One common operation when working with data in Python is subtracting two lists. You will often need to carry out element-wise subtraction between two lists to derive meaningful results or insights. There are many approaches to subtract two lists in Python, each with its own advantages and use cases.
To subtract two lists in Python, you can use Python’s numerical library, NumPy. It comes with a subtract() function which makes subtracting two lists easy. Furthermore, you can also use Python’s built-in list comprehension and zip() functions to achieve the same outcome.
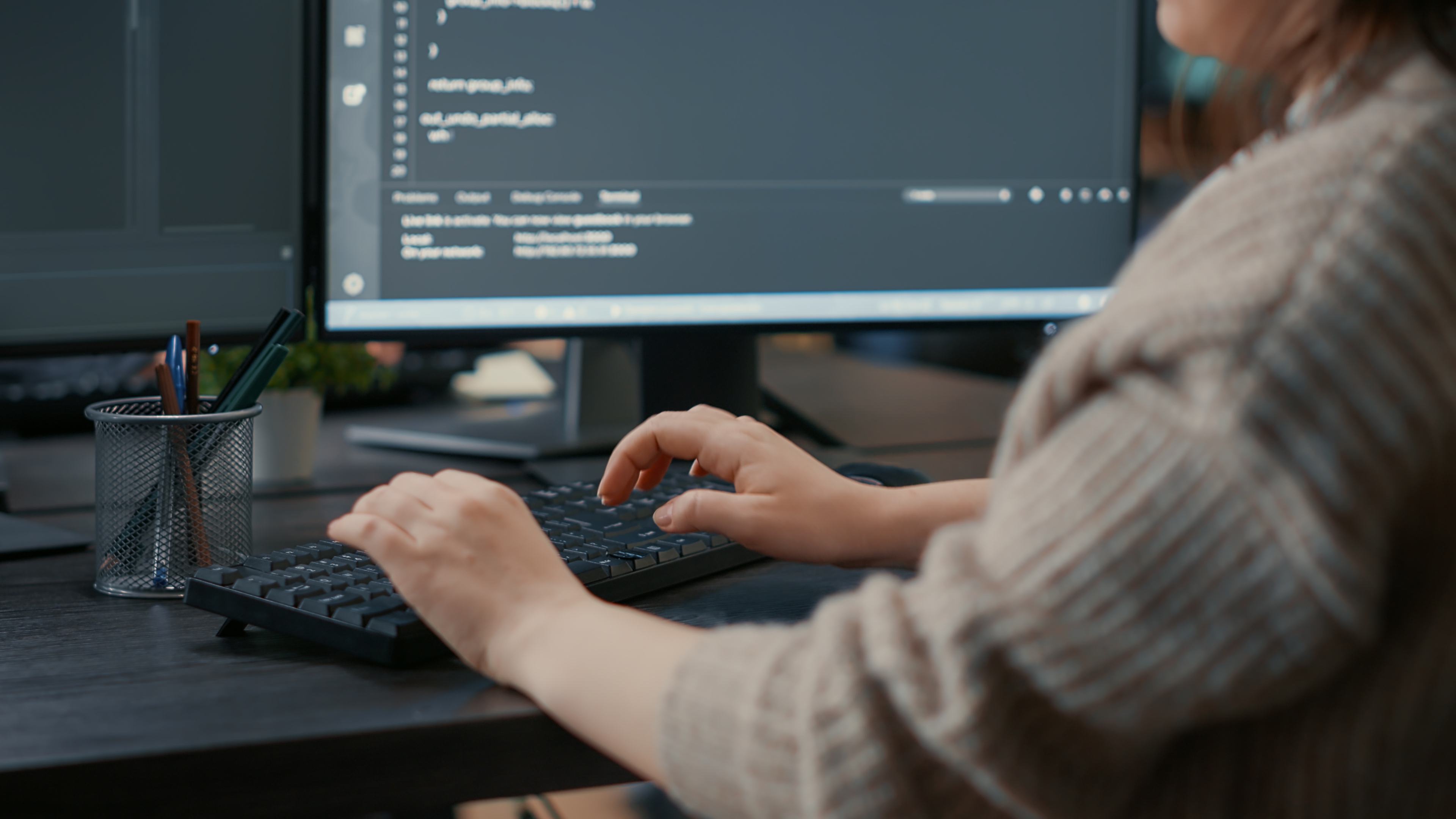
When you are choosing a method for list subtraction in Python, you must consider factors such as the size of the data, desired level of performance, and requirements related to the order of list elements.
