The concept of absolute value is an important mathematical operation. It’s applicable in many scenarios and calculations. In Python, absolute value refers to the non-negative value of a number, regardless of its original sign.
This operation becomes relevant when dealing with quantities or measurements where only the magnitude is important and the sign is irrelevant, such as when calculating distances, errors, or differences.
To find the absolute value of a number in Python, you can use the abs() function. The native abs() function is designed to handle this operation effectively. This function accepts a single parameter, which can be an integer, a floating-point value, or even a complex number. It returns the corresponding positive value.
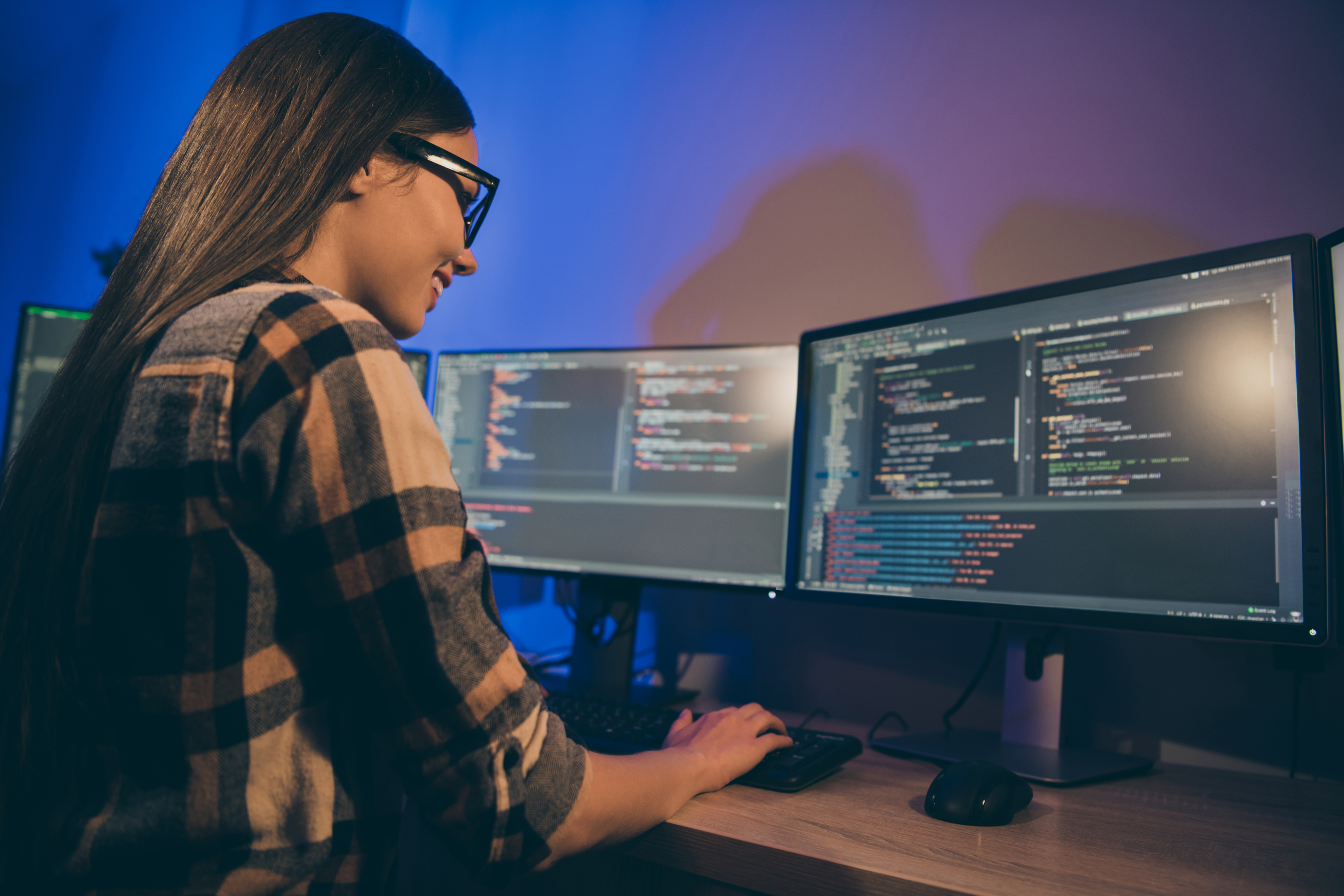
By using the applications of the abs() function, you can manage numerical computations, taking full advantage of the language’s power. Understanding how the abs() function operates helps you make more sophisticated arithmetic calculations. It also enables the customization of object behavior when necessary.
