As you continue your journey as a Python programmer, you’ll want to write code that is more efficient, readable, and easy to maintain. The Python programming language comes with a list of tools that allows you to simplify your code for better readability. One such tool is Python’s inline if statement.
In Python programming, an inline if statement, also known as a conditional expression or ternary operator, is used to assign a value to a variable based on some condition. It’s a compact version of the regular if statement.
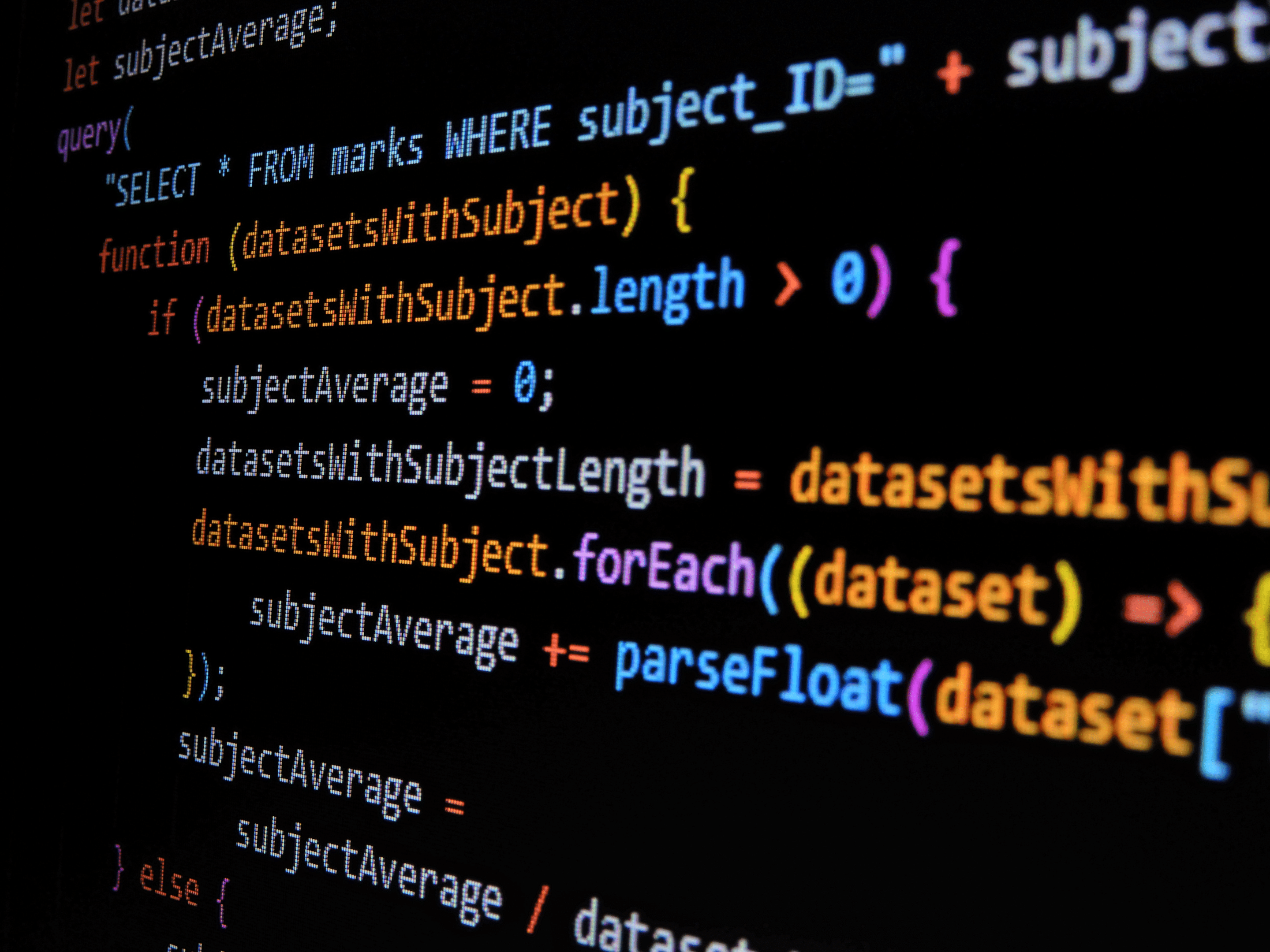
Using the ternary operator in Python enables you to embed an if statement within other expressions. This offers a higher level of flexibility and control. When you use inline if statements in your code, you can maintain Python’s principles of readability while maximizing efficiency.