In this article, we’ll go explore ways to subtract two lists in Python. We’ll also look at specific examples to help you better understand the concepts.
Let’s get into it!
Understanding the Concept of Subtracting Lists in Python
Subtracting lists in Python is a straightforward operation, and there are multiple ways you can achieve this task.
However, it’s very important that you understand the underlying reasons that allow us to subtract one list from another. This will help perform more efficient calculations and reason when errors occur in your code.
In this section, we’ll cover the following:
- What are Python Lists
- Are lists mutable?
- What is element-wise list subtraction?
1. What Are Python Lists?
A list is a mutable, ordered data structure in Python that can hold a collection of items. Lists are commonly used to store and manipulate data.
Subtraction of lists can refer to one of two operations:
- Removing elements from one list that are present in another list.
- Performing element-wise subtraction, where corresponding elements in two lists are subtracted from each other.
In this section, we will discuss how to perform both operations and their use cases.
2. Are Lists Mutable?
Lists are mutable data types, which means you can update their elements at runtime. This characteristic allows Python lists to accommodate various list operations. These include appending, extending, slicing, and element-wise subtraction.
To perform some of these operations, you will require the use of external libraries or custom functions to achieve the desired outcome.
For example, subtracting two lists element-wise is not supported in Python. However, you can do it by using external libraries like NumPy or by implementing custom-built functions
3. What is Element-Wise List Subtraction?
Element-wise subtraction refers to subtracting the elements of two lists at the same index positions. This operation requires both lists to be of the same length to have a one-to-one correspondence between their elements.
When the length is not equal, this operation will result in either an error or an unexpected outcome.
To perform element-wise subtraction, you can use list comprehensions, the zip() function, or external libraries such as NumPy.
What Are the Different Approaches for Subtracting Lists in Python?
You can use several methods to subtract two lists in Python.
This section will cover the most common approaches, which include:
- Using for loop method and Range Functions
- Applying List Comprehension
- Employing Lambda Function and the map() Function
- Exploiting Sets and Set Difference Operations
1. Using ‘For’ Loop and Range Functions for List Subtraction
You can subtract two list elements using for loop and range(), which is a built-in function. This approach is ideal for element-wise subtraction in lists of equal length.
The following example shows you how to perform the subtraction:
list1 = [5, 8, 12, 16]
list2 = [3, 6, 9, 11]
result = []
for i in range(len(list1)):
result.append(list1[i] - list2[i])
print(result)
In this code, we are subtracting corresponding elements in two lists. For each index in the range of the length of list1, it subtracts the value at that index in list2 from the value at the same index in list1. It then appends the result to the result list.
The output of this code is given below:
2. Using List Comprehensions for List Subtraction
List comprehension is a concise and efficient way to subtract two lists in Python. You can use this approach when the input lists are of equal length.
The following code shows you how to perform this operation:
list1 = [7, 10, 14, 18]
list2 = [3, 8, 9, 15]
result = [a - b for a, b in zip(list1, list2)]
print(result)
This Python code is performing element-wise subtraction between two lists using list comprehension and the zip function. The zip function pairs up the corresponding elements from list1 and list2.
The list comprehension then subtracts each pair of elements (represented as a and b), creating a new list result with these differences.
The output is given below:
3. Using Lambda and map() Functions for List Subtraction
Another function you can use is the Lambda functions to subtract two lists. By using the map() object, you can apply the lambda function to the corresponding elements of the input lists.
This method is suitable for lists of equal length.
