Python is a versatile and widely used programming language, known for its simplicity and easy-to-understand syntax. When you’re setting up a Python development environment, one of the first tasks is locating where Python is installed on your machine. This information is crucial for configuring different tools and managing libraries and environments.
Python is normally installed in the system’s default program files directory. On Windows, it’s typically found in the “C:\PythonXX” folder. On Linux or Mac, it’s often in “/usr/local/bin/pythonX.X”. You can check your Python installation path by running “python –version” or “which python” in the command line.
This article will help you understand how to find the Python installation location on your system, focusing on different methods and operating systems. This knowledge will assist you in setting up your programming environment correctly and provide a better understanding of how Python interacts with your machine.
Let’s get into it!
Python Installation Locations
On various operating systems like Windows, macOS, or Linux, Python’s installation location can vary due to user preferences or installation packages from different sources.
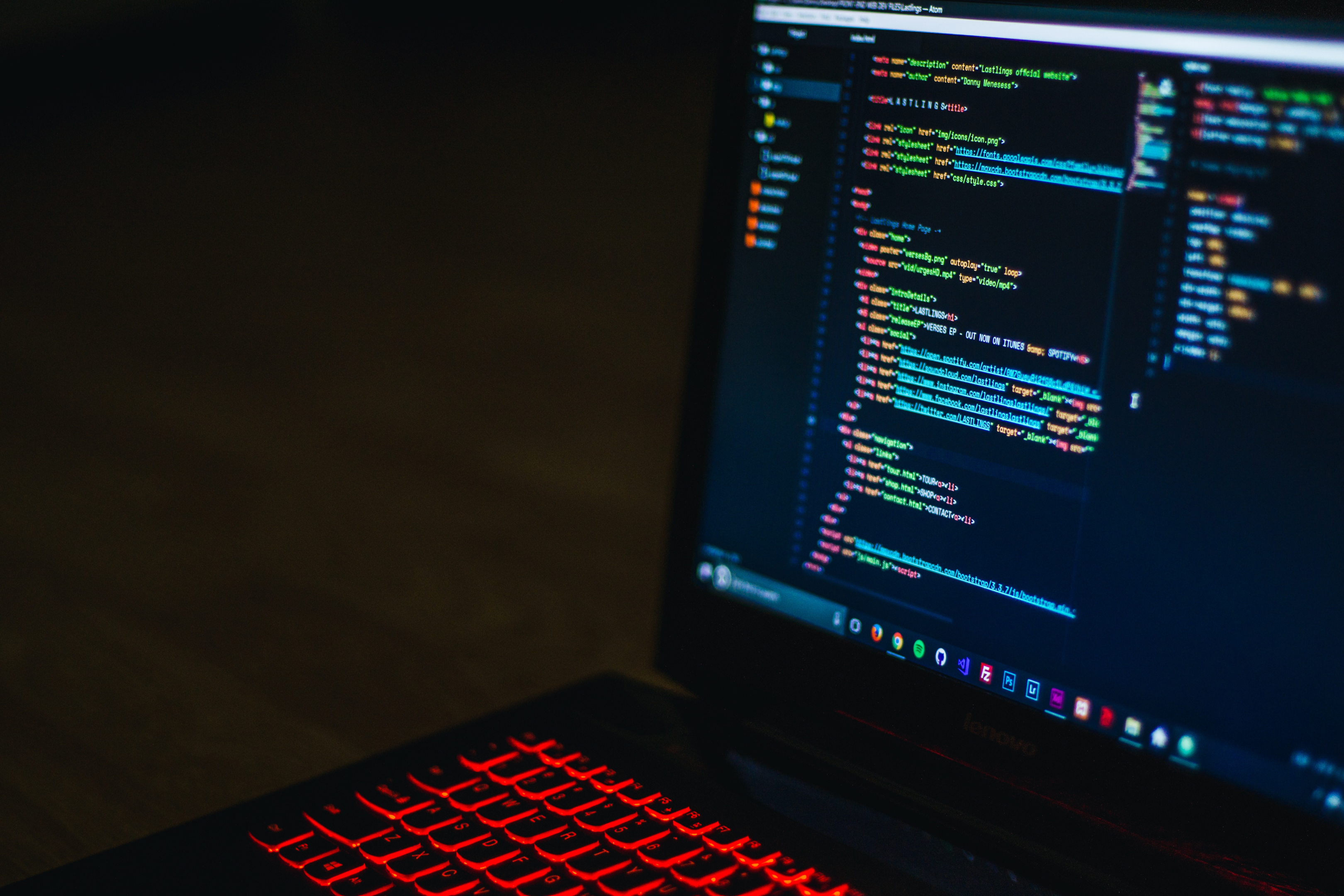
In this section, we’ll look at the installation location of Python for different operating systems. Specifically, we’ll look at the following:
- Python Installation location on Windows
- Python Installation location on MacOS
- Python Installation location on Linux
1. Python Installation Location on Windows
By default, Python installations on Windows are located in the C:\ directory or C:\Users\<User>\AppData\Local\Programs.
