In programming, you may encounter situations where a variable does not have a value. These instances are commonly represented by a special value known as null. Many programming languages, such as C, Java, JavaScript, and PHP, have built-in null values, but Python takes a slightly different approach to representing null variables.
In Python, the equivalent of null is None. It’s a special constant in Python that represents the absence of a value or a null value. It’s an instance of its own datatype — the NoneType. It’s not the same as 0, False, or an empty string as it’s a data type of its own that represents no value or no object.
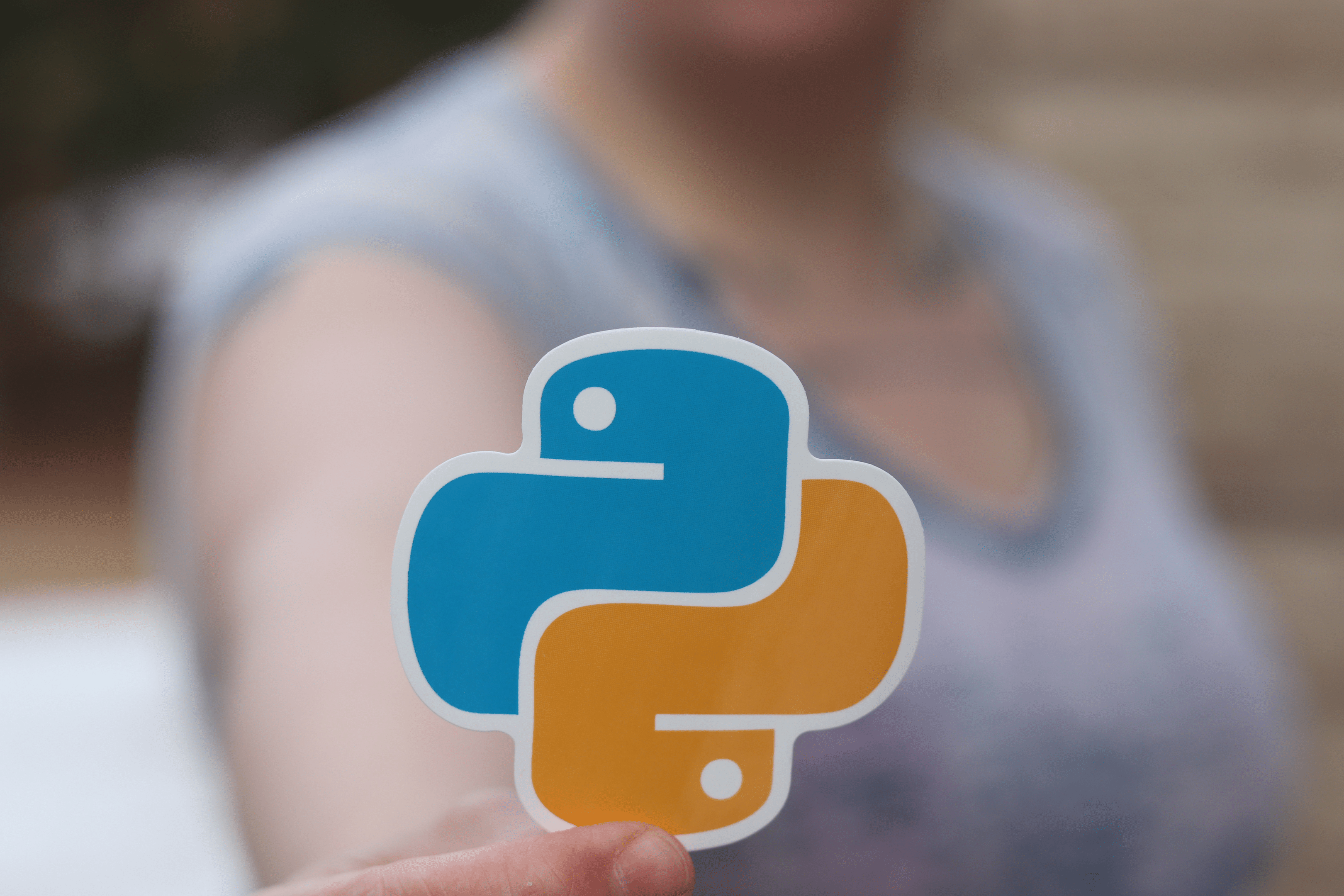
If you’re learning Python, understanding the concept of None in Python is important. It provides you with the means to create efficient code that handles edge cases. As you explore Python programming, you’ll come across various scenarios where None proves to be essential.
In this article, we’ll explore what a null, or none, is in Python. We’ll go over examples and use cases to help you solidify the concept of null values. But first, let’s understand the concept of None, or Null, values in Python.
What is Null in Python?
