Iteration is an important task when working with data in Python. There are several data structures in different Python versions, each with its own iteration methods. Dictionaries are very common when working with data, and learning how to iterate through a dictionary can help you unlock its full potential.
There are multiple ways to iterate through Python dictionaries. You can simply iterate over the keys or use the .keys() method for explicitness. To iterate over values, you can use .values(). For accessing both keys and values simultaneously, .items() is useful. Additionally, you can use enumerate to iterate with an index.
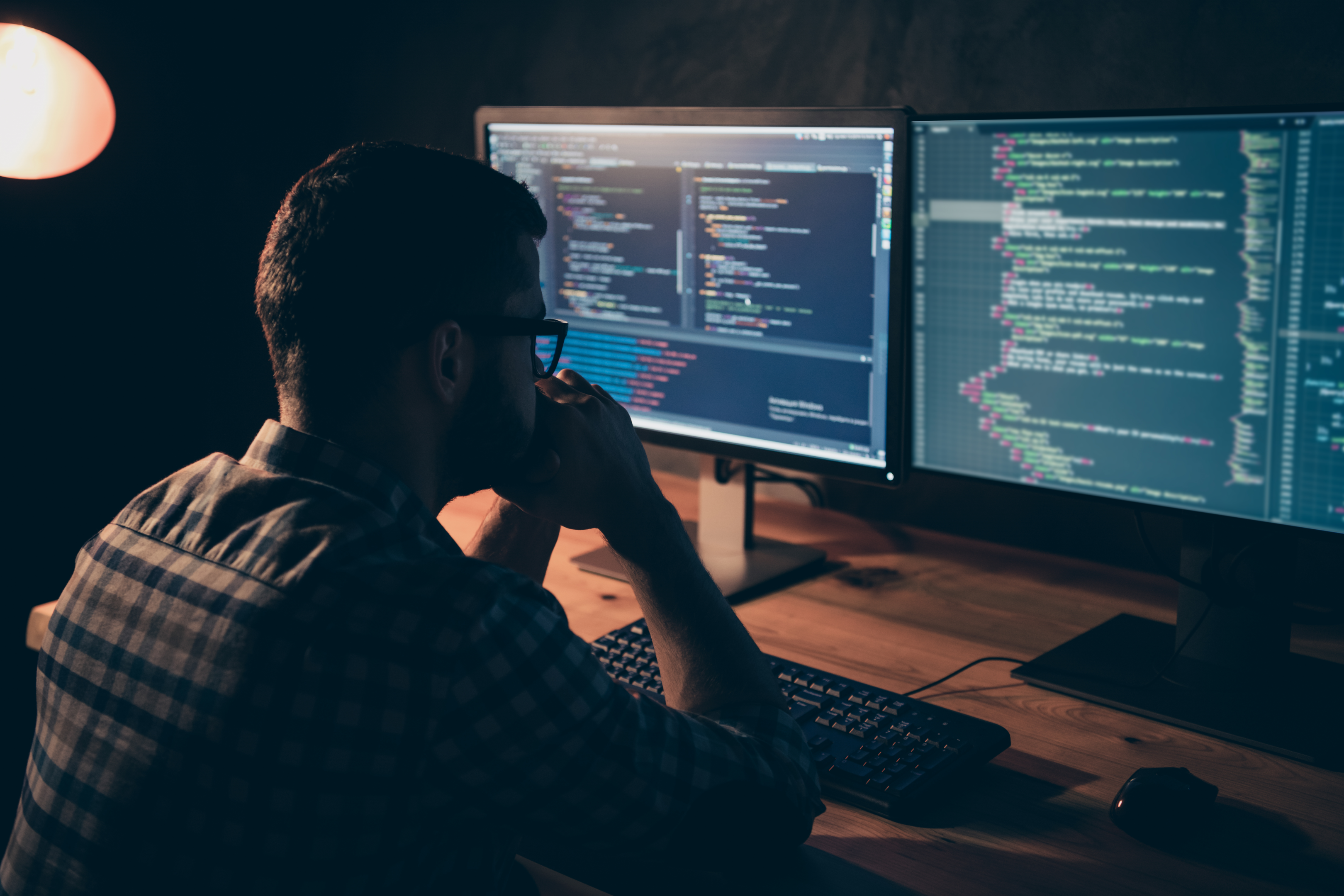
Understanding the art of dictionary iteration can help streamline your Python code. It enables you to manipulate and analyze data with greater ease.