The common installation paths for both 32-bit and 64-bit versions may reside in the C:\PythonXX folder, where XX stands for the Python version (e.g., C:\Python27 for Python 2.7).
In newer installations, the 64-bit version is often located in the C:\Program Files\PythonXX folder, while the 32-bit version is in the C:\Program Files (x86)\PythonXX folder.
To find the exact location of your Python installation on Windows, you can use the command prompt by typing where python and press Enter. This command will display the file paths of any installed Python versions on your system.
The following image demonstrates this prompt:
2. Python Installation Location on MacOS
On macOS, Python is typically installed in the /Library/Frameworks/Python.framework/Versions directory, with different versions contained in their respective subfolders (e.g., /Library/Frameworks/Python.framework/Versions/3.9 for Python 3.9).
Alternatively, Python installations managed by Homebrew are located in /usr/local/Cellar/python.
To check the exact path of your Python installation, you can open the terminal and type which python or which python3. Pressing “Enter” will display the file path of the active Python version on your system.
3. Python Installation Location on Linux
In most Linux distributions, Python is installed by default and can be found in the /usr/bin/ directory. The installed versions usually include both Python 2 and Python 3.
For Python 2.x, the executable is named python, while for Python 3.x, it’s named python3.
For systems with multiple Python installations or custom installation paths, you can find the location by typing which python or which python3 in the terminal and pressing Enter.
The command will show the file path of the active Python version on your system.
In the next section, we’ll go over how you can use built-in Python modules to locate the Python folder in your system.
How to Use Python’s os and sys Modules to Locate Python Folder
Python’s os and sys modules are built-in modules that allow you to interact with the operating system.
To locate where Python is installed, follow the steps given below:
- Run Python interpreter by typing python in the terminal or command prompt.
- Execute the following commands:
import os
import sys
print(os.path.dirname(sys.executable))
This Python script is used to print the directory of the Python interpreter being used to execute the script. os.path.dirname(sys.executable) gets the path of the currently running Python interpreter.
The output is given below:
How to Check the Location of Python Executables
When you are working in Python, you’ll want to install libraries from time to time. In most cases, you might want to check the path of a certain library.
You can check the path of a Python library or executable with the pip package manager. To do this, open up CMD and run the following code:
pip show <package name>
Let’s say I want to check the location of NumPy library on my operating system. To do this, I’ll type the following prompt in CMD:
pip show numpy
The output is given below:
How to Configure Python Path
When you first install Python on Windows, it’s important that you configure the path environment variable.
This means you should let your operating system know the path of your installation folder, which will allow the interpreter to refer to this path when running Python files.
We’ve listed a step-by-step guide to setting your environment variable and variables below:
Windows
- Right-click on ‘My Computer’ or ‘This PC,’ and select ‘Properties.’
- Click on ‘Advanced system settings.’
- Go to the ‘Advanced’ tab and click on ‘Environment Variables.’
- In the ‘System variables’ section, locate the ‘Path’ variable, select it, and click on ‘Edit.’
- Add the path to the Python executable (e.g., C:\Python<version>\Scripts;C:\Python<version>), and click ‘OK.’
macOS and Linux
- Open the terminal (Terminal on macOS, and Ctrl + Alt + T on Linux).
- Edit the shell configuration file (~/.bashrc for bash shell, ~/.zshrc for zsh shell).
- Add the following line: export PATH=”/path/to/python-<version>/bin:$PATH”
- Save the file and restart the terminal for the changes to take effect.
How to Verify Python Version and Installation
Before starting any Python project, it’s essential to know the version of Python installed on the system as well as its location.
It’ll help you ensure compatibility between packages, libraries, and other tools.
This section will guide you through verifying your Python version and installation using various Python-related commands and performing cross-platform checks.
1. Python-related Commands
To find the version of Python installed on any platform, you can use the python –version command in the terminal or command prompt.
