If you’re working with SQL or SQL Server, you’re probably dealing with a wide variety of data type options every day. Now, we all know how important it is to ensure our data types are compatible with our operations. That’s where the SQL Cast function comes into play.
The SQL Cast function helps you convert one data type into another. To use it, you specify the CAST function followed by the value you want to convert in parentheses, then AS, and finally the data type you want the value converted to.
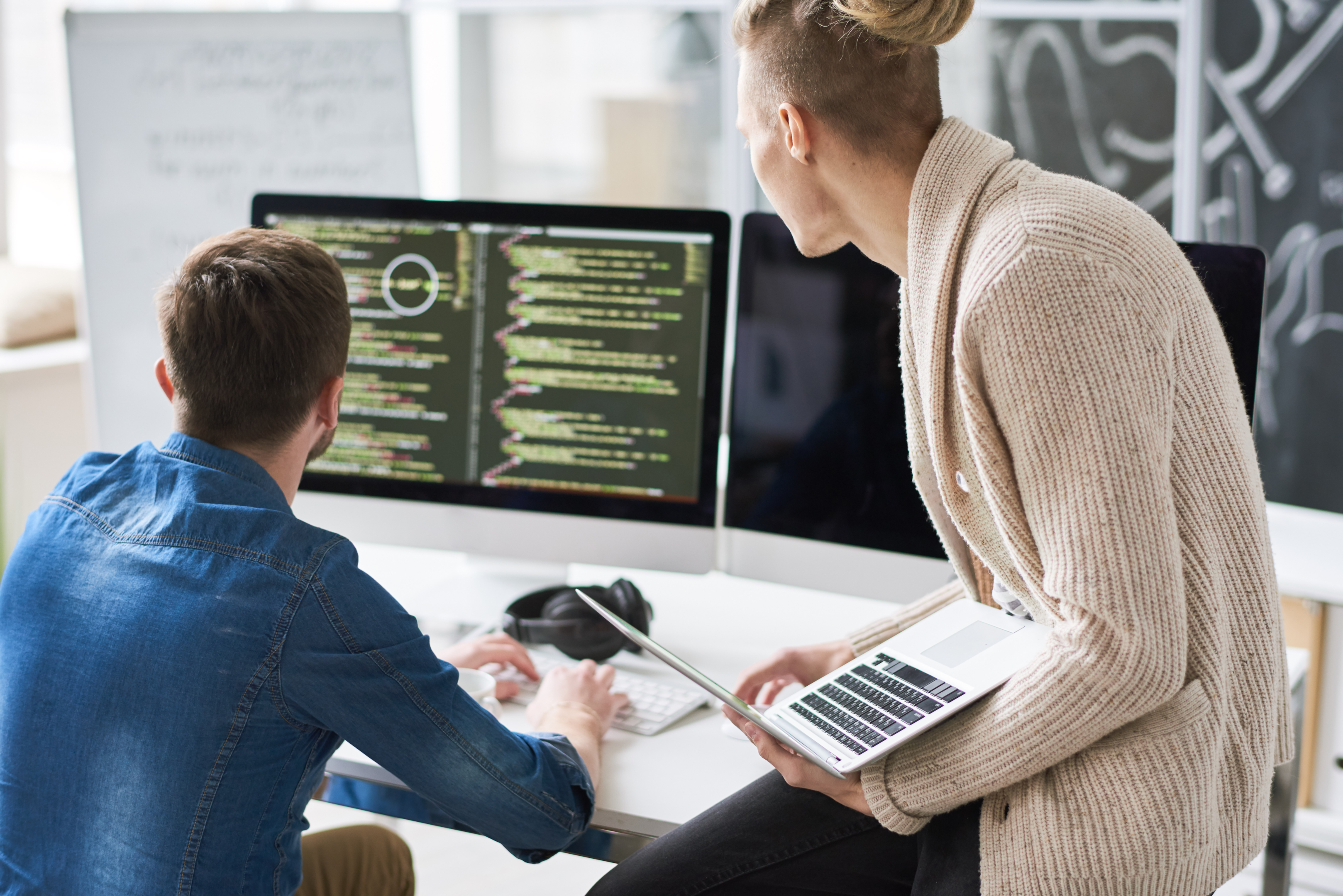
In this article, you’ll learn how to convert data from one type to another using SQL CAST function. This includes a detailed exploration of the syntax and parameters of the CAST function, and how to use it to convert various data types, such as strings to integers, integers to decimal numbers, or even date strings to actual date data types.
Let’s dive in!
What is the CAST() Function in SQL?
Imagine you’re integrating data from different databases and stumble upon a date stored as a string in one database and as a date object in another.
Sounds like a headache, right? Not with the CAST() function!
This powerful and simple command can standardize the desired data type across different databases.
The CAST function in SQL is used to convert a data type of one data type into another data type. This can be particularly useful when you need to manipulate the data type of a column to perform certain operations that are specific to a different data type.
