In the Python programming language, understanding operators is as crucial as understanding the alphabet for reading. Among these operators, ‘==’ holds a unique significance. Often mistaken for a single equal sign ‘=’, the ‘==’ operator plays a different, yet extremely important role in Python.
In Python, the “==” operator is used for comparison purposes. It compares whether the values on the left and right sides of the operator are equal, returning either True or False. In contrast, the “=” symbol is an assignment operator used to assign a value to a variable.
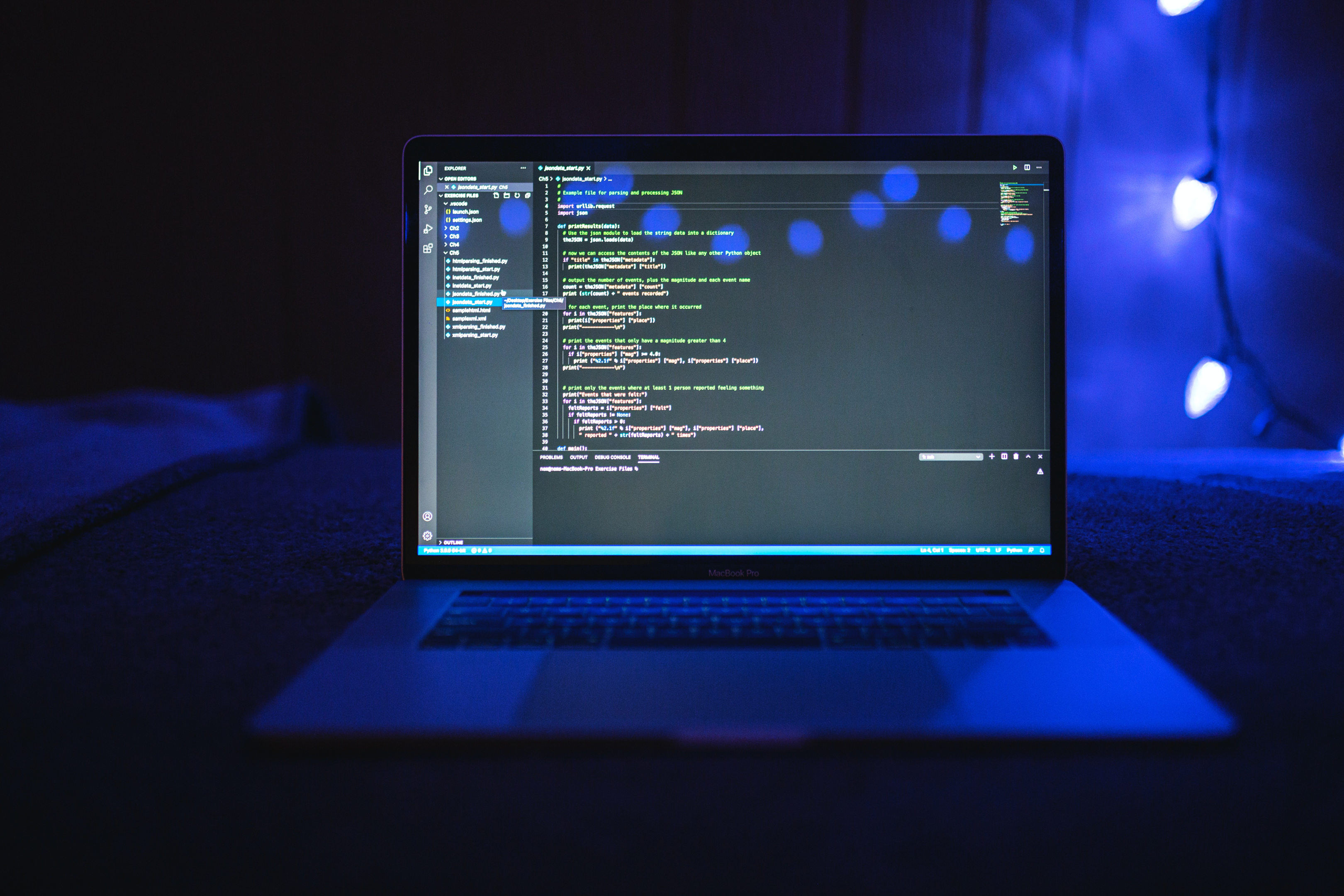
If you’re just getting started with learning Python, it is important to understand the concept of different Python operators, including “=” for value assignment, “==” for equality comparison, and other arithmetic, logical, and bitwise operators.