It returns the Python 2 version installed on your system. To check for the Python 3 version, you can use the python3 –version command instead. These commands help you determine which Python interpreter you are currently using.
In case you are using both Python 2 and Python 3 versions, you can verify the compatibility of a specific module by importing it into the Python interpreter.
For example, to check if the re module is compatible, you can try running the following command:
import re
print(re.__version__)
When you run the above command, you’ll get an output similar to the following:
An output like the above suggests that Python’s re module is compatible with your current Python version.
If you’d like to learn how to switch to the latest version of Python, check out our quick and easy guide on how to update Python.
2. Cross-platform Verification
To find where Python is installed on your system, you can use the following Python code snippet for a cross-platform solution:
import sys
print(sys.executable)
This code will print the location of the Python interpreter executable. Depending on your operating system, this might differ.
The output is given below:
By knowing this location, you can ensure that you are using the correct Python interpreter for your projects.
Troubleshooting Common Python Issues
In this section, we’ll talk about some common issues that you may face when working with Python paths.
Let’s get into it!
1. Path-related Problems
For beginners, one common problem when using Python is related to file paths.
When Python is installed, its location may not be added automatically to the system environment variables PATH.
This could result in the “Python not found” error when trying to run Python scripts or access the Python shell.
To troubleshoot this issue, you can:
- Ensure Python is installed correctly by checking the installation folder, typically found in:
- Windows: C:\Python{version}
- macOS and Linux: /usr/local/bin/python{version}
- Add Python to the PATH variable to make it accessible system-wide:
- Windows: Open the Control Panel, navigate to System and Security > System > Advanced system settings > System Environment Variables, and edit the PATH variable to include the Python installation folder.
- macOS and Linux: Open the terminal and add the Python installation directory to the PATH variable using the export command, such as export PATH=/usr/local/bin/python{version}:$PATH.
- Save and restart the terminal or Command Prompt to apply the changes.
After following the steps above correctly, you’ll no longer run into any errors with running Python files.
2. Version Compatibility
Different Python versions might lead to version compatibility issues, especially when working with third-party modules and libraries.
To overcome this, you can:
- Check your Python version by running python –version in the terminal or Command Prompt.
- Verify the compatibility of a package by checking its documentation or the PyPI page. Packages usually list compatible Python versions.
- Use a virtual environment to manage and isolate dependencies for different projects.
By addressing path-related problems and ensuring version compatibility, you can resolve some of the most common Python issues.
To learn more about error resolution in Python, check the following video out:
Final Thoughts
Understanding where Python is installed on your system is a fundamental aspect of becoming an efficient programmer.
It allows you to manage your programming environment effectively and is key when dealing with using multiple versions of Python versions or when installing modules.
It also helps you in cases when you want to use specific versions for different projects, or when you need to install packages globally or just for one project.
Furthermore, knowing the path of your Python installation can also help you troubleshoot issues related to your environment setup or version conflicts and fine-tune your coding environment to your liking.
It’s an essential step in setting up an integrated development environment, and it makes using code editors or Python-related software smoother and more efficient!
Frequently Asked Questions
In this section, we’ve listed some frequently asked questions that most beginners have related to the Python installation directory.
Where is Python install location on Windows?
Python is installed in the default location C:\PythonXY where XY represents the version number.
For example, C:\Python39 for Python 3.9. It might also get installed under C:\Users\YourUsername\AppData\Local\Programs\Python\PythonXY for per-user installations.
How to find Python location in CMD?
To find the Python installation path in CMD, you can use the following command:
python -c
import os, sys
print(os.path.dirname(sys.executable))
This command imports the os and sys modules and prints the directory of the Python executable.
Where is Python’s location in Windows 10?
On Windows 10, Python’s default location is C:\PythonXY or C:\Users\YourUsername\AppData\Local\Programs\Python\PythonXY for per-user installations.
How to check if Python is installed?
To check if Python is installed, open a command prompt or terminal and type in:
python --version
If Python is installed, the command will display the version of Python.
If it’s not installed, you’ll get an error or the Microsoft Store may open for Python installations in certain cases on Windows.
How to find Python package’s installation location?
Python packages are generally installed in a subfolder of the Python installation. The specific location depends on whether you’re using a virtual environment or not.
To find the installation location of a package, open a terminal or command prompt and type:
pip show package-name
Replace “package-name” with the name of the package you want to find the location for. The output will show the package’s location along with other information.