When working with numerical data, an important and frequently used programming operation is to sum the numbers. Python comes with a built-in sum() function that simplifies the process of summing an individual or a series of elements.
The Python sum() function is a built-in function that returns the sum of all elements in an iterable (like a list or a tuple) and an optional start value. If the iterable contains numerical values, it calculates the sum of these values. If the optional start value is provided, the function adds this value to the sum.
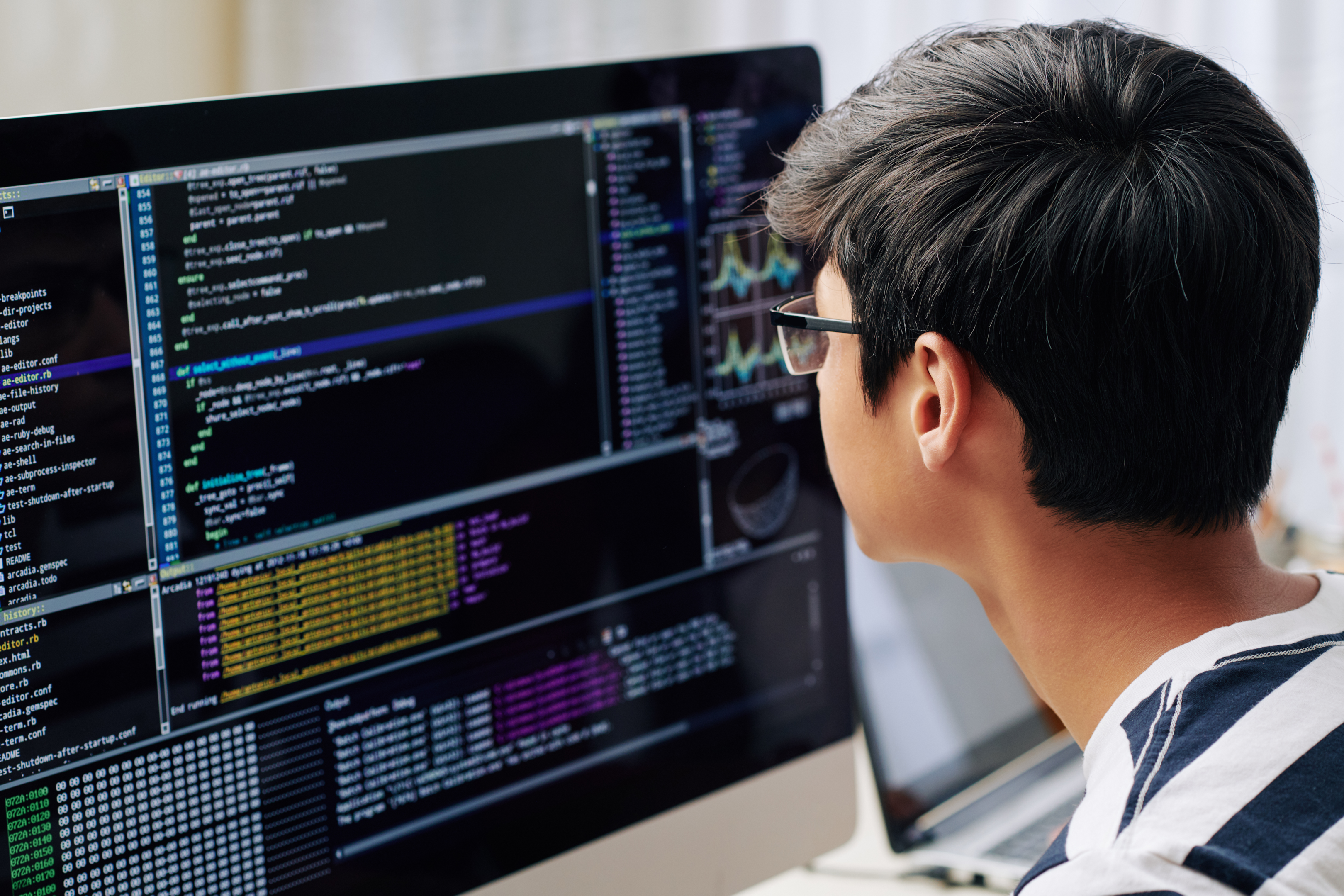
The sum() function makes it easy and efficient to compute the cumulative total of a sequence of numbers in Python. It is useful in diverse computational tasks, such as data analysis, numerical modeling, and problem-solving.