In this article, we’ll have a detailed discussion of Python’s absolute value. We’ll go over examples to help you better understand the concepts.
What is an Absolute Value?
Before we look at the programming aspect of absolute values in Python, let’s first understand the basics of absolute values. You should have a solid understanding of absolute values to be able to interpret its applications in Python.
Mathematical Definition of Absolute Value
The absolute value is an important concept that enables us to determine the magnitude or size of a number, regardless of its direction.
For real numbers, the direction can be either positive or negative number. In other words, the absolute value represents a number’s distance from zero on the number line.
It is always a non-negative value, meaning it is either zero or positive values.
Distance on Number Line
When considering the number line, the absolute value helps us understand the position of a number relative to zero.
It can be visualized as follows:
-5 -4 -3 -2 -1 0 1 2 3 4 5
<---><---><---><---><-><->
In the example above, the absolute value of -3 is 3, as its distance from zero is three units. Similarly, the absolute value of 2 is also 2, since it is two units away from zero.
4 Python Absolute Value Functions
After reviewing the basics, let’s get into the implementation of absolute values in Python. There are two ways of finding the absolute values of a function in Python.
We’ll go over the following four ways of finding absolute values in Python:
- abs() function
- math.fabs() function
- np.abs() function
- pd.abs() function
1. abs() Function
The abs() function in Python returns the absolute value of a number. The absolute value represents a number’s distance from zero on the number line. This function works with integers, floats, and complex numbers.
The syntax for the abs() function is straightforward:
abs(number)
- number: Integer, floating-point number, or complex number.
- return: Returns the absolute value of the input number.
The following are examples of calculating absolute values using the abs() function:
abs_int = abs(-5)
print(abs_int) # Output: 5
abs_float = abs(-5.7)
print(abs_float) # Output is non negative floating point number
abs_complex = abs(3+5j)
print(abs_complex) # Output: 5.830951894845301
As you can see below, the abs() function returns the absolute value for the three variables:
The output number refers to the distance between the number from 0.
2. math.fabs() Function
You can also calculate the absolute value in Python using the fabs() function from the math module.
The math.fabs() function is specifically designed for floating-point numbers. It doesn’t work with complex numbers. This function returns a float, even if you input an integer.
The syntax for the math.fabs() function is:
math.fabs(number)
- number: Integer or floating-point number.
- return: Returns the absolute value of the input number as a float.
The following are examples of math.fabs():
import math
math_fabs_int = math.fabs(-5)
print(math_fabs_int) # Output: 5.0 (notice the float return type)
math_fabs_float = math.fabs(-5.7)
print(math_fabs_float) # Output: 5.7
The output will be:
3. np.abs() Function
NumPy is a powerful library for numerical computing in Python. It offers a simple method to calculate absolute values on NumPy arrays efficiently.
The following is an example of this method:
import numpy as np
arr = np.array([-5, 0, 3.5, -10])
abs_arr = np.abs(arr)
This will calculate the absolute values element-wise for each element in the array.
4. pd.abs() Function
Pandas is a popular library for data analysis and manipulation in Python. You can use the abs() function with Pandas series and dataframes as well.
To demonstrate, let’s start by importing the library and creating a pandas series:
import pandas as pd
ser = pd.Series([-6, 2.5, 8, -3.5])
Now, you can calculate absolute values using the abs() function directly on the series:
abs_ser = ser.abs()
This will return another pandas series with the absolute values of the original series.
The same approach can be used with dataframes, applying the abs() function on individual columns.
Working with Different Numeric Types
Working with absolute values is straightforward and you can calculate it for different numeric types like integers, floats, and complex numbers.
We’ll go through each numeric type and explain how to find their absolute values.
1. How to Find Absolute Values of Integers and Floats
When dealing with integers or floats, the built-in abs() function provides a simple method to calculate the absolute value of a given number.
The absolute value represents a number’s distance from 0 on the number line, which ensures the result remains non-negative.
The following is an example of finding absolute values of integers and floats:
x = -5
absolute_x = abs(x)
print(absolute_x) # Outputs 5
y = -3.2
absolute_y = abs(y)
print(absolute_y) # Outputs 3.2
This function takes both positive and negative numbers and float values, and it returns the distance from zero as positive numbers in both cases.