The following is an example of using the Lambda and map() functions for list subtraction:
list1 = [4, 7, 11, 15]
list2 = [2, 5, 6, 12]
result = list(map(lambda a, b: a - b, list1, list2))
print(result)
This Python code performs element-wise subtraction between two lists using the map and lambda functions.
The map function applies the lambda function, which subtracts its two arguments, to each pair of corresponding elements from list1 and list2. This creates a new list result of the differences.
4. Using Sets and Set Difference Operations for List Subtraction
You can use sets and the set difference operation when the order of elements in the lists does not matter, and lists contain unique elements.
To do this, you convert both lists to set() objects and apply the built-in set difference method to find the resulting list.
The following example shows you how to use sets and set difference operations:
list1 = [8, 11, 15, 19]
list2 = [3, 11, 9, 15]
set1 = set(list1)
set2 = set(list2)
result = set1 - set2
print(result)
This Python code uses sets to perform a “subtraction” operation between two lists. First, it converts list1 and list2 into sets, set1 and set2. It then performs the difference operation (set1 – set2), which results in a new set, result. It consists of elements that are in set1 but not in set2.
How to Use Numpy Arrays for List Subtraction in Python
NumPy is a powerful library for numerical computing in Python. It is widely used for tasks such as data analysis, machine learning, and scientific research.
The NumPy library is designed to work with large arrays of data. It provides you with a wide range of functions for performing mathematical operations on arrays.
In this section, we’ll first go over NumPy arrays, data types, and array objects. Then we’ll look at how you can use NumPy to perform list subtraction.
1. Understanding NumPy Arrays, Data Types, and Array Objects
A NumPy array is a grid of values that are all of the same types. It is indexed by non-negative integers.
Arrays are fundamental to NumPy and you can easily create and manipulate them using various functions provided by the library. Arrays can be one-dimensional or multi-dimensional. NumPy arrays have a fixed size and allow only one data type per array.
Some of the built-in data types supported by NumPy include:
- int8, int16, int32, int64: Signed integers
- uint8, uint16, uint32, uint64: Unsigned integers
- float16, float32, float64: Floating-point numbers
- complex64, complex128: Complex numbers
The array object in NumPy is a powerful and flexible container for storing, slicing, and performing element-wise operations on data. NumPy’s array object is very similar to Python lists but offers better performance and more functionality.
2. How to Perform Array Operations Using np.subtract()
To subtract two lists in Python using NumPy, you can use the np.subtract() function or the – operator.
The following is an example of how to use the np.subtract() method to perform element-wise subtraction between two lists:
import numpy as np
list1 = [3, 5, 6]
list2 = [3, 7, 2]
array1 = np.array(list1)
array2 = np.array(list2)
result = np.subtract(array1, array2)
result_list = result.tolist()
print(result_list)
This Python code performs element-wise subtraction between two existing lists using NumPy’s subtract function. It first converts list1 and list2 into NumPy arrays array1 and array2.
Then, np.subtract(array1, array2) function returns the difference between corresponding elements in the two arrays. This creates a new NumPy array result which is then converted back to a Python list result_list.
The output of the code is given below:
You can also use the – operator to achieve the same result:
import numpy as np
list1 = [3, 5, 6]
list2 = [3, 7, 2]
array1 = np.array(list1)
array2 = np.array(list2)
result = array1 - array2
result_list = result.tolist()
print(result_list)
The output is the same as the above code:
In both cases above, we create the NumPy arrays using the np.array() method, and then we perform element-wise subtraction. The result is then converted back to a Python list using the tolist() method.
To learn more about transformation in Python, check the following video out:
Final Thoughts
In conclusion, mastering list subtraction is a vital skill in your Python toolkit. As a programmer, especially if you work in data science, you’ll often find yourself needing to compare two lists, remove duplicates, delete an empty list, or filter data.
This is where list subtraction becomes extremely important. By learning this technique, you can manipulate data more efficiently and with greater precision.
This skill adds value to your coding by equipping you with an important tool to manage and manipulate data. It streamlines your code and makes it more readable and maintainable.