In this article, we will explore the Python sum() function and how you can use it to sum the contents of a list. There will be examples to help you better understand the concepts.
Understanding the Basics of Sum List
Before we dive into writing the code for the sum list, it is crucial that you understand the basics of Python lists and the sum() function.
In this section, we will have a quick look at:
- Python Lists
- Built-in sum() Function
1) What is a Python List
Python lists are powerful data structures that can store a collection of items.
These items can be of any data type, such as numbers, strings, or other objects.
They are mutable, meaning that the items within a list can be changed, and their order can be rearranged.
The following is an example of a simple list in Python:
my_list = [1, 2, 3, 4, 5]
2) What is sum() Function
Python offers a built-in sum() function that can add all the elements in a list of numbers. The function takes an iterable, typically a list, as its first argument and an optional start value as its second argument.
The syntax of a sum() function is given below:
#syntax
sum(iterable, start)
iterable (required): This is a sequence (list, tuple, etc.) or collection (set, dictionary, etc.) or an iterator object to be summed up.
start (optional): This value is added to the sum of items in the iterable. It’s default value is 0.
How to Sum a List in Python
To sum a list in Python, you can pass the list as an argument to the sum() function, and it will return the sum of the list’s contents.
Below, you’ll find three examples of the sum() function:
Example 1: Sum of numbers in a list
To sum the numbers in a list in Python from the initial value to the final, you can use the following code:
numbers = [10, 20, 30, 40, 50]
total = sum(numbers)
print(total)
In this example, sum() function is used to add all numbers in the list. The output will be 150 as the total of all numbers in the list is 150.
Example 2: Sum of Numbers in a List With a Start Value
To sum numbers in a list with a start as input values, you can use the following code:
numbers = [5, 10, 15, 20]
start_value = 100
total = sum(numbers, start_value)
print(total)
In this example, we have used a start value of 100. The sum() function will add this start value to the sum of numbers in the list.
The output sum will be 150 as the total of all elements in the list plus the start value is 150.
Example 3: Sum of a List of Floating Point Numbers
To sum a list of floating point numbers, you can use the following code:
numbers = [1.2, 2.3, 3.4, 4.5]
total = sum(numbers)
#print sum of list
print(total)
In this example, the sum() function is used to add all floating point numbers in the list.
The final value will be 11.4 as the total of all numbers in the list is 11.4. Note that sum() function can work with both integers and floating-point numbers.
4 Alternatives of The Python sum() Function
When finding the sum of the elements of a list, the go-to function of every Python programmer is the sum() function.
At times, depending on the use case, you might want to opt for an alternative way of summing up a Python list. In this section, we will go over 4 ways to sum a Python list.
- Using For Loop or While Loop
- Using reduce() Function From functools Module
- Using NumPy’s sum() Function
- Using Pandas sum() Function
1) How to Sum a List Using For Loops
You can use a simple for loop to iterate over the elements of the iterable and add them up.
The following Python code shows how you can sum a list using a for loop:
numbers = [1, 2, 3, 4, 5]
total = 0
for num in numbers:
total += num
print(total)
In the above example, we iterate over each element of the list and add it to the total variable.
The final sum in shown in the image below:
2) How to Sum List Using reduce() Function
The reduce() function applies a binary function (a function that takes two arguments) to the elements of an iterable in a cumulative way.
For adding up numbers, you can use the addition operator as shown below:
from functools import reduce
import operator
numbers = [1, 2, 3, 4, 5]
#reduce function called
total = reduce(operator.add, numbers)
print(total)
The output sum of the list elements will be:
3) How to Sum List Using NumPy’s sum() Function
If you’re working with numerical data, the NumPy library offers its own sum() function, which can be faster than Python’s built-in sum() for large arrays.
The following is an example of using the NumPy’s sum() function:
import numpy as np
numbers = np.array([1, 2, 3, 4, 5])
total = np.sum(numbers)
print(total)
The output will be:
4) How to Sum List Using Pandas sum() Function
To sum a list using Pandas sum() function, you first need to convert the list into a Pandas Series or DataFrame.