If you want to present numerical data in a more readable format, the CAST() function allows you to convert those numbers into strings and even append symbols for better presentation.
If you have numerical values stored as strings and need to perform some calculations on them, you can use the CAST() function to convert the string data type to an integer.
What is The Syntax and Parameters of the CAST Function
The basic syntax of the CAST function is given below:
CAST (expression AS data_type [length])
In this syntax, expression is the value or values you want to convert, and data_type is the target data type you want to convert the expression into.
The parameters of the CAST function are listed below:
- Expression: This is the value or column value that you want to convert.
- Data_type: This is the target data type you want to convert the expression into. It could be any valid SQL data type, like INT, DATE, VARCHAR, etc.
- Length: This is an optional parameter. Certain data types, such as VARCHAR, require or allow a length specification, which determines how many characters the converted value should have.
How to Use CAST Function to Convert Data Types
Now that you’ve learned the syntax and parameters of the CAST function, let’s see how you can use it for data type conversion.
The following is an example of using CAST to convert a numeric value into a character string:
This would return ‘12345’ as a string, not a number.
What is The Different Between Cast And Convert
When working with SQL, you’ll find these two functions for data conversions most useful to your workflow: CAST() and CONVERT().
These can be used to change the data type of a value or an expression. While they might seem similar, there are important differences between them that you should be aware of.
1) The Cast Function
The SQL Server Cast function is a part of the ANSI-SQL specification, making it a standard across different SQL implementations.
The syntax of the Cast() function is straightforward like the following:
CAST (expression AS data_type)
Here, ‘expression’ can be a column or a value that you want to convert, and ‘data_type’ is the target data type you want to convert the expression to.
2) The Convert Function
The Convert() function, on the other hand, is specific to SQL Server. It offers more flexibility than SQL Server Cast(), especially when dealing with date and time values.
The syntax of the Convert() function is:
SELECT CONVERT (data_type, expression, [style])
The ‘style’ parameter is an optional parameter and allows you to format the output, which is not possible with Cast().
For instance, to convert a date string data type variable to a datetime value in a specific format, you can use the following query:
SELECT CONVERT(DATETIME, '2020-12-31', 101) AS NewFormat;
This will be used to convert to the date string to a datetime value and format it as ’12/31/2020′.
The output will be:
As you can see, Convert() has an optional style parameter allowing you to format the output into a numeric data type variable, especially when dealing with date/time and money/data type conversions.
SELECT CONVERT(varchar, '2023-07-11', 103)
The result would be
11/07/2023
If you’re looking for a simple, straightforward data type conversion and want your code to be highly portable, then Cast() is your best bet.
However, if you’re working with SQL Server and need additional control over the formatting of your data, particularly with dates and times, then Convert() would be more suitable.
4 Examples of Using the CAST() Function
In this section, we will review 4 examples of using the SQL Cast function in various scenarios involving SELECT statement, INNER JOIN, and GROUP BY.
Example 1: Converting a Decimal Number to an Integer
Suppose you have a column with decimal numbers, and you need to convert them to integers.
You can use the CAST function as shown below:
SELECT CAST(your_column_name AS INT)
FROM your_table_name;
The demonstration of each line is shown below:
The output will be:
Example 2: Converting a VARCHAR To a DATETIME
In SQL Server, you can convert a VARCHAR to a DATETIME using the CONVERT function.
The syntax is as follows:
CONVERT(DATETIME, your_varchar_column)
For example, consider a table named Events with a VARCHAR column EventDate, which contains dates stored as strings in the format ‘YYYY-MM-DD.
You can convert these varchar dates to datetime like this:
The output would look like this:
It’s important to note that the VARCHAR string must represent a valid date. If it doesn’t, the conversion will result in an error.
Example 3: Using CAST With INNER JOIN
Suppose you have two tables with a common key, but the data types of the key are different – one is an integer and the other is a varchar.
We’ll use the CAST function to perform an inner join between these tables.
First, we’ll create two tables: Table1 and Table2.
Now, if you want to join these two tables on the ID field, you need to convert the ID in Table2 to an integer as shown below:
SELECT t1.ID, t1.Name, t2.Description
FROM Table1 t1
INNER JOIN Table2 t2 ON CAST(t2.ID AS INT) = t1.ID;
In this case, the CAST function is used to convert the ID field in Table2 from a varchar to an integer so it can be compared to the integer ID field in Table1.
The INNER JOIN only returns the rows where there’s a match between the ID fields in both tables.
The output would be:
Example 4: Using CAST with GROUP BY
Sometimes, you may want to group the result set by a specific expression.
