Managing files and folders is a common task in most programming projects. Python provides many built-in tools for dealing with them. One of the important operations in file management is deleting files.
Python offers different methods for file deletion. The built-in ‘os’ module, the ‘shutil’ library, and the more recent ‘pathlib’ module all provide useful functions for handling file and directory deletion. When handling file deletion operations, paying attention to potential errors, such as file not found or permission issues, is crucial to ensure your code runs smoothly and prevents data loss.
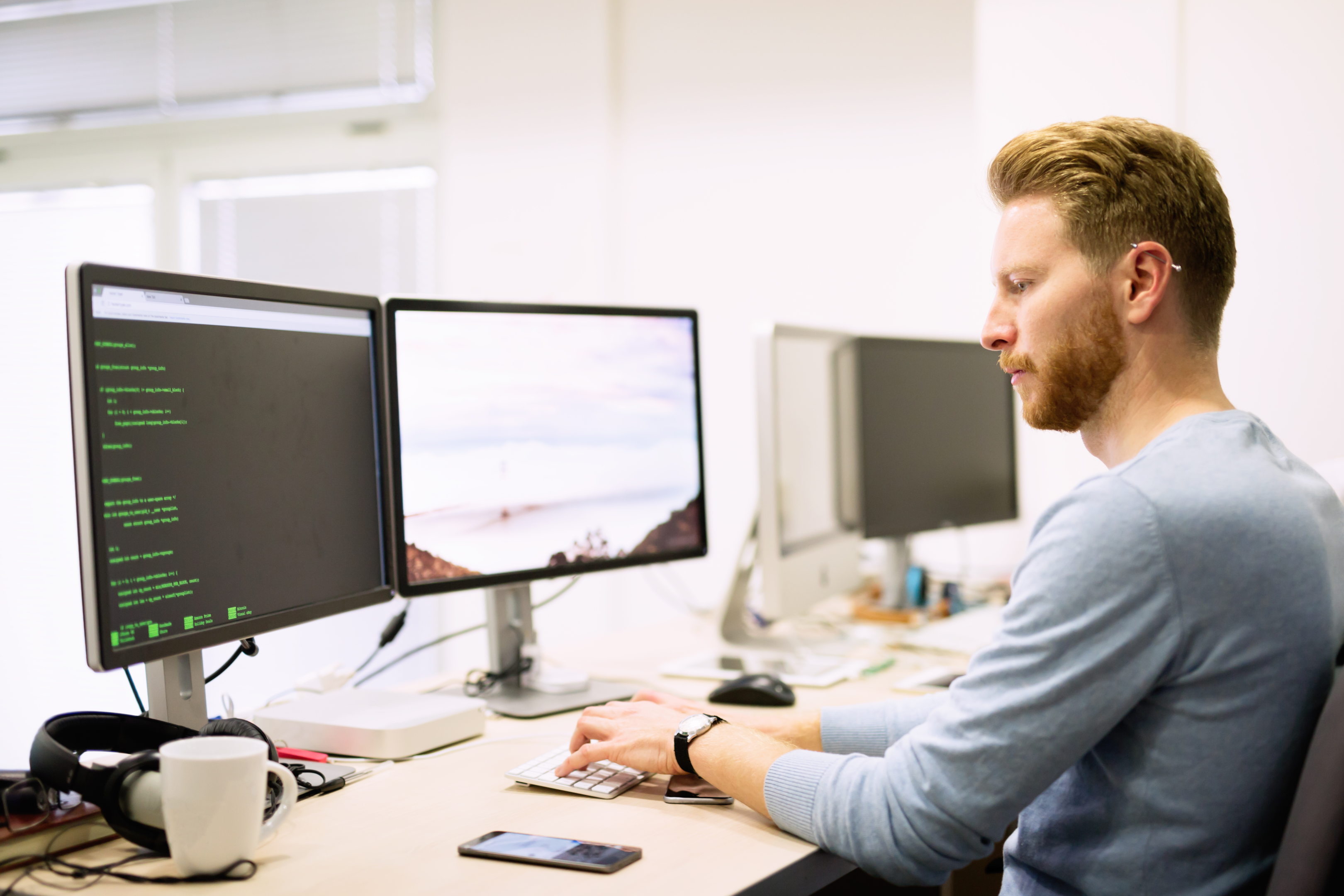
We’ll explore different ways to remove files in Python, along with tips and best practices for managing file operations. These techniques will help you maintain order in your projects and ensure efficient file management.
Let’s get into it!
6 Methods to Delete Files From Python
Working with files is a regular part of programming, and Python offers several built-in methods to handle file operations such as reading, writing, and deleting.
In this section, we’ll go over six methods for deleting files and directories in Python.
Note: Deleting files must be done with care as it is a permanent operation.
1. os.remove(file_path)
The os.remove() function is a part of the os module, which is a set of operating system dependent functionalities.
This function is compatible with both Python 2 and 3 and can be used to delete a single file from either Windows or Unix-based systems.
To use os.remove(), first import the os module by adding import os at the beginning of your script. Then, call the os.remove() function and pass the path to the file you want to delete as an argument.
Let’s say I have a folder named app in local drive E. The folder contains a new file named file1.txt.
On the top left of the screen, you can see a file named file1.txt. This is the file we want to delete.
To delete file, I can use the following code in the main.py Python file:
import os
file_path = "E:\\app\\file1.txt"
os.remove(file_path)
The result will be:
As you can see, the file1.txt is no longer present in the directory and is successfully deleted.
2. os.unlink(file_name)
The os.unlink() function operates in the same way as os.remove(), and both can be used interchangeably to delete files.