In this article, we’ll explore the various options for iterating through dictionaries and provide useful examples to visualize their practical implications.
Let’s dive in!
What is a Python Dictionary?
Before we start writing code for iterating over a dictionary, it’s crucial that you understand the basics of a Python dictionary.
A dictionary is a data structure in Python that can hold a collection of key-value pairs, where each key must be unique. Dictionaries are mutable, meaning you can change the data and values present in the dictionaries.
You can create dictionaries in Python by using curly braces {} or the dict() constructor. Each key-value pair is separated by a colon, and pairs are separated by commas.
The following is an example of a dictionary:
example_dict_1 = dict() # an empty dictionary
example_dict_2 = {
"apple": 2,
"banana": 3,
"orange": 1
} # a dictionary with key-value pairs
8 Ways to Iterate Through a Dictionary
In this section, we’ll explore 8 ways of iterating through a dictionary in Python. Each method will be followed by examples to help you better understand the concepts.
Specifically, we’ll discuss the following:
- Iterating through the keys
- Iterating through the values
- Iterating through both keys and values
- Iterating using dictionary comprehension
- Iterating using enumerate
- Iterating using the iter() function
- Iterating using the map() function
- Iterating using itertools
1. How to Iterate Through the Keys of a Dictionary
The ‘for’ loop is an important feature of Python, and you can use a simple for loop to iterate through the keys of a Python dictionary.
The following is an example of this method:
my_dict = {"A": 1, "B": 2, "C": 3}
for key in my_dict:
print(key)
This code will print all the keys of the dictionary.
You can also iterate through the dictionary keys using the .keys() method.
The following is an example of this use case:
my_dict = {"A": 1, "B": 2, "C": 3}
for key in my_dict.keys():
print(key)
The output will be the same as the above example.
2. How to Iterate Through the Values of a Dictionary
To iterate through the values of a dictionary, you can use a simple for loop with the .values() method.
The following example illustrates this method:
my_dict = {"A": 1, "B": 2, "C": 3}
for value in my_dict.values():
print(value)
This script will print only the values of a dictionary.
3. How to Iterate Through Both Keys and Values
To access both dictionary keys and values while iterating, you can use the .items() method. This returns a tuple for each key-value pair.
The following example demonstrates this method:
my_dict = {"A": 1, "B": 2, "C": 3}
for key, value in my_dict.items():
print(key, value)
This script will print both the keys and values of a Python dictionary.
4. How to Iterate Using Dictionary Comprehension
Dictionary comprehension (not to be confused with list comprehension) allows you to create a new dictionary from an existing one. In the process, it also allows you to iterate over the existing dictionary.
In the following example, we create a new Python dictionary that contains only the pairs from the original dictionary where the value is greater than 2:
old_dict = {"A": 1, "B": 2, "C": 3, "D": 4, "E": 5}
new_dict = {k: v for k, v in old_dict.items() if v > 2}
print(new_dict) # Output: {'C': 3, 'D': 4, 'E': 5}
The output will be a new dictionary that has all the values that satisfy the condition:
5. How to Iterate a Dictionary Using Enumerate
The enumerate() function is used to add a counter to an iterable and it returns an enumerate object. This can be particularly useful with dictionaries when you want to get the index of each element during the iteration.
To iterate the dictionary using enumerate, you can use the following code:
my_dict = {"A": 1, "B": 2, "C": 3}
for i, (key, value) in enumerate(my_dict.items()):
print(f"Index: {i}, Key: {key}, Value: {value}")
In the above code, the enumerate(my_dict.items()) function produces an iterable series of index-key-value tuples from a dictionary. We use a for loop through a dictionary to unpack and display these elements, with print(f”Index: {i}, Key: {key}, Value: {value}”).
The output is an index along with each key-value pair in the iteration.
6. How to Iterate a Dictionary Using the iter() Function
The iter() function returns an iterator object. When you use it on a dictionary, it iterates over the keys of the dictionary.