In order to understand null in Python, you’ll have to understand the following two concepts:
Difference between None and Null
Nonetype
1. Difference Between None and Null
Null in many programming languages is used to denote an empty pointer or an unknown value. You can define the null value as 0 in these languages.
However, in Python, instead of null, you use the keyword None to define empty objects and variables. None serves some of the same purposes as null in other languages. But it is a distinct entity and not defined to be 0 or any other value.
2. NoneType
In Python, None is a unique kind of data structure, classified under a type called NoneType. It’s a first-class citizen, meaning you can assign it to variables. You can also pass it as an argument and return as a result.
When you assign a variable value None, it becomes a NoneType object. None is not equivalent to an empty string, the Boolean value False, or the numerical value 0. It simply means null variable or nothing.
Now that you understand the terminologies related to none, let’s go ahead and see how you can use None for different use cases!
How to Use None in Python
In Python, you can use None for a number of use cases. In this section, we’ll look at 4 important use cases of None in Python.
Specifically, we’ll look at the following use cases:
Assigning None to variables
Using None as default parameter
Checking for None with identity operator
Handling missing values
Let’s get into it!
1. Assigning None to Variables
You can assign None to variables to indicate the absence of a value.
For example:
x = None
None here means that the value of x is absent or a valid value has not been decided yet.
2. Using None as Default Parameter
You can use None as a default parameter value to define functions as well. This is useful when you want to allow optional arguments for default parameters in your functions.
The following code shows you how to use None as a default parameter:
def greet(name=None):
if name is None:
print("Hello, World!")
else:
print(f"Hello, {name}!")
# Call the function without providing a name
greet()
# Call the function with a name
greet("Alice")
When you run this code, it prints “Hello, World!” when you call greet() without any argument. But when you call greet() with an argument, like greet(“Alice”), it prints “Hello, Alice!”. This allows the function to have optional arguments.
3. Checking for None With Identity Operator
You can use the is identity operator to check if a variable is None. This allows you to compare if two variables refer to the same object.
The following is an example to show you how to use the identity operator:
# Define a variable with no value
var1 = None
# Define a variable with a value
var2 = "Hello, World!"
# Check if var1 is None
if var1 is None:
print("var1 is None")
else:
print("var1 is not None")
# Check if var2 is None
if var2 is None:
print("var2 is None")
else:
print("var2 is not None")
In this code, var1 is None will return True because var1 was assigned the value None. On the other hand, var2 is None will return False because var2 has a string value.
The output for the script is given below:
4. Handling Missing Values
You may also encounter data with missing values. You can represent these missing values with None and handle them accordingly.
The following is an example when dealing with a list of numbers:
# Define a list of data with some missing values
data = [1, 2, 3, None, 5, None, 7, 8, None, 10]
def calculate_average(data):
# Filter out None values using list comprehension
valid_data = [value for value in data if value is not None]
# Calculate average if valid_data is not empty
average = sum(valid_data) / len(valid_data) if valid_data else None
return average
# Call the function and print the result
print(calculate_average(data))
This function calculates the sum of all non-None values in the list and divides it by the count of non-None values. If all the values in the list are None, the function returns None.
The output is given below:
By using None and the different techniques mentioned above, you can effectively handle missing values in Python.
To learn more about handling missing values in Python, check the following video out:
Some More Use Cases of None in Python
Apart from the use cases discussed in the section above, there are some other use cases of None as well. These use cases are not frequently used ones but are good to know and are useful in some scenarios.
In this section, we’ll look go over the following 3 use cases of None in Python:
Using None in return statements
Using None as flags
Using None for custom objects
Let’s dive into it!
1. Using None as Return Statements
You can use None in return statements, particularly when a function completes its execution without finding a specific result.
For example, a function that searches for an element in a list can return None if the element is not found. The following code demonstrates using None as return value:
# Define a list
numbers = [10, 20, 30, 40, 50]
def find_in_list(lst, target):
for i, value in enumerate(lst):
if value == target:
return i
return None
# Search for a number in the list
print(find_in_list(numbers, 30))
print(find_in_list(numbers, 60))
In this code, the find_in_list function iterates over the input list lst. If it finds the target value, it returns the index of that value. If it doesn’t find the target value after checking the entire list, it returns None.
The output is given below:
2. Using None as Flags
You can also use None as a flag to indicate the state of a variable. For instance, you might use it to mark whether a value is uninitialized or to track a special condition in the program.
The following is an example of how you can use None as a flag:
def find_first_even_number(numbers):
# Initialize the first_even_number to None
first_even_number = None
# Iterate over the list of numbers
for number in numbers:
# If we find an even number and first_even_number is still None
if number % 2 == 0 and first_even_number is None:
first_even_number = number
break
return first_even_number
numbers = [1, 3, 5, 7, 8, 10]
# Call the function and print the result
print(find_first_even_number(numbers)) # Outputs: 8
In this code, None is used as a flag to indicate that we haven’t found an even number in the list yet.