2. How to Find the Absolute Values of Complex Numbers
Python also has built-in support for complex numbers, which consist of real and imaginary parts.
The built-in function abs() can compute the absolute value of a complex number, also known as its magnitude. It does so by calculating the square root of the sum of the square of its real and imaginary components.
For example, the absolute value of a complex number can be calculated as follows:
z = 3 + 4j
absolute_z = abs(z)
print(absolute_z) # Outputs 5.0
In this example, the real part of the complex number is 3, and the imaginary number is 4. The magnitude of the complex number 5.0 is computed using the Pythagorean theorem. This states that the magnitude is equal to the square root of the sum of the squares of the real and imaginary parts (sqrt(9 + 16) in this case).
The output will be:
Applications and Examples of Absolute Values in Python
In this section, we’ll go look at some examples and applications of finding absolute values in Python. The examples will help you understand when to use the absolute value functions.
We’ll go over the following applications:
- Calculating Distance
- Temperature Differences
- Error Calculation
1. Calculating Distance
The abs() function is helpful when computing distances between two points, typically on a number line or a Cartesian plane.
For example, if you wish to find the distance between two points on a number line with coordinates a and b, you can calculate the absolute value of their difference:
a = 5
b = -2
distance = abs(a - b)
print(distance)
The output will be:
2. Temperature Differences
Another valuable application involves temperature differences. Suppose you want to compare two temperature readings in Celsius and express the difference in absolute terms.
You can use the abs() function for this purpose:
temp1 = 10
temp2 = -4
abs_difference = abs(temp1 - temp2)
print(abs_difference)
From the above example, you learn that there is a 14-degree difference between the negative integer -4 and positive number 10. The return value is 14, as shown below:
3. Error Calculation
You can also use the absolute value to calculate the error or difference between a predicted value and the actual value in various machine learning algorithms.
For instance, Mean Absolute Error (MAE) is a common metric for regression models, which measures the average magnitude of the errors in a set of predictions, without considering their direction.
You can use the abs() function to calculate the absolute values in this case:
def mae(y_true, y_pred):
return sum(abs(y_t - y_p) for y_t, y_p in zip(y_true, y_pred)) / len(y_true)
y_true = [3, -0.5, 2, 7]
y_pred = [2.5, 0.0, 2, 8]
print(mae(y_true, y_pred))
The output will be:
Learn more about Python’s applications by watching the following video:
Final Thoughts
Understanding the use of absolute values in Python proves to be an essential tool in your programming journey. It’s a simple concept to learn, yet the value it brings to your code can’t be overstated. Whether it’s refining data, calculating distances, or improving machine learning models, the utility of absolute values is immense.
This simple function can make your code more robust, efficient, and comprehensible. The magic of programming lies in mastering these small yet powerful functions to solve complex problems.
In this article, we explored the concept of absolute value and its implementation in Python using the built-in abs() function, which is highly useful in a variety of mathematical, scientific, and data analysis scenarios. You saw that Python’s abs() function is versatile and accepts integers, floats, and complex numbers as input.
Frequently Asked Questions
How do I calculate the absolute value in Python?
To calculate the absolute value in Python, you can use the built-in abs() function.
For example:
abs(-10) # returns 10
abs(4.5) # returns 4.5
abs(-3.2) # returns 3.2
abs(3+4j) # returns 5.0
What is the alternative to the abs() function in Python?
An alternative to abs() in Python is the math.fabs() function. This function works for floating-point numbers and returns a float.
To use math.fabs(), you’ll need to import the math module first:
import math
math.fabs(-7) # returns 7.0
math.fabs(-2.4) # returns 2.4
How does Python’s fabs function work?
Python’s fabs function from the math module calculates the absolute value of real and complex numbers(integer or floating-point numbers), returning a floating-point result.
First, import the math module and then use math.fabs():
import math
number = -3.7
result = math.fabs(number) # returns 3.7
How do I calculate the absolute difference between two numbers in Python?
To calculate the absolute difference between two numbers in Python, you can subtract one number from the other and use the abs() function to return the non-negative result:
num1 = 5
num2 = 12
diff = abs(num1 - num2) # returns 7
What is the role of the abs() function in programming?
The abs() function plays a vital role in programming by providing a way to calculate the absolute value or non-negative distance of a number from zero.