The syntax for os.unlink() is straightforward: you pass the path of the file you want to delete as a string argument to the function. Here’s a basic example:
import os
# Specify the file name
file_name = "test.txt"
# Use os.unlink() to delete the file
os.unlink(file_name)
In this example, we first import the os module, which provides a portable way of using operating system-dependent functionality. We then specify the name of the file to be deleted and use os.unlink() to delete it.
3. pathlib.Path.unlink()
You can also delete single files using the pathlib.Path.unlink() method available in the pathlib module, which has been introduced in Python 3.4.
To use pathlib.Path.unlink(), first import the pathlib module by adding import pathlib at the beginning of your script. Then, create a Path object for the file you want to delete and call the unlink() method on it.
Let’s repeat the same example as the one above, this time using pathlib.path.unlink():
from pathlib import Path
file_path = Path("E:\\app\\file1.txt")
file_path.unlink()
The above code will delete file1.txt from the app folder.
4. os.rmdir(directory_name)
The os.rmdir() function in Python is used to delete an empty directory. It’s important to note that this function can only delete empty directories. If the directory is not empty, you’ll encounter an error.
The syntax for os.rmdir() is simple: you pass the path of the directory you want to delete as a string argument to the function. Here’s a basic example:
import os
# Specify the directory name
directory_name = "empty_directory"
# Use os.rmdir() to delete the directory
os.rmdir(directory_name)
In this example, we first import the os module, which provides a portable way of using operating system-dependent functionality. We then specify the name of the directory to be deleted and use os.rmdir() to delete it.
As with any operation that modifies the file system, care should be taken when using os.rmdir(). It’s always a good idea to first check if the directory exists and is indeed empty before attempting to delete it. This can prevent errors and unintended data loss.
5. os.walk()
Another effective method to remove multiple files is by using the os.walk() function.
The os.walk() generates a directory tree, allowing you to filter files based on their names or other attributes like is_dir for directories.
To demonstrate this method, we’ll create files named file1.txt and file2.txt as shown in the top left of the image:
Let’s delete these files using the os.walk() function. You can use the following code to achieve this:
import os
folder_path = 'E:\\app'
for root, dirs, files in os.walk(folder_path):
for file in files:
if file.endswith('.txt'):
os.remove(os.path.join(root, file))
After running the above Python script, the 2 files with .txt extension will be deleted:
6. Glob Module
The glob module makes finding and deleting multiple files matching a specified pattern easy.
For instance, to remove all .jpg files starting with ‘P’, you can use the following code:
import glob, os
for f in glob.glob("P*.jpg"):
os.remove(f)
This code snippet uses the glob.glob() function and returns a list of file paths matching the given pattern. It then iterates through this list, removing each file using the os.remove() function.
How to Handle Errors and Exceptions When Deleting Files
When working with files in Python, it’s important to handle errors and exceptions to ensure the stability of your code.
One common error that might occur is attempting to delete a particular file that does not exist or has an incorrect path.
To handle such situations, you can use the os.path.exists() function to check if the file exists before attempting to remove it.
The following code snippet demonstrates how to do this:
import os
filename = "example.txt"
if os.path.exists(filename):
os.remove(filename)
else:
print("The file does not exist.")
Since we have no example.txt in our directory, we will get the following error output:
However, a more Pythonic way to handle exceptions is by using the try and except blocks. This allows you to handle specific exceptions such as FileNotFoundError or the more general OSError.
The following is an example of how to handle these exceptions:
import os
filename = "example.txt"
try:
os.remove(filename)
except FileNotFoundError:
print("The file does not exist.")
except OSError:
print("An error occurred while attempting to remove the file.")
In this example, we try to remove the file using the os.remove() function. If a FileNotFoundError occurs, we print a message informing the user that the file does not exist or it’s an empty directory.
For other operating system-related errors, the OSError exception is used to handle them.
By handling errors and exceptions, you can ensure that your code runs smoothly and minimize the likelihood of unexpected behavior.
Learn more about error handling in Python files by watching the following video:
Final Thoughts
Learning how to delete files using Python is a critical skill that adds immense value to your coding toolkit. It gives you the control to manage your system’s resources in a flexible manner.
These concepts empower you to automate file cleanup processes and maintain a tidy and optimized workspace.
It’s also an essential step towards mastering Python’s file-handling capabilities, which can be important in numerous applications such as data analysis, machine learning, and web development.
Frequently Asked Questions
How can I remove a single file using Python?
To remove a single file in Python, you can use the os.remove() function from the os module. First, import the module and then call the function with the file path as an argument.
For example:
import os
os.remove('path/to/your/file.txt')
What is the method to delete multiple files in Python?
To delete multiple files in Python, you can use a loop with the os.remove() function. First, import the os module, and then iterate over a list of file paths, removing each one in the loop.
For example:
import os
file_paths = ['file1.txt', 'file2.txt', 'file3.txt']
for file_path in file_paths:
os.remove(file_path)
How do I delete a directory and all its contents in Python?
To delete a directory and all its contents in Python, you can use the shutil.rmtree() function from the shutil module. First, import the module and then call the function with the directory path as an argument.
For example:
import shutil
shutil.rmtree('path/to/your/directory')
Can I remove a file only if it exists in Python?
Yes, you can remove a file in Python only if it exists. To do this, you can use a try-except block with the os.remove() function. If the file does not exist, the FileNotFoundError exception is caught and you can handle it accordingly.
For example:
import os
try:
os.remove('path/to/your/file.txt')
except FileNotFoundError:
print("File not found")
How can I use pathlib to delete a file?
Using pathlib, you can delete a file with the unlink() method. First, import the Path class from the pathlib module and create a Path object for your file. Then, call the unlink() method to remove the file.
For example:
from pathlib import Path
file_path = Path('path/to/your/file.txt')
file_path.unlink()