Reversing a range in Python is a useful skill for programmers when they need to manipulate the order of sequences or iterate over elements in a non-conventional order. Python offers several built-in functions and techniques that allow developers to reverse a range effortlessly.
The range() function in Python is helpful in generating a sequence of numbers within a given range. By incorporating reverse techniques using functions like reversed() or adding a negative step in the range() function itself, we can effectively create a reverse range.
In this article, we’ll take a closer look at each method including, using the range function with a negative step, utilizing the reversed function, and other alternatives.
We’ll also cover some examples to showcase the implementation and provide a clear understanding of reversing a range in Python.
Let’s dive into it!
How to Create a Simple Range in Python
You can create a simple range using the built-in range() function in Python 3.
The range() function generates a series of numbers, usually used for looping a specific number of times in for loops. It can take one, two, or three parameters:
range(stop): Creates a range of numbers from 0 up to, but not including, the stop value.
range(start, stop): Creates a range of numbers from the start value up to, but not including, the stop value.
range(start, stop, step): Creates a range of numbers from the start value up to, but not including, the stop value, with a custom step value between each number.
The numbers generated in the range are integers and can be either in ascending or descending order, depending on the values provided.
Here’s an example of creating a range with different parameters:
# Range up to 5, excluding 5
simple_range = range(5)
print(list(simple_range))
# Output: [0, 1, 2, 3, 4]
# Range from 2 to 8, excluding 8
custom_start = range(2, 8)
print(list(custom_start))
# Output: [2, 3, 4, 5, 6, 7]
# Range from 1 to 10, excluding 10, with a step value of 2
step_value = range(1, 10, 2)
print(list(step_value))
To create a range with a descending order, you can use a negative step value:
# Range from 10 to 0, excluding 0, with a step value of -1
descending_range = range(10, 0, -1)
print(list(descending_range))
The above code snippet will return:
That covers the fundamentals of the range() function. In the next section, we’ll take a look at the five best ways of reversing a range in Python.
5 Ways How to Reverse a Range in Python
In this section, we are going to cover 5 different ways of reversing a python range. The python range reverse methods discussed are :
Using Range, List and Reversed Functions.
Using Range Function with negative step parameter value.
Using Range Function and list slicing.
Using the Sorted Function.
Using NumPy.
1. How to Use the Python Range, List, and Reversed Functions
This section covers in detail how Range(), List(), and Reversed() functions are used together to reverse a Python range into a reversed range.
Range() function is used to create the Python range just as discussed in the above section.
List() function is used to convert the range to an iterable object.
Reversed() function is used to convert the iterable object to a reversed range.
The reversed() function is a built-in function in Python, which is used to reverse an iterable. It takes a single parameter, the iterable to be reversed, and returns an iterator in the reversed order.
The reversed() function can’t be used directly to reverse a range object. This is because range objects are not iterable in the usual sense.
To reverse a range, you need to convert it to a list or another iterable object first and then use the reversed() function on that iterable.
Here’s a step-by-step explanation of how to use the reversed() function to reverse a range in Python:
1. Define a range object using the range() function. For example, let’s create a range from 1 to 10:
my_range = range(1, 11)
2. Convert the range object to a list using the list() function:
my_list = list(my_range)
The list() function converts the range object into a list, which is an iterable object that can be reversed.
3. Use the reversed() function to reverse the list:
reversed_list = list(reversed(my_list))
The reversed() function takes the list as an argument and returns a reverse iterator. To convert it back to a list, we use the list() function again.
4. Now, you have the reversed list stored in the reversed_list variable. You can use it as desired. For example, you can iterate over the reversed list using a loop:
for item in reversed_list: print(item)
This loop will print the elements of the reversed list in reverse order. The decreasing numbers start from 10 down to 1.
