Lists are one of the most frequently used data structures in Python. This makes list operations crucial for working with data.
One frequent operation is to determine whether a list is empty or not. Knowing if a list is empty is crucial for proper control flow and error handling within your code.
To check if a list is empty, you can use the bool() function. When evaluated in a boolean context, an empty list returns False, while a non-empty list returns True. You can also use the len() function, if statement and filter functions to check if a list is empty in Python.
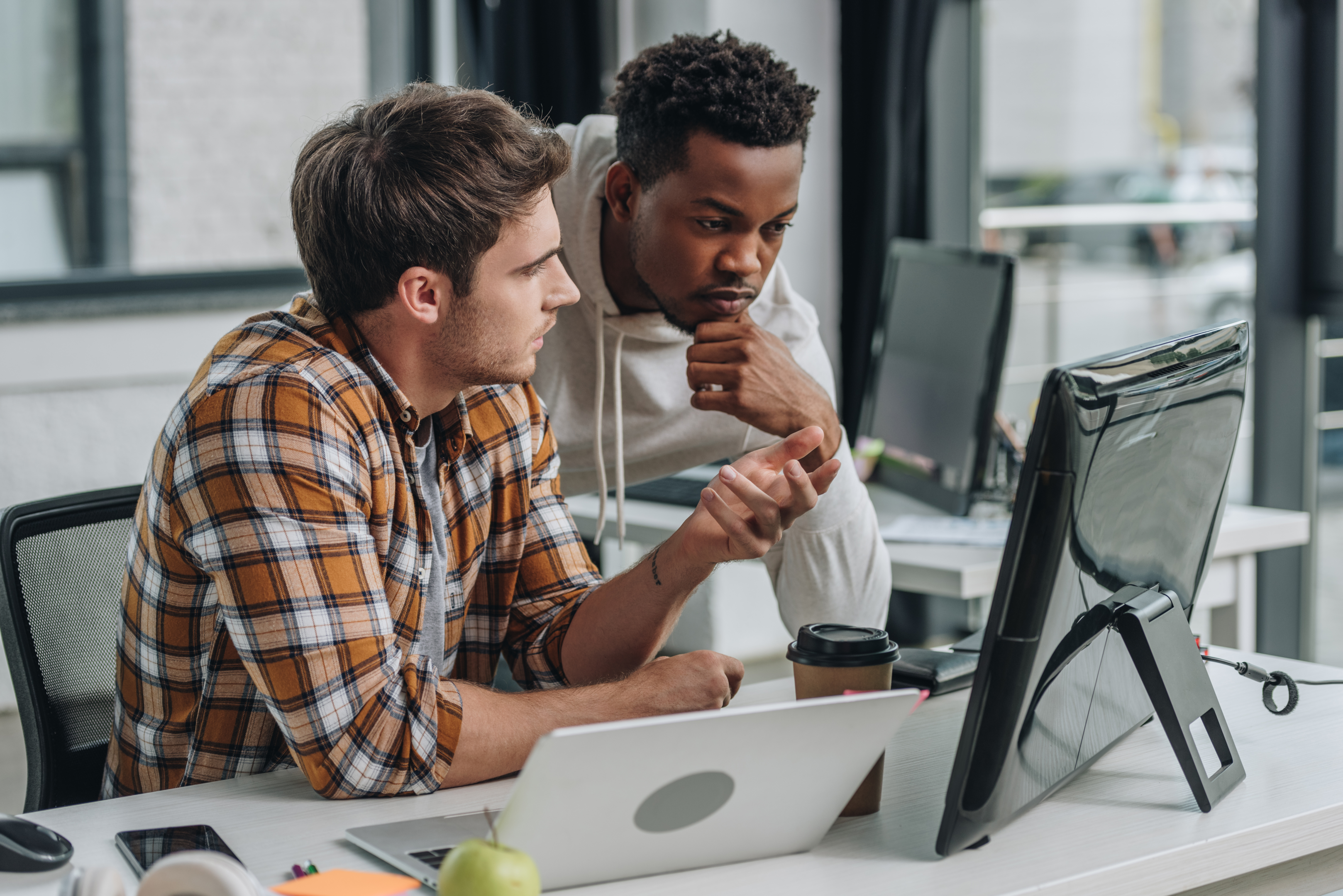
This article will explore these techniques for determining if a list is empty in Python.
By the end of the article, you will have a clear understanding of the best approach for your specific use case. This will allow you to write more efficient and specified code.
Let’s get into it!
7 Ways to Check if a List is Empty
In this section, we will explore 5 ways to check if a list is empty in Python.
Specifically, we will go over the following methods:
- Using the bool() function
- Using the len() function
- Using a try-except block
- Using the filter() function
- Using set comparison
- Using a custom function
- Using Numpy Arrays
1) How to Use The bool() Function to Check if a List is Empty
You can use the bool() function in Python to return the boolean value of a specified object.
The bool() function allows you to evaluate any value, and for many standard Python structures, including lists, it returns False if they are empty and True otherwise.
To use the bool() function to check if a Python list is empty, you can pass the list as an argument to the bool() function.
The following is an example of this method:
my_list = []
# Using bool() function to check emptiness
if not bool(my_list):
print("The list is empty.")
# else block
else:
print("The list is not empty.")
The following output will be displayed in the console:
2) How to Use the len() Function to Check if a List is Empty
The len() function in Python returns the number of items in an object.
When applied to Python lists, it returns the number of elements present in that list. If the number of elements is 0, that would indicate that the list is empty.