For example, let’s assume you have a table with sales data, and you want to group it by year and month. You can use the SQL Cast function with GROUP BY like this:
Now, if you want to group these sales by year and month, you can use the CAST function like this:
SELECT CAST(YEAR(SaleDate) AS VARCHAR(4)) + '-' + RIGHT('00' + CAST(MONTH(SaleDate) AS VARCHAR(2)), 2) AS YearMonth,
SUM(Amount) as TotalSales FROM Sales
GROUP BY CAST(YEAR(SaleDate) AS VARCHAR(4)) + '-' + RIGHT('00' + CAST(MONTH(SaleDate) AS VARCHAR(2)), 2);
The output would be:
What Are the Types of Data Type Conversion
In SQL, data type conversion is a crucial aspect of working with different data types within the database.
The key difference between implicit and explicit conversion is control. While implicit conversion can make your code cleaner and easier to read, explicit conversion gives you more control over your data and helps prevent unexpected errors or results.
There are two primary types of data type conversions:
- Implicit Conversion
- Explicit Conversion
Let’s go over each and understand how they differ from each other.
1) What is Implicit Conversion
Implicit conversion is when SQL automatically converts one data type to another without you having to explicitly command it to do so.
This usually happens when you’re trying to combine or compare different data types in a single statement.
For example, if you try to add an integer value and a decimal together, SQL will implicitly convert the integer to a decimal before performing the operation.
The following code demonstrates this:
SELECT CAST((3 + 4.5) AS FLOAT) AS Result;
In this case, the integer 3 is implicitly converted to a decimal 3.0, and the function returns a result that is added to a decimal point 7.5.
2) What is Explicit Conversion
Explicit conversion is when you instruct SQL to convert a data type to another using functions like the SQL Cast.
This gives you more control over your data manipulation.
The following is an example of explicit conversion using the Cast() function:
SELECT CAST('2021-01-01' AS DATE) AS NewDate;
In this example, the string ‘2021-01-01’ is explicitly converted to a date.
Important Points to Remember When Working With Explicit Conversions
When working with explicit conversions in SQL, there are several important points to remember.
We have listed some important pointers below:
- Data Loss: Explicit conversions can lead to data loss if you’re not careful. For instance, if you convert a decimal number to an integer, the fractional part will be truncated.
- Conversion Functions: SQL provides two main functions for explicit conversion: CAST and CONVERT. While both can be used for similar purposes, CONVERT provides additional flexibility with date and time conversions.
- Compatibility: Not all data types can be converted to others. For example, you cannot convert a string that contains non-numeric characters to an integer. Attempting to do so will throw an error.
- Precision and Scale: When converting numeric data types, be mindful of precision and scale. Precision is the total number of digits, while scale is the number of digits after the decimal point. If you’re converting to a type with lower precision or scale, rounding or truncation may occur.
- Date and Time Formats: When converting strings to date or time data types, the string must be in a format that SQL Server recognizes as valid for a date/time. Otherwise, you’ll get an error.
- Performance: Excessive or unnecessary conversions can negatively impact query performance. It’s best to store data in the appropriate type from the beginning to minimize the need for conversions.
- Error Handling: Be prepared to handle errors that may arise from unsuccessful conversions. This could include catching exceptions or validating data before attempting a conversion.
Learn more about SQL tables and functions by watching the following video:
Final Thoughts
The SQL CAST and CONVERT functions are your go-to tools for data type conversions. You can use SQL CAST and CONVERT functions to prevent future headaches by ensuring your data is formatted properly.
This is because data type conversion is based on compatibility rules of the SQL database system you are using. The Cast() function is widely supported across various SQL dialects and can be a powerful tool in your SQL toolbox for converting data types when needed.
When used correctly, it can greatly enhance the flexibility and robustness of your SQL queries and operations. As with all functions and commands in SQL, it’s essential to understand how they work, their syntax, and their potential pitfalls.
Frequently Asked Questions
In this section, you’ll find some frequently asked questions that you may have when working with CAST in SQL.
How to type CAST to string in SQL?
To convert a value to a string in SQL, you can use the CAST function. The syntax is as follows:
CAST(expression AS VARCHAR(max_length))
Here, expression is the value or column you want to convert, and max_length is the maximum number of characters you want in the output string.
How do I CAST a char to a string?
In SQL Server, CHAR is already a string datatype, albeit of fixed length.
If you want to convert it to a VARCHAR, which is a variable-length string datatype, you can use the CAST function like this:
SELECT CAST(your_char_column AS VARCHAR(max_length)) FROM your_table;
What is safe CAST in SQL?
SAFE_CAST in SQL is a function that attempts to cast a value to a specified data type, and returns NULL if the cast is not possible.
This prevents errors that might otherwise occur if you try to cast a value to an incompatible data type.
Here’s the syntax for SAFE_CAST:
SAFE_CAST(expression AS data_type)
What if CAST fails SQL?
If a CAST operation fails in SQL, it typically results in an error.
However, to avoid this, SQL Server provides the TRY_CAST() function which returns NULL instead of an error when the cast fails.
Here’s how you can use TRY_CAST:
TRY_CAST(expression AS data_type)
In this syntax, expression is the value or column you want to convert, and data_type is the target data type to which you want to convert the expression.