In this article, we’ll break down the Python inline if and show you how it works and when to use it. We’ll also share some handy examples so you can see it in action. So, buckle up and let’s simplify your conditional expressions with the Python inline if!
What is the Syntax of Inline If in Python?
The following is the syntax of Python inline if:
value_if_true if condition else value_if_false
In the above syntax, we first evaluate the condition, which results in a boolean value. If the condition is true, the value_if_true is returned. Otherwise, the value_if_false is returned.
However, in order to understand the syntax better, it’s important to take a look at the various components of the inline if statement.
What Are the Components of Inline if in Python?
The Python inline if statement has three main components:
Condition: The expression that is evaluated, resulting in a boolean value (True or False).
Value_if_true: The value returned if the condition is true.
Value_if_false: The value returned if the condition is false.
The following is an example of a Python inline if statement:
x = 5
y = "Even" if x % 2 == 0 else "Odd"
print(y)
In this example, the condition is x % 2 == 0, which checks if the value of x is even. If the condition is true, the variable y is assigned the string “Even”; otherwise, it gets assigned the string “Odd”.
Python inline if statements can also be used in different constructs, such as list comprehensions. An example of this is given below:
data = [1, 2, 3, 4, 5]
squared = [x*x if x > 2 else x for x in data]
print(squared)
In this list comprehension, we square each value in the list data if it is greater than 2. Otherwise, it returns the value without squaring it.
Using inline if statements can make your code easier to read when applied appropriately. However, it’s crucial that you maintain a balance between concision and readability.
If your inline if statement is too complex, it may be better to revert to a multi-line if-else structure for clarity.
How to Handle Multiple Conditions in Python Inline If
As a Python programmer, handling multiple conditions in Python is a task that you may encounter. To handle multiple conditions, you can use the elif and inline together.
In Python, the elif is used as a shorthand for an if-else statement. When using the if-else structure, you can chain any number of elif statements to write more complex code.
Suppose we want to categorize the given input as small, medium, or large based on the value of a variable. You can do this using the following if-else code:
x = 15
if x < 10:
size = 'small'
elif x < 20:
size = 'medium'
else:
size = 'large'
print(size)
In the above example, you can see the else clause used to capture alternate conditions. To get the same result using Python inline if-else statement, you can nest the if-else expressions like so:
x = 15
size = 'small' if x < 10 else 'medium' if x < 20 else 'large'
print(size)
Let’s also explore some of the advanced use cases of an inline statement. These use cases will help you better understand when to use these statements.
Advanced Use Cases of Inline If in Python
As you explore Python inline if, you’ll want to know its advanced use cases. This section will do just that for you and show you examples of how to use inline if statements in various ways.
Specifically, we’ll be looking at the following:
Using inline if in loops
Using conditional expressions within inline if
Let’s get into it!
1. How to Use Inline If in Loops
You can use inline if statements within loops in Python. This will help you write more readable code.
Let’s consider a situation where you want to print the squares of even numbers and the cubes of odd numbers in a given range. You can achieve that in a single line using the for statement combined with an inline if, as shown below:
for i in range(1, 11):
print(i ** 2 if i % 2 == 0 else i ** 3)
This will output the calculations for each number in the range without the need for a full if-else block in multiple lines.
2. How to Use Conditional Expressions Within Inline If
You can use conditional expressions with inline if statements by nesting your code. This is useful when handling multiple conditions in your script.
The syntax to use conditional expressions within inline if is shown below:
value_if_true if condition1 else (value_if_true2 if condition2 else value_if_false)
To help you better understand the concept, take a look at the following example:
x = 5
result = (
"x is equal to 5"
if x == 5
else ("x is between 1 and 10" if 1 <= x <= 10 else "x is not between 1 and 10")
)
print(result)
This nested inline if statement evaluates multiple conditions and returns the output.
Principles to Keep in Mind When Writing Advanced Inline If Statements
When you’re using advanced inline if statements, consider:
Limiting the nested levels, as nested inline if statements can be hard to read.