If first_even_number is still None after the loop, this means that there were no even numbers in the list.
If first_even_number is not None, it contains the first even number from the list.
3. Using None for Custom Objects
You can use None for custom, user-defined objects, as well. When dealing with attributes, properties, or methods that return other objects, you might use None to signify the absence of a value or a relationship.
The following example demonstrates how you can use None for user-defined objects:
class Person:
def __init__(self, name):
self.name = name
self.best_friend = None
def set_best_friend(self, person):
self.best_friend = person
def get_best_friend_name(self):
if self.best_friend is not None:
return self.best_friend.name
else:
return None
# Create two Person objects
alice = Person('Alice')
bob = Person('Bob')
# Alice's best friend is Bob
alice.set_best_friend(bob)
print(alice.get_best_friend_name())
# Charlie doesn't have a best friend
charlie = Person('Charlie')
print(charlie.get_best_friend_name())
In this example, None is used as a default value for the best_friend attribute of a Person instance. The get_best_friend_name method returns the name of the best friend if there is one, and None if there isn’t.
The above code will output the following:
Special Functions and Techniques for Null Object
We’ll now discuss some special functions and techniques that you can use related to NoneType and handling null values. We’ll cover two main sub-sections:
The Id Function
The Getattr Function
1. Id Function
The id() function in Python is a built-in function that returns the identity of an object. This identity is a unique and constant integer assigned to an object during its lifetime.
This allows you to distinguish between objects with different memory addresses. The function is especially useful when working with NoneType objects, as it can be used to check for the presence of None in variables or expressions.
The following is an example using the id() function:
a = None
b = None
c = 42
print(id(a)) # Output: some unique integer representing None
print(id(b)) # Output: same integer as id(a)
print(id(c)) # Output: different integer from id(a) and id(b)
The output of this code is given below:
Since None is a first-class citizen in Python, it has its own unique identity. This means you can use the id() function to compare variables to None using their identities:
if id(a) == id(None):
print("a is None")
The output is given below:
2. Getattr Function
The getattr() function is another built-in Python function that allows you to access an object’s attributes by name.
Given an object and a string, getattr() returns the value of the named attribute or the None object if the attribute doesn’t exist.
This can be helpful for you when handling null values, especially in cases where you need to access attributes that might be missing.
The following is an example using the getattr() function:
class ExampleClass:
def __init__(self, x, y):
self.x = x
self.y = y
example_obj = ExampleClass(3, 4)
# Access attribute using getattr
print(getattr(example_obj, "x")) # Output: 3
print(getattr(example_obj, "z", None)) # Output: None
In the example above, we use getattr() to access the x attribute and attempt to access a non-existent z attribute.
By providing a default value of None, we prevent an AttributeError and instead receive the None object.
As a result, this function allows for safe attribute access even in the presence of null objects or missing attributes.
The output of this code is given below:
Comparing Null in Other Programming Languages
At the start of this article, we made a short comparison of Null in Python and Null in other programming languages.
In this section, we’ll take this topic forward and compare Null in other popular programming languages with Null in Python. This will help you better understand the concept of None in Python if you are coming from a different programming background.
Let’s get into it!
1. Null in Java
In Java, the “null” keyword represents a reference that does not point to any object or value. It is used to indicate the absence of a value and is often used as the default value for object references.
For instance:
String myString = null; // Declare a String object with a null reference
Java’s null is different from Python’s None. Null represents a lack of an object, whereas None is an actual object of NoneType.
2. Null in C
In the C programming language, null is represented by a null pointer, which indicates an uninitialized or empty pointer. The null pointer is often represented by the integer value 0.
The following is an example of using a null pointer in C:
int *myPointer = NULL; // Declare an integer pointer with a null reference
Null in C is not associated with a specific data type. It serves as a placeholder for uninitialized or empty pointers.
3. Null in PHP
In PHP, null represents a variable with no value or no reference. Like in other languages, null is used to denote uninitialized or empty variables.
The following is an example of Null in PHP:
$myVar = NULL; // Declare a variable with a null reference
PHP’s null is not an object of a specific data type but rather a special constant that represents the absence of a value.
Final Thoughts
In your journey as a Python programmer, learning about None values is of high importance. It’s a unique feature that serves as Python’s way of saying “nothing here.”
It’s essential for representing the absence of a value. By understanding and correctly using None, you’re able to create more flexible functions with optional arguments and effectively handle missing data.
Furthermore, None values allow your functions to communicate clearly when something is absent or when a condition hasn’t been met. Moreover, understanding None is crucial when dealing with databases, user-defined objects, or when you need to initialize variables.
This guide on None values in Python demonstrates some of the important scenarios where you’ll want to use None object in your code.