To use the len() function to check if a list is empty, you can pass the list as an argument to the len() function as shown below:
my_list = []
# Using len() function to check emptiness
if len(my_list) == 0:
print("The list is empty.")
else:
print("The list is not empty.")
In this example, the output will be “The list is empty.” because my_list doesn’t contain any elements.
3) How to Use Try-Except Block to Check if a List is Empty
Using a try-except block to check if a list is empty is not a common or recommended method. It is useful in scenarios where you might be unsure about the variable’s existence or its type.
The following is an example of this method:
try:
if not my_list:
print("The list is empty.")
else:
print("The list is not empty.")
# catches any unwanted errors
except NameError:
print("The variable 'my_list' is not defined.")
In the above example, if my_list is not defined or if it’s empty, the corresponding messages will be printed.
4) How to Use The filter() Function to Check if a List is Empty
The filter() function in Python is primarily used to filter the values in an iterable based on a function that returns True or False.
To check if a list is empty using filter(), you can filter the list based on the identity function and then convert the result to a list to determine its length or truthiness. This is also known as truth value testing.
The following is an example of this method:
my_list = [0, False, "", None] # A list with falsy values
# Using filter() function to check for emptiness based on truthiness
filtered_list = list(filter(lambda x: x, my_list))
if not filtered_list:
print("The list is empty or contains only falsy values.")
else:
print("The list is not empty.")
In this example, we have some constants defined in my_list. The output will be “The list is empty or contains only falsy values.”, because while my_list does have elements, all of them are considered false values.
5) How to Use Set Comparison to Check if a List is Empty
Using set comparison operation to check if a list is empty is an unconventional method, but it’s still doable.
The core idea behind this method is to compare the set representation of the list to an empty set. Since a list can be converted to a set, an empty list would translate to an empty set.
The following is an example of this method:
my_list = []
# Using set comparison to check for emptiness
if set(my_list) == set():
print("The list is empty.")
else:
print("The list is not empty.")
In this example, the output will be “The list is empty.” because my_list does not contain any elements.
However, it’s worth noting that this approach is less efficient and less intuitive than the direct methods for checking list emptiness.
6) How to Use a Custom Function to Check if a List is Empty
Creating a custom function to check if a list is empty can provide clarity, especially if you aim to use the function multiple times in your code or if you want to abstract away certain checks.
The custom function encapsulates the logic required to check the emptiness of the list.
The following is an example of this method:
def is_empty(lst):
"""Return True if the list is empty, otherwise return False."""
return not lst
my_list = []
# Using the custom function to check for emptiness
if is_empty(my_list):
print("The list is empty.")
else:
print("The list is not empty.")
In this example, the custom function is_empty checks the truthiness of the list and returns the result.
The output will be “The list is empty.” because my_list doesn’t have any elements.
7) How to Check if a List is Empty in Numpy
In the context of numpy, an “empty” list typically refers to an array with no elements.
While you can still use many of the Python-native methods to check if a numpy array is empty, numpy offers a more efficient and idiomatic way to handle this.
You can use the size attribute of the numpy array, which returns the number of elements in the array. If the size is 0, the array is empty.
The following is an example of this method:
import numpy as np
arr = np.array([]) # An empty numpy array
if arr.size == 0:
print("The numpy array is empty.")
else:
print("The numpy array is not empty.")
In the above code, the output will be “The numpy array is empty.” because arr doesn’t contain any elements.
Learn more about the important Python functions by watching the following video:
Final Thoughts
Understanding how to check if a list is empty in Python is an essential skill that paves the way for robust and error-free programs.
When you handle lists, which are among the most ubiquitous data structures in Python, ensuring their content can prevent unexpected behaviors or bugs in your applications.
Learning this skill signifies that you have a strong grasp of the fundamentals, which always pays off in programming.
Moreover, by efficiently checking list emptiness, you not only optimize your code but also make it more readable for others.
Frequently Asked Questions
In this section, you’ll find some frequently asked questions you may have when checking if a list is empty in Python.
How do you determine if a list is empty in Python?
To determine if a list is empty in Python, you can use the not operator.
This method takes advantage of the implicit boolean value of an empty list. When you use not with an empty list, it returns True.
For example:
my_list = []
if not my_list:
print("List is empty")
What is the best way to check if a list has elements?
The best way to check if a list has elements is to use the len() function.
This function returns the length of the list, and you can then evaluate if the length is greater than zero:
my_list = [1, 2, 3]
if len(my_list) > 0:
print("List has elements")
How can you verify if a list contains any items?
You can verify if a list contains any items by using the bool() built-in objects function.
This converts the list into a boolean value, which is True when the list has elements and False when the list is empty:
my_list = [1, 2, 3]
if bool(my_list):
print("List contains items")
What is the preferred method to test for an empty list?
The preferred method to test for an empty list is to use the not operator, as it exploits the implicit boolean value of an empty list and is considered more Pythonic:
my_list = []
if not my_list:
print("List is empty")
How can you assert that a list is not empty in Python?
To assert that a list is not empty in Python, you can simply check its boolean value directly in an if statement, like this:
my_list = [1, 2, 3]
if my_list:
print("List is not empty")
Is there a built-in function to check for empty lists in Python?
There isn’t a specific built-in function to check for empty lists in Python.
However, you can use the implicit boolean value of a list or the len() function to achieve this.
As shown in previous examples, you can use the not operator, the bool() function, or the len() function to check if a list is empty.