Output
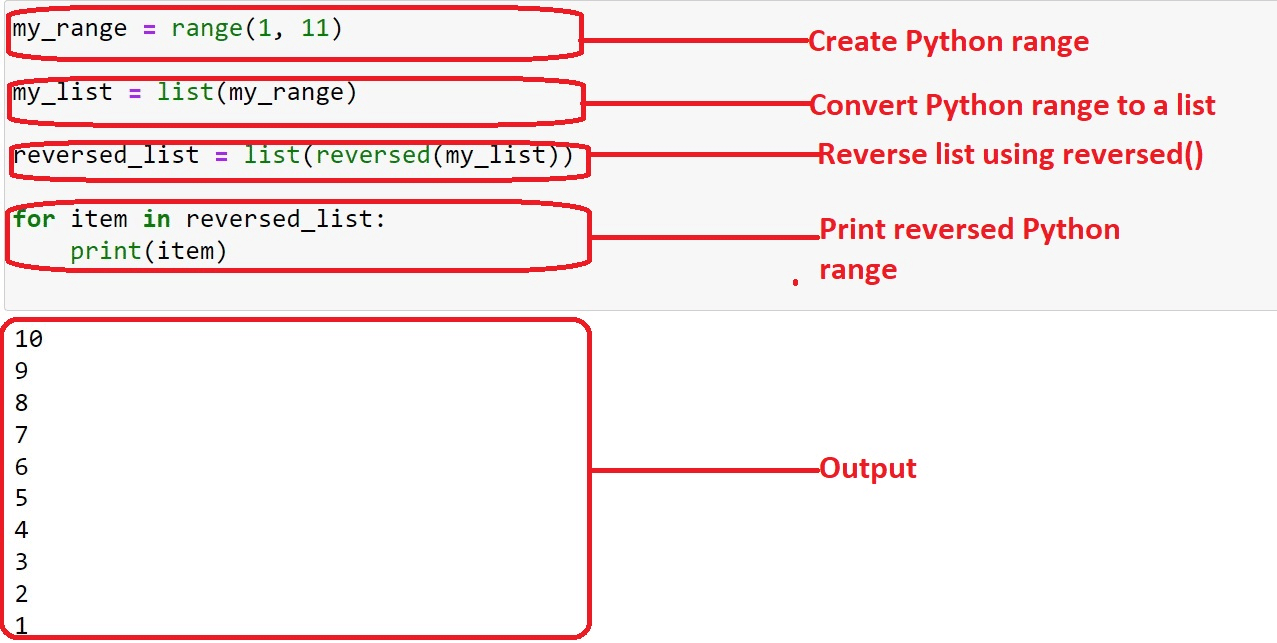
In summary, to reverse a range in Python, you need to convert it to a list, use the reversed() function on the list, and then convert the resulting reverse iterator back to a list if needed.
2. How to Use the Python Range Function with Negative Step Parameter
original_range = range(1, 10)
reversed_range = range(max(original_range), min(original_range) - 1, -1)
for num in reversed_range:
print(num)
Here, we utilize the range() function with a negative step argument value.
By setting the default start value as the maximum number in the original range and the default stop value as the minimum number minus one, we ensure that the range is generated in reverse order.
The negative step value of -1 instructs the range to decrease by one on each iteration. The function returns:
3. How to Use Python Range Function and List Slicing
original_range = range(1, 10)
reversed_range = list(original_range)[::-1]
for num in reversed_range:
print(num)
In this method, we convert the range object to a list using the list() function and then apply list slicing to reverse the order of the range elements.
The [::-1] syntax creates a slice that starts from the end of the list and goes backwards, effectively reversing the list.
The result is a list that represents the reversed range.
4. How to Use the Sorted Function in Python
The sorted() function can be used with the reverse=True parameter to reverse a range. Here’s an example:
for i in sorted(range(10), reverse=True):
print(i)
This will create a range object and then reverse the sequence, outputting the numbers in descending order.
5. How to Use Python NumPy
Finally, you can use the NumPy library to reverse a range easily:
import numpy as np
numbers = np.arange(10)[::-1]
for i in numbers:
print(i)
This code utilizes NumPy’s arange() function to create an array and reverses it using the slicing syntax [::-1]. The output will display numbers from 9 to 0.
In the next section, we look at the practical applications of reversing a Python range.
Applications and Practical Examples of Python Range Reverse
Reversing a range in Python has numerous practical applications in various domains.
In this section, we will discuss some of the common use cases and provide examples for different scenarios.
1. Data Manipulation and Analysis
In data manipulation and analysis, Python’s range reversal technique can be employed for tasks like sorting data in descending order, plotting data in reverse chronological order, or even filtering data.
With libraries like NumPy, you can efficiently iterate through arrays, lists, or matrices using reversed ranges to perform manipulations.
For example, given an input array of sales data, you can use a reversed range to calculate the top 5 highest sales using the following snippet:
import numpy as np
sales_data = np.array([100, 200, 150, 500, 250, 50])
sorted_indices = np.argsort(sales_data)[::-1]
top_sales = sales_data[sorted_indices[:5]]
To learn more about how to load a dataset in Python, check out the tutorial in the following video:
2. Algorithm Implementation
Various algorithms, particularly in search and sorting, may require iterating through the elements in reverse order.
In programming languages like Python, using reversed ranges makes it easier to implement such algorithms without modifying the original data.
For example, as part of an algorithm that expects a sequence of numbers in decreasing order, you can reverse the range using the range(start, stop, step) function with a negative step parameter:
for i in range(10, 0, -1):
print(i)
Final Thoughts
In conclusion, reversing a range in Python, though it might seem a bit tricky at first, is quite straightforward once you understand the concept. There are various methods to achieve this, ranging from slicing techniques to using built-in functions like `reversed()` or implementing loops.
The beauty of Python lies in its simplicity and the flexibility it offers to approach a problem from multiple perspectives. The method chosen largely depends on the specific requirements of your program and your personal coding style.
As you continue exploring Python, you’ll find that its rich set of features and functionalities make it an incredibly powerful tool for any programming task. Always remember, the key to mastering Python or any programming language is consistent practice and exploration. Happy coding!