In this guide, we’ll navigate the waters of Python operators, with a specific focus on ‘==’, exploring its function, uses, and intricacies.
Let’s dive in!
What Are Comparison Operators in Python?
Before we dive into code examples, let’s quickly review what comparison operators are in Python.
Like every other programming language, Python comes loaded with a range of operators. The “==” is a Python identity operator that allows you to compare values or variables. It’s commonly called a comparison operator.
The comparison operator is used to compare values and return a boolean result (True or False).
The following is a list of comparison operators in Python:
- ==: Checks if the values are equal (“abc” == “abc” => True)
- !=: Checks if the values are not equal (e.g., 2 != 3 => True)
- <: Checks if the left value is less than the right value: (e.g., 2 < 4 => True)
- >: Checks if the left value is greater than the right value (e.g., 6 > 4 => True)
- <=: Checks if the left value is less than or equal to the right value (e.g., 4 <= 4 => True)
- >=: Checks if the left value is greater than or equal to the right value (e.g., 2 >= 1 => True)
What is the == Operator in Python?
In Python, you can use the equality operator to compare two variables for equality. When comparing the same object values, it returns True, and False otherwise.
The operator is different from the assignment operator =, which is used to assign a value to a variable.
The following is an example of the == operator in Python:
a = 5
b = 5
c = a
print(a == b)
print(a == c)
In the above example, we compare two variables, a with b, and a with c using the == operator.
The code checks if a variable equal b.
The output of this code is given below:
How to Compare Different DataTypes With == Operator
You can use the == operator to compare different data types as well, such as strings, integers, and floating-point numbers.
The following example demonstrates comparing different data types:
number1 = 7
number2 = 7.0
string1 = "Hello"
string2 = "hello"
print(number1 == number2) # True, as 7 and 7.0 have the same value
print(string1 == string2) # False, as 'Hello' and 'hello' are different strings (case-sensitive)
When comparing different data types, the == operator checks if their values are equal.
It doesn’t matter if the variables are of different types, as long as they represent the same value, the return value will be true.
How to Compare Collections With == Operator
You can compare collections objects with the == operator as well. In Python, collections are lists, tuples, and dictionary data types.
In this section, we’ll go over how you can compare lists, tuples, and dictionaries using the == operator.
1. How to Compare Lists With == Operator
A list in Python is an ordered collection of items that can be of any type. Lists are mutable, meaning their elements can be changed after they are created.
The == operator compares the values of lists element-wise. Two lists are equal if they have the same length and their elements are equal in the same order.
The following example demonstrates comparing lists with the == operator:
# Lists comparison
list1 = [1, 2, 3]
list2 = [1, 2, 3]
list3 = [1, 2, 4]
print(list1 == list2) # Returns True
print(list1 == list3) # Returns False
This code compares Python lists using the equality operator ==. When list1 is compared to list2, it returns True because they have the exact same elements in the same order.
However, when list1 is compared to list3, it returns False because the last elements of the lists are different (3 vs 4).
2. How to Compare Tuples With == Operator
A tuple in Python is an ordered collection of items, similar to a list, but it is immutable, meaning its elements cannot be changed after it is created.
Two tuples are equal if they have the same length and their elements are equal in the same order.
The following example demonstrates comparing tuples with the == operator:
# Tuples comparison
tuple1 = (1, 2, 3)
tuple2 = (1, 2, 3)
tuple3 = (1, 2, 4)
print(tuple1 == tuple2) # Returns True
print(tuple1 == tuple3) # Returns False
This code compares Python tuples using the equality operator.
The first comparison of tuple1 and tuple2 returns True because both tuples have exactly the same elements in the same order.
The second comparison tuple1 == tuple3 returns False because the last elements of the tuples are different (3 vs 4).
3. How to Compare Dictionaries With == Operator
A dictionary in Python is an unordered collection of key-value pairs, where each key is unique.
Two dictionaries are equal if they have the same keys and the values associated with each key are equal.
The following example demonstrates comparing dictionaries with the == operator:
# Dictionaries comparison
dict1 = {'a': 1, 'b': 2, 'c': 3}
dict2 = {'a': 1, 'b': 2, 'c': 3}
dict3 = {'a': 1, 'b': 2, 'c': 4}
print(dict1 == dict2) # Returns True
print(dict1 == dict3) # Returns False
This code compares Python dictionaries using the equality operator.
The first comparison dict1 == dict2 returns True because both dictionaries have the same keys and corresponding values.