The following is an example of iter() function:
my_dict = {"A": 1, "B": 2, "C": 3}
my_iter = iter(my_dict.items())
for _ in my_dict:
key, value = next(my_iter)
print(f"Key: {key}, Value: {value}")
In this example, iter(my_dict.items()) creates an iterator for the key-value pairs in my_dict. The for loop iterates over the dictionary, and key, value = next(my_iter) gets the next key-value pair from the iterator.
7. How to Iterate Using the map() Function
The map() function applies a function to all items in an input iterable. You can use the map() function to iterate over all the values and keys in a dictionary by applying a function to them.
The following code demonstrates iterating through the keys of the dictionary using the map() function:
my_dict = {"A": 1, "B": 2, "C": 3}
for key in map(lambda x: x, my_dict):
print(key)
In this example, the map() function applies the lambda function lambda x: x to the dictionary’s keys. The lambda function simply returns its input, so it doesn’t change the keys.
The output will be:
If you want to iterate over the dictionary values using the map() function, you can use the following code:
for value in map(lambda x: my_dict[x], my_dict):
print(value)
In this example, the map() function applies the lambda function lambda x: my_dict[x] to each key in my_dict. This lambda function returns the value corresponding to its input key, effectively converting the keys to values.
8. How to Iterate a Dictionary Using itertools
The itertools library in Python is a collection of tools for handling iterators.
The itertools.chain() function takes several iterators as arguments and returns a new iterator that yields the items of each one in turn, effectively concatenating the input iterators.
The following example demonstrates this method:
import itertools
dict1 = {'A': 1, 'B': 2}
dict2 = {'C': 3, 'D': 4}
for key, value in itertools.chain(dict1.items(), dict2.items()):
print(f"Key: {key}, Value: {value}")
In this code, itertools.chain(dict1.items(), dict2.items()) creates a new iterator that first yields the key-value pairs of dict1 and then the key-value pairs of dict2.
The output will be both key and value of the dictionary.
Learn more about dictionaries and its applications by watching the following video:
Final Thoughts
As we conclude our exploration of how to iterate through a dictionary in Python, it’s essential to remember why these techniques are so critical for you as a programmer. Learning dictionary iteration enables you to access and manipulate data more effectively. It also empowers you to write clean, efficient, and Pythonic code.
Understanding and implementing these methods provides a solid foundation for data manipulation. Be it in data science, web development, or automation scripts, using dictionary iterations adds tremendous value by facilitating data extraction and transformation tasks.
Frequently Asked Questions
How to loop through key-value pairs in a Python dictionary?
In Python, you can iterate through both keys and values of a dictionary using the .items() method in a for loop:
my_dict = {'a': 1, 'b': 2, 'c': 3}
for key, value in my_dict.items():
print(key, value)
What is the most efficient method to iterate a dictionary in Python?
Using the dictionary’s built-in methods, such as .keys(), .values(), and .items(), is the most efficient way to iterate a dictionary. For example:
my_dict = {'a': 1, 'b': 2, 'c': 3}
for key in my_dict.keys():
print(key)
for value in my_dict.values():
print(value)
for key, value in my_dict.items():
print(key, value)
How can I iterate over a nested dictionary in Python?
To iterate over a nested dictionary, you can use nested for loops:
nested_dict = {'a': {'x': 1, 'y': 2}, 'b': {'x': 3, 'y': 4}, 'c': {'x': 5, 'y': 6}}
for outer_key, outer_value in nested_dict.items():
for inner_key, inner_value in outer_value.items():
print(outer_key, inner_key, inner_value)
How do I iterate over dictionary keys in a specific order in Python?
To iterate over dictionary keys in a specific order, you can use the sorted() function:
my_dict = {'c': 3, 'a': 1, 'b': 2}
for key in sorted(my_dict.keys()):
print(key, my_dict[key])
How to add items to a dictionary while iterating through it in Python?
Modifying a dictionary while iterating over it is not recommended. Instead of directly changing the dictionary, you can create a new dictionary:
original_dict = {'a': 1, 'b': 2, 'c': 3}
new_dict = original_dict.copy()
for key, value in original_dict.items():
if key == 'b':
new_dict['d'] = 4
print(new_dict)