Using parentheses to improve readability.
Using these guidelines, you can make great use of inline if statements within loops and conditional expressions.
Now that you’ve understood the basics of inline if statements, let’s take a look at what are the best practices and common pitfalls when writing such statements in the next section!
Best Practices and Common Pitfalls When Writing Python Inline If Statements
We’ve listed a few best practices and common pitfalls to make your code more presentable. Specifically, we’ll be looking at the following:
Making proper indentation
Avoiding using semicolons
Let’s dive into it!
1. Make Proper Indentation
When you are using inline if statements, it is important to use proper indentation. This will help you in maintaining readability and understanding the code flow.
In the example below, we’re comparing two ways of writing the same code:
# Without proper indentation
my_value = 5
if my_value > 10: print("Value is greater than 10"); print("This might be confusing"); else: print("Value is not greater than 10"); print("It's really hard to follow this code.")
# With proper indentation
my_value = 5
if my_value > 10:
print("Value is greater than 10")
print("This is much easier to understand")
else:
print("Value is not greater than 10")
print("Now it's clear what this code does.")
You can use the following tips to make proper indentation:
Use consistent indentation, preferably four spaces, throughout your code.
Avoid mixing tabs and spaces for indentation.
Always indent the nested expressions to show the flow.
2. Avoid Using Semicolons
You can use semicolons to write multiple statements on a single line, but they’re discouraged. In the context of inline if, using semicolons can lead to confusion and less readable code.
The following example shows why you should avoid semicolons:
# Good practice
grade = "A" if score >= 90 else ("B" if score >= 80 else "C")
# Bad practice (semicolons)
grade = "A" if score >= 90 else "B"; if score >= 80 else "C"
When working with inline if statements, it’s important to stick to best practices and understand common pitfalls.
Now that you’ve understood the best practices and common pitfalls when working with inline if statements, let’s look at how you can use it with other Python features in the next section.
How to Use Inline If With Other Python Features
In this section, we’ll explore how to use the inline if together with other Python features. We’ll be looking at the following two use cases:
Using inline if with lambda functions
Using inline if for input validation
1. How to Use Inline If with Lambda Functions
You can use lambda functions to create simple functions. These functions consist of a single expression that’s evaluated when the lambda function is called.
The following is an example of using inline if with lambda functions:
multiply_or_add = lambda x, y: x * y if x > 5 else x + y
result = multiply_or_add(3, 4)
print(result)
In this example, the lambda function multiply_or_add takes two arguments x and y. It multiplies them if x is greater than 5; otherwise, it adds them. The inline if allows us to express this logic in a single line.
2. How to Use Inline If for Input Validation
You can use inline if for input validation as well. When receiving input from a user or external source, it is necessary to validate that the input meets certain criteria.
For instance, you might want to ensure that an inputted value is an integer or that it falls within a specific range. You can achieve this by the following code:
input_value = input("Enter a number between 1 and 100: ")
integer_value = int(input_value) if input_value.isdigit() else None
if integer_value is None or not (1 <= integer_value <= 100):
print("Invalid input, please enter a number between 1 and 100.")
else:
print(f"Your input is {integer_value}.")
In this example, we prompt the user to enter a number between 1 and 100. First, we use the inline if to check if the input is a digit and convert it to an integer.
Then, we use another inline if to validate that the integer falls within the given range. If the input is invalid, we print an error message; otherwise, we print the inputted integer.
To know more about handling errors in Python, check out the following video:
Final Thoughts
On your journey with Python, you’ll find the inline if statement useful in multiple scenarios. It helps make your code shorter and cleaner. Once you master the use of inline if, it’ll boost your coding speed and enable you to solve problems with style and efficiency.
Why learn it if you have the simple if statement? It’s important to learn the inline if statement because it makes your code easier to understand for others to understand.
Furthermore, using the inline if statement adds a new style to your code that’s commonly found in the code of expert Python programmers. With inline if, you use fewer lines of code and more straightforward logic.
In the end, the Python inline if is all about making your life as a coder a little bit easier. It’s about writing cleaner, more efficient code without sacrificing readability. So, give it a shot, play around with it, and see how it can simplify your conditional expressions. Happy Pythoning!