The second comparison dict1 == dict3 returns False because, although the keys are the same, the value for key ‘c’ differs between the two dictionaries (3 vs 4).
In the case of lists and tuples, the order matters of the elements matter. If both the lists or tuples have exactly the same value. If the contents are identical, it returns True; otherwise, it returns False.
In the case of dictionaries, the order does not matter, but the key-value pairs in one dictionary should be equal to the key-value pairs in the other for the operator to return true.
To learn more about different data types in Python, check the following video out:
4 Advanced Use Cases of == Operator in Python
In this section, we’ll cover 4 advanced use cases of the == operator. When you start working on more complex projects, you’ll frequently come across situations where you’d want to use the == operator.
We’ll go over the following:
- Using == with Conditional Statements
- Using == with Loops
- Comparing the Output of Functions
- Comparing Objects of Custom Classes
1. How to Use == With Conditional Statements
You can use the == operator in conditional statements to test for equality. It allows you to execute specific blocks of code based on whether a certain condition is true.
For instance, in an if-else structure, when the condition (that involves ==) in the if statement is true, the code inside the if block is executed.
If it’s not true (i.e., the condition is false), then the code in the else block is executed.
The following example demonstrates this use case:
age = 18
if age == 18:
print("You are just old enough to vote.")
else:
print("You are either too young or old enough to vote.")
In this example, we’re using the == in an if-else statement. If the age is exactly 18, it will print “You are just old enough to vote.” Otherwise, it will print “You are either too young or old enough to vote.”
2. How to Use == Operator with Loops
The operator is often used with loops to check a condition for each item in a sequence, or to stop the loop when a certain condition is met. It is also used with try-except blocks to identify and handle any syntax error.
You can use == operator to filter items, search for specific items, or break out of a loop early.
The following example demonstrates this use case:
counter = 0
target = 5
while True:
counter += 1
print(f"Counter is at: {counter}")
if counter == target:
print("Target reached!")
break
In this code, the while True loop will continue indefinitely because the condition is always True. Inside the loop, we increment counter by 1, then print the current value of counter.
We then check if counter is equal to target (5).
If counter equals target, we print “Target reached!” and break out of the loop with the break statement. This stops the loop from running indefinitely.
The output is given below:
3. How to Compare the Output of Functions With == Operator
A very handy use case of == operator is to compare the output of a function with a variable.
The following example demonstrates this use case:
def square(x):
return x * x
print(square(5) == 25)
This code defines a function called square that takes an argument x and returns the square of x.
Then, it checks if the result of square (5) is equal to 25 using the == operator, which is True because 5 squared equals 25.
The output is given below:
4. How to Compare Objects of Custom Classes
With the == operator, you can also compare objects of custom class method.
The following examples demonstrate this use case:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
person1 = Person("Alice", 25)
person2 = Person("Alice", 25)
print(person1 == person2) # Returns False
The will return False because of the difference in the age of the two instances. The output is given below:
In custom classes, you can also check if instances of class variables point to the same memory address or memory location. This is a more advanced use case and is often used in system programming.
Final Thoughts
As you explore Python programming, mastering the == operator is important. It’s a fundamental part of Python’s syntax, playing a key role in comparing values, controlling program flow, and making decisions in your code.
Understanding and using == opens up a new level of interactivity in your programs. You can write code that responds differently depending on user input, or loops that execute until a certain condition is met. You can also search for specific elements in a list or check if two data structures are identical.
In essence, the == operator adds value by making your code more flexible and powerful. It allows your programs to make decisions, which is a key aspect of intelligent behavior!
Frequently Asked Questions
Now that you’ve learned the == operator in Python, let’s go over some questions that you might have when using the == operator in your applications.
What is the Difference Between Equality and Assignment Operator?
In Python, a single equal sign = is used for assignment, while two consecutive equal signs == are used for comparison. The expression a = 10 will assign variable a a value of 10.
On the other hand, the expression a == 10 tests if the value stored in the variable a is equal to 10, resulting in a Boolean value (True or False).
What is the Purpose of Double == Equality Operator?
The purpose of the double equal sign == in Python is to compare values of two expressions or objects. It checks if the values are equal and returns a Boolean result (True or False).
This operator is often used in conditional statements and loops to check for equality and control the program flow accordingly.
Can I Compare Values With == Operator?
Yes! In Python, you can compare different types of values with the operator, such as integers, floats, strings, lists, tuples, and dictionaries.
When using the operator for comparison, Python checks if the values on both sides of the operator are equal and returns True if they are, or False if they are not.