes, it is possible to append a string to a list. The append() method will treat the string as a single element and add it to the end of the list.
Here’s an example:
my_list = ['apple', 'banana']
my_list.append('orange')
print(my_list) # Output: ['apple', 'banana', 'orange']