The following code example demonstrates this method:
import pandas as pd
# Create a list of numbers
numbers = [1, 2, 3, 4, 5]
# Convert the list to a Pandas Series
numbers_series = pd.Series(numbers)
# Use the sum() function to add up the numbers in the series
total = numbers_series.sum()
# Print the result
print(total)
In this example, the pd.Series() function is used to convert the list numbers into a Pandas Series. Then, the sum() function is called on the series to calculate the sum of the numbers. The result is 15, which is the sum of the numbers in the original list.
How to Sum a Nested List
A nested list is a list within another list. In this section, we will discuss different methods to sum a nested list in Python.
Specifically, we will go over the following:
- Using Nested For Loop
- Using sum() Function With List Comprehension
- Using NumPy’s sum() Function
- Using itertools.chain
- Using functools and operator
1) How to Sum Nested List Using Nested For Loop
The most straightforward way to sum a nested list is with a nested for loop where the outer loop goes through each sublist, and the inner loop adds up its elements.
The following is an example of this method:
numbers = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
total = 0
for sublist in numbers:
for num in sublist:
total += num
print(total)
The output will be:
2) How to Sum a Nested List Using sum() Function With List Comprehension
You can use Python’s built-in sum() function along with a list comprehension to sum all the numbers in a nested list.
The following is an example of this method:
numbers = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
total = sum(num for sublist in numbers for num in sublist)
print(total)
The output will be:
3) How to Sum Nested List Using NumPy’s sum() Function
NumPy provides the sum() function which can sum a nested list in a much simpler way.
It treats the nested lists as a 2D array.
The following is an example of those method:
import numpy as np
numbers = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
total = np.sum(numbers)
print(total)
The output will be:
4) How to Sum Nested List Using itertools.chain
You can use the itertools.chain function to flatten the list, which can then be summed up using the built-in sum() function.
The following code demonstrates this method:
import itertools
numbers = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
total = sum(itertools.chain.from_iterable(numbers))
print(total)
The output will be:
5) How to Sum Nested List Using Functools and Operator
The functools.reduce() function along with operator.add or generator expression can be used to flatten the list and then sum it up.
The following is an example of this method:
import functools
import operator
numbers = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
total = sum(functools.reduce(operator.concat, numbers))
print(total)
The output will be:
Supercharge your analytics potential with Code Interpreter for data analysis by watching the following video:
Final Thoughts
Mastering the various ways to sum a list in Python is an invaluable skill that can significantly streamline your code. By learning and implementing the Python sum() function and its alternatives, you are tapping into the heart of simplicity and readability.
Understanding these concepts equips you to effectively deal with large data sets, nested lists, and complex data structures with ease.
These methods prepares you for more advanced data manipulation techniques, critical for areas like data analysis, machine learning, and more.
Frequently Asked Questions
In this section, you’ll find some frequently asked questions you may have when summing lists in Python.
How to sum elements in a list using a for loop?
To sum elements in a list using a for loop, initialize a variable to store the sum and then iterate through the list, adding each element to the sum.
my_list = [1, 2, 3, 4, 5]
total = 0
for element in my_list:
total += element
print(total) # Output: 15
What is the sum() function in Pandas?
In Pandas, the sum() function is used to calculate the sum of values in a Series or DataFrame along a specified axis.
For example:
import pandas as pd
data = {'A': [1, 2, 3], 'B': [4, 5, 6]}
df = pd.DataFrame(data)
total = df.sum(axis=0) # Output: A 6
# B 15
# dtype: int64
How to use lambda to sum a list in Python?
You can use the reduce() function from the functools module along with a lambda function to sum a list in Python.
from functools import reduce
my_list = [1, 2, 3, 4, 5]
total = reduce(lambda x, y: x + y, my_list)
print(total) # Output: 15
How do you find the sum of an array in Python?
To find the sum of an array in Python, you can use the built-in sum() function, which works with lists, tuples, or arrays.
import numpy as np
my_array = np.array([1, 2, 3, 4, 5])
total = sum(my_array)
print(total) # Output: 15
How to calculate the average of a list?
To calculate the average of a list, you can use the built-in sum() function and divide the sum by the length of the list.
my_list = [1, 2, 3, 4, 5]
average = sum(my_list) / len(my_list)
print(average) # Output: 3.0
How to sum two lists element-wise in Python?
To sum two lists element-wise in Python, you can use the zip() function along with a list comprehension.
list1 = [1, 2, 3]
list2 = [4, 5, 6]
sum_list = [x + y for x, y in zip(list1, list2)]
print(sum_list) # Output: